Content Management System für ein Blog-System
Unser System hat eine festgelegte Ordnerstruktur, die die Übersichtlichkeit des Projektes während der Implementierung garantieren soll.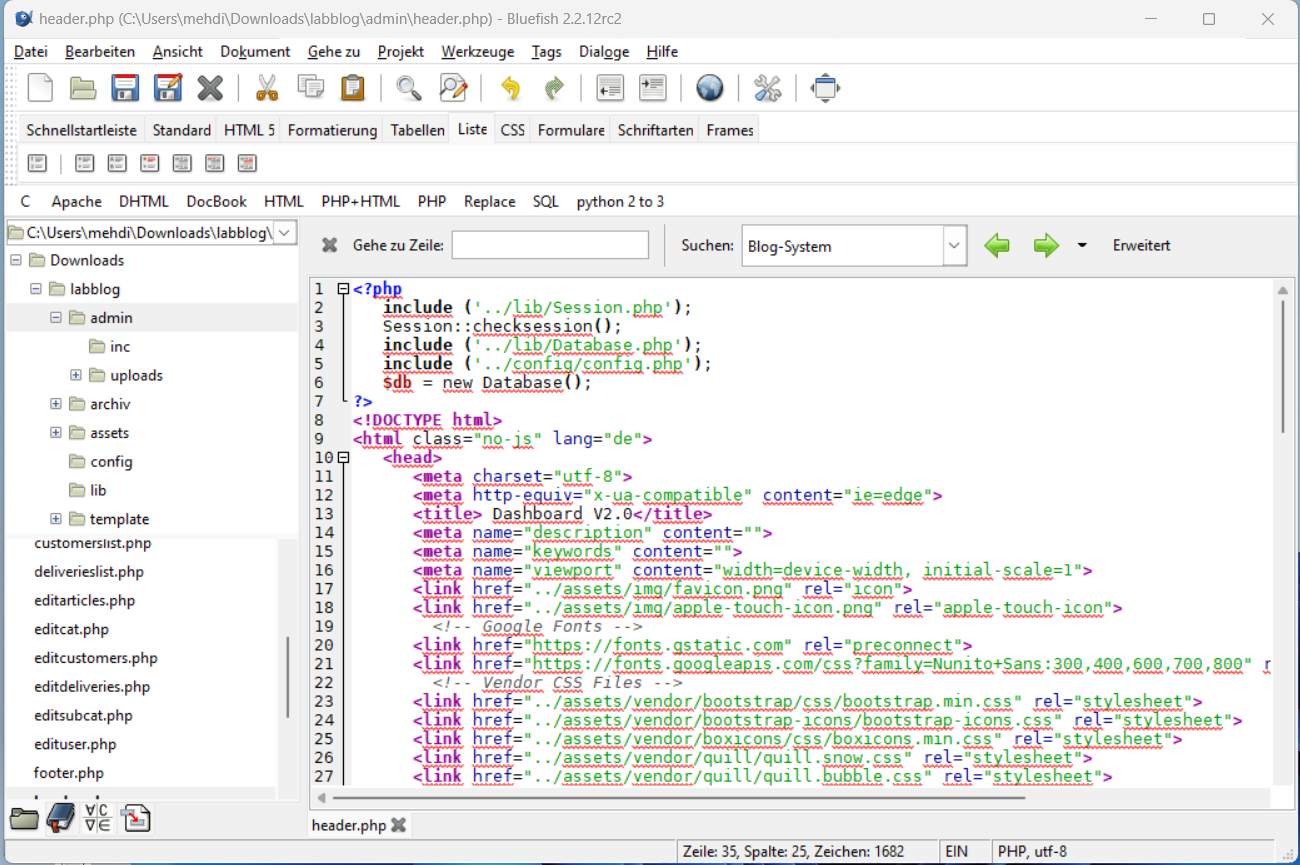
Verzeichnisstruktur des Basissystems Blogsystem
Im Ordner assets sind alle einbindbaren Cascading-Stylesheet-Dateien, Bilder, JavaScript-Dateien, Fonts-Dateien und fertiges Frontend-Framework wie Bootstrap enthalten, die für die u.a. Stammdatenverwaltung eingesetzt werden können.
Im Hauptordner werden die Hauptdateien, Infodateien und Verwaltungsdateien abgelegt.
Hauptdateien
Die Hauptdateien auf unterster Ebene des Blogsystems werden wie folgt verwendet:connect.php
Diese Datei (config/connect.php) baut die Verbindung mit dem MYSQL-Server auf. Dabei müssen der Hostname, Datenbankname, Benutzername und das Passwort bekannt und richtig angegeben werden. Die Datei soll in einem Unterverzeichnis mit dem Namen "config" gespeichert werden. Der Inhalt könnte wie folgt aussehen:<?php define("DB_HOST", "localhost"); define("DB_USER", "dbshop"); define("DB_PASSWORD", "dbshop_password"); define("DB_NAME", "dbshop"); function db_connect() { $result = new mysqli("localhost", "dbshop", "dbshop_password", "dbshop"); if (!$result) return FALSE; $result->autocommit(TRUE); return $result; } function get_cgi_param ($feld, $default) { $var = $default; $rmeth = $_SERVER["REQUEST_METHOD"]; if ($rmeth == "GET") { if (isset ($_GET[$feld]) && $_GET[$feld] != "") { $var = $_GET[$feld]; } } elseif ($rmeth == "POST") { if (isset ($_POST[$feld]) && $_POST[$feld] != "") { $var = $_POST[$feld]; } } return $var; } ?>
Database.php
Diese Datei (lib/Database.php) beinhaltet alle wichtigen Funktionen, die für die Abfragen der Tabellen verwendet werden. Die Datei soll in einem Unterverzeichnis mit dem Namen "lib" gespeichert werden.<?php Class Database{ public $host = DB_HOST; public $user = DB_USER; public $password = DB_PASSWORD; public $dbname = DB_NAME; public $link; public $error; public function __construct(){ $this->connectDB(); } private function connectDB(){ $this->link = new mysqli($this->host, $this->user, $this->password, $this->dbname); if(!$this->link){ $this->error ="Connection fail".$this->link->connect_error; return false; } } public function select($query){ $result = $this->link->query($query) or die($this->link->error.__LINE__); if($result->num_rows > 0){ return $result; } else { return false; } } public function insert($query){ $insert_row = $this->link->query($query) or die($this->link->error.__LINE__); if($insert_row){ return $resultid= $this->link->insert_id; exit(); } else { die("Error :(".$this->link->errno.")".$this->link->error); } } public function insert1($query){ $insert_row = $this->link->query($query) or die($this->link->error.__LINE__); if($insert_row){ return 1; exit(); } else { die("Error :(".$this->link->errno.")".$this->link->error); } } public function update($query){ $update_row = $this->link->query($query) or die($this->link->error.__LINE__); if($update_row){ return 1; exit(); } else { die("Error :(".$this->link->errno.")".$this->link->error); } } public function update1($query){ $update_row = $this->link->query($query) or die($this->link->error.__LINE__); if($update_row){ return $resultid1= 1; exit(); } else { die("Error :(".$this->link->errno.")".$this->link->error); } } public function delete($query){ $delete_row = $this->link->query($query) or die($this->link->error.__LINE__); if($delete_row){ return "<span style='color:#fff;'>Entry successfully deleted. </span>"; exit(); } else { die("Error :(".$this->link->errno.")".$this->link->error); } } public function delete1($query){ $delete_row = $this->link->query($query) or die($this->link->error.__LINE__); if($delete_row){ return 1; exit(); } else { die("Error :(".$this->link->errno.")".$this->link->error); } } } ?>
Session.php
Diese Datei (lib/Session.php) enthält die Funktionen, um die Sitzung der Variablen zu ermitteln. Die Datei soll in einem Unterverzeichnis mit dem Namen "lib" gespeichert werden.<?php class Session{ public static function init(){ session_start(); } public static function set($key,$value){ $_SESSION[$key]=$value; } public static function get($key){ if (!empty($_SESSION[$key])){ return $_SESSION[$key]; }else{ return false; } } public static function checksession(){ self::init(); if (self::get("login")==false || self::get("role")=="subscriber"){ self::destroy(); header("Location:login.php"); } } public static function destroy(){ session_destroy(); } } ?>
header.php
Diese Datei (admin/header.php) beinhaltet das HTML-Grundgerüst, das Einbinden von CSS-, JS- und PHP-Dateien.<?php include ("../lib/Session.php"); Session::checksession(); include ("../lib/Database.php"); include ("../config/connect.php"); $db = new Database(); ?> <!DOCTYPE html> <html class="no-js" lang="en"> <head> <meta charset="utf-8"> <meta http-equiv="x-ua-compatible" content="ie=edge"> <title> Dashboard V2.0</title> <meta name="description" content=""> <meta name="keywords" content=""> <meta name="viewport" content="width=device-width, initial-scale=1"> <link href="../assets/img/favicon.png" rel="icon"> <link href="../assets/img/apple-touch-icon.png" rel="apple-touch-icon"> <!-- Google Fonts --> <link href="https://fonts.gstatic.com" rel="preconnect"> <link href="https://fonts.googleapis.com/css?family=Nunito+Sans:300,400,600,700,800" rel="stylesheet"> <!-- Vendor CSS Files --> <link href="../assets/vendor/bootstrap/css/bootstrap.min.css" rel="stylesheet"> <link href="../assets/vendor/bootstrap-icons/bootstrap-icons.css" rel="stylesheet"> <link href="../assets/vendor/boxicons/css/boxicons.min.css" rel="stylesheet"> <link href="../assets/vendor/quill/quill.snow.css" rel="stylesheet"> <link href="../assets/vendor/quill/quill.bubble.css" rel="stylesheet"> <link href="../assets/vendor/remixicon/remixicon.css" rel="stylesheet"> <link rel="stylesheet" href="https://cdn.jsdelivr.net/npm/summernote@0.8.20/dist/summernote-lite.min.css"> <!--link href="../assets/vendor/datatables/style.css" rel="stylesheet"--> <link href="https://cdn.jsdelivr.net/npm/simple-datatables@latest/dist/style.css" rel="stylesheet" type="text/css"> <!-- Template Main CSS File --> <link href="../assets/css/style.css" rel="stylesheet"> </head> <body> <!-- ======= Header ======= --> <header id="header" class="header fixed-top d-flex align-items-center"> <div class="d-flex align-items-center justify-content-between"> <a href="../index.php" class="logo d-flex align-items-center"> <img src="../assets/img/haw-logo.jpg" alt=""> <span class="d-none d-lg-block">Dashboard</span> </a> <i class="bi bi-list toggle-sidebar-btn"></i> </div><!-- End Logo --> <div class="search-bar"> <form class="search-form d-flex align-items-center" method="POST" action="#"> <input type="text" name="query" placeholder="search" title="Enter the search keyword"> <button type="submit" title="Search"><i class="bi bi-search"></i></button> </form> </div><!-- End Search Bar --> <nav class="header-nav ms-auto"> <ul class="d-flex align-items-center"> <li class="nav-item dropdown pe-3"> <?php $uploadDirectory = __DIR__."/"; $pic=Session::get("pic"); $path=$uploadDirectory.$pic; ?> <a class="nav-link nav-profile d-flex align-items-center pe-0" href="#" data-bs-toggle="dropdown"> <img src="<?php echo $pic; ?>" alt="Profile" class="rounded-circle"> <span class="d-none d-md-block dropdown-toggle ps-2"><?php echo Session::get("name");?></span> </a><!-- End Profile Iamge Icon --> <ul class="dropdown-menu dropdown-menu-end dropdown-menu-arrow profile"> <li class="dropdown-header"> <h6><?php echo Session::get("name");?></h6> </li> <li> <hr class="dropdown-divider"> </li> <li> <a class="dropdown-item d-flex align-items-center" href="profile.php"> <i class="bi bi-person"></i> <span>Profile</span> </a> </li> <li> <hr class="dropdown-divider"> </li> <?php if(isset($_GET["action"]) && $_GET["action"]=="logout"){ Session::destroy(); echo '<script>window.location="login.php";</script>'; } ?> <li> <a class="dropdown-item d-flex align-items-center" href="?action=logout"> <i class="bi bi-box-arrow-right"></i> <span>Exit</span> </a> </li> </ul><!-- End of Profile Dropdown Items --> </li><!-- End of Profile Nav --> </ul> </nav><!-- End of Icons Navigation --> </header>
nav.php
Diese Datei (admin/nav.php) beinhaltet den Code für die Navigation von Stammdaten.<aside id="sidebar" class="sidebar"> <ul class="sidebar-nav" id="sidebar-nav"> <li class="nav-item"> <a class="nav-link collapsed" href="index.php"> <i class="bi bi-grid"></i> <span>Dashboard</span> </a> </li><!-- End Dashboard Nav --> <?php if(Session::get("role")=="admin"){ ?> <li class="nav-item"> <li class="nav-item"> <a class="nav-link collapsed" data-bs-target="#forms-user" data-bs-toggle="collapse" href="#"> <i class="bi bi-journal-text"></i><span>Users Management</span><i class="bi bi-chevron-down ms-auto"></i> </a> <ul id="forms-user" class="nav-content collapse " data-bs-parent="#sidebar-nav"> <li> <a href="adduser.php"> <i class="bi bi-circle"></i><span>Insert User</span> </a> </li> <li> <a href="userlist.php"> <i class="bi bi-circle"></i><span>Views Users</span> </a> </li> </ul> </li> <li class="nav-item"> <a class="nav-link collapsed" data-bs-target="#forms-cat" data-bs-toggle="collapse" href="#"> <i class="bi bi-journal-text"></i><span>Categories Management</span><i class="bi bi-chevron-down ms-auto"></i> </a> <ul id="forms-cat" class="nav-content collapse " data-bs-parent="#sidebar-nav"> <li> <a href="addcat.php"> <i class="bi bi-circle"></i><span>Insert Category </span> </a> </li> <li> <a href="catlist.php"> <i class="bi bi-circle"></i><span>Views Categories</span> </a> </li> </ul> </li> <li class="nav-item"> <a class="nav-link collapsed" data-bs-target="#forms-subcat" data-bs-toggle="collapse" href="#"> <i class="bi bi-journal-text"></i><span>Subcategories Management</span><i class="bi bi-chevron-down ms-auto"></i> </a> <ul id="forms-subcat" class="nav-content collapse " data-bs-parent="#sidebar-nav"> <li> <a href="addsubcat.php"> <i class="bi bi-circle"></i><span>Insert Subcategory </span> </a> </li> <li> <a href="subcatlist.php"> <i class="bi bi-circle"></i><span>Views Subcategories</span> </a> </li> </ul> </li> <?php } ?> <li class="nav-item"> <a class="nav-link collapsed" data-bs-target="#forms-article" data-bs-toggle="collapse" href="#"> <i class="bi bi-journal-text"></i><span>Articles Management</span><i class="bi bi-chevron-down ms-auto"></i> </a> <ul id="forms-article" class="nav-content collapse " data-bs-parent="#sidebar-nav"> <li> <a href="addarticle.php"> <i class="bi bi-circle"></i><span>Insert Article </span> </a> </li> <li> <a href="articleslist.php"> <i class="bi bi-circle"></i><span>Views Articles</span> </a> </li> <!-- <li> <a href="addgallery.php"> <i class="bi bi-circle"></i><span>Insert Image </span> </a> </li> <li> <a href="gallerieslist.php"> <i class="bi bi-circle"></i><span>Views Images</span> </a> </li> --> </ul> </li> <li class="nav-item"> <a class="nav-link collapsed" data-bs-target="#forms-delivery" data-bs-toggle="collapse" href="#"> <i class="bi bi-journal-text"></i><span>Deliveries Management</span><i class="bi bi-chevron-down ms-auto"></i> </a> <ul id="forms-delivery" class="nav-content collapse " data-bs-parent="#sidebar-nav"> <li> <a href="adddelivery.php"> <i class="bi bi-circle"></i><span>Insert Delivery </span> </a> </li> <li> <a href="deliverieslist.php"> <i class="bi bi-circle"></i><span>Views Deliveries</span> </a> </li> </ul> </li> <li class="nav-item"> <a class="nav-link collapsed" data-bs-target="#forms-customer" data-bs-toggle="collapse" href="#"> <i class="bi bi-journal-text"></i><span>Customers Management</span><i class="bi bi-chevron-down ms-auto"></i> </a> <ul id="forms-customer" class="nav-content collapse " data-bs-parent="#sidebar-nav"> <li> <a href="addcustomer.php"> <i class="bi bi-circle"></i><span>Insert Customer </span> </a> </li> <li> <a href="customerslist.php"> <i class="bi bi-circle"></i><span>Views Customers</span> </a> </li> </ul> </li> </ul> </aside>
footer.php
Diese Datei (admin/footer.php) beinhaltet den Fußbereich und das Einbinden von JS-Dateien.<footer id="footer" class="footer"> <div class="copyright"> <span class="text-center text-sm-left d-md-inline-block">Copyright © Mehdi Bandegani 2022. All Rights Reserved.</span> </div> </footer> <a href="#" class="back-to-top d-flex align-items-center justify-content-center"><i class="bi bi-arrow-up-short"></i></a> <script src="../assets/vendor/javascripts/jquery-3.3.1.min.js"></script> <script src="../assets/vendor/bootstrap/js/bootstrap.min.js"></script> <script src="../assets/vendor/apexcharts/apexcharts.min.js"></script> <script src="../assets/vendor/bootstrap/js/bootstrap.bundle.min.js"></script> <script src="../assets/vendor/chart.js/chart.umd.js"></script> <script src="../assets/vendor/echarts/echarts.min.js"></script> <script src="../assets/vendor/quill/quill.min.js"></script> <script src="../assets/vendor/summernote/summernote-lite.min.js"></script> <script src="../assets/vendor/summernote/lang/summernote-en-US.js"></script> <script src="../assets/vendor/datatables/datatables.js" type="text/javascript"></script> <!--script src="https://cdn.jsdelivr.net/npm/simple-datatables@latest" type="text/javascript"></script--> <script src="../assets/vendor/php-email-form/validate.js"></script> <!-- Template Main JS File --> <script src="../assets/js/main.js"></script> <script> $('#summernote').summernote({ placeholder: 'Write a note', tabsize: 2, height: 400, lang: 'en-US', // default: 'en-US' }); </script> </body> </html>
index.php
Diese Datei (admin/index.php) wird automatisch gestartet und ist die Hauptdatei.<?php include("header.php"); include("nav.php"); ?> <main id="main" class="main"> <div class="pagetitle"> <h1>Dashboard</h1> <nav> <ol class="breadcrumb"> <li class="breadcrumb-item"><a href="../index.php">Home</a></li> <li class="breadcrumb-item active">Dashboard</li> </ol> </nav> </div><!-- End Page Title --> <section class="section"> <div class="row"> <div class="col-lg-2 col-md-2"></div> <div class="col-lg-8"> <div class="card"> <div class="card-body"> <h5 class="card-title">Welcome to the admin dashboard</h5> We use MySQL for the shop system. Since the tables lab_tblcategories, lab_tblsubcategories and lab_tblarticles are to be managed in this shop system, the system should also be a user-dependent shop system. This is achieved using a table lab_tblusers. </div> </div><!-- End Default Card --> </div> <div class="col-lg-2 col-md-2"></div> </div> </section> </main> <?php include("footer.php"); ?>
login.php
Diese Datei (admin/login.php) wird gestartet, damit ein User sich anmeldet, um die Daten zu verwalten. Die Userdaten befinden sich in der Tabelle "lab_tblusers".(Siehe das Tabellenschema)<?php include ("../lib/Session.php"); Session::init(); include ("../lib/Database.php"); include ("../config/connect.php"); $db = new Database(); ?> <?php if(isset($_POST["login"])){ $email=mysqli_real_escape_string($db->link,$_POST["email"]); $password=mysqli_real_escape_string($db->link,$_POST["password"]); $password=md5($password); $query="SELECT * FROM lab_tblusers where email='$email' and password='$password'"; $result=$db->select($query); if($result!=false){ $val= mysqli_fetch_array($result); $row = mysqli_num_rows($result); if($row>0){ Session::set("login",true); Session::set("email",$val["email"]); Session::set("id",$val["id"]); Session::set("role",$val["role"]); Session::set("pic",$val["pic"]); if(Session::get("role")=="admin" || Session::get("role")=="editor"){ header("Location:index.php"); }else{ header("Location:../index.php"); } }else{ $error1="not found!"; echo "<div class='alert alert-danger' role='alert'> <span class='glyphicon glyphicon-exclamation-sign' aria-hidden='true'></span> <span class='sr-only'></span>".$error."</div>"; } }else{ $error2="The username or password does not match!"; } } ?> <html lang="en"> <head> <meta charset="utf-8"> <meta content="width=device-width, initial-scale=1.0" name="viewport"> <title>Pages / Login - Shopsystem</title> <meta content="" name="description"> <meta content="" name="keywords"> <!-- Favicons --> <link href="../assets/img/favicon.png" rel="icon"> <link href="../assets/img/apple-touch-icon.png" rel="apple-touch-icon"> <!-- Google Fonts --> <link href="https://fonts.gstatic.com" rel="preconnect"> <link href="https://fonts.googleapis.com/css?family=Open+Sans:300,300i,400,400i,600,600i,700,700i|Nunito:300,300i,400,400i,600,600i,700,700i|Poppins:300,300i,400,400i,500,500i,600,600i,700,700i" rel="stylesheet"> <!-- Vendor CSS Files --> <link href="../assets/vendor/bootstrap/css/bootstrap.min.css" rel="stylesheet"> <link href="../assets/vendor/bootstrap-icons/bootstrap-icons.css" rel="stylesheet"> <link href="../assets/vendor/boxicons/css/boxicons.min.css" rel="stylesheet"> <link href="../assets/vendor/quill/quill.snow.css" rel="stylesheet"> <link href="../assets/vendor/quill/quill.bubble.css" rel="stylesheet"> <link href="../assets/vendor/remixicon/remixicon.css" rel="stylesheet"> <link href="../assets/vendor/simple-datatables/style.css" rel="stylesheet"> <link href="../assets/css/style.css" rel="stylesheet"> </head> <body> <main> <div class="container"> <section class="section register min-vh-100 d-flex flex-column align-items-center justify-content-center py-4"> <div class="container"> <div class="row justify-content-center"> <div class="col-lg-4 col-md-6 d-flex flex-column align-items-center justify-content-center"> <div class="card mb-3"> <div class="card-body"> <div class="pt-4 pb-2"> <h5 class="card-title text-center pb-0 fs-4">Login to Your Account</h5> <p class="text-center small">Enter your email & password to login</p> </div> <form method="post" enctype="multipart/form-data" action="login.php" class="row g-3 needs-validation" novalidate> <div class="col-12"> <label for="yourEmail" class="form-label">Email</label> <div class="input-group has-validation"> <span class="input-group-text" id="inputGroupPrepend">@</span> <input type="text" name="email" class="form-control" id="yourEmail" required> <div class="invalid-feedback">Please enter your username.</div> </div> </div> <div class="col-12"> <label for="yourPassword" class="form-label">Password</label> <input type="password" name="password" class="form-control" id="yourPassword" required> <div class="invalid-feedback">Please enter your password!</div> </div> <div class="col-12"> <div class="form-check"> <input class="form-check-input" type="checkbox" name="remember" value="true" id="rememberMe"> <label class="form-check-label" for="rememberMe">Remember me</label> </div> </div> <div class="col-12"> <button type="submit" name="login" class="btn btn-primary w-100" value="Login" >Login</button> </div> <div class="col-12"> <p class="small mb-0">Don't have account? <a href="signup.php">Create an account</a></p> </div> </form> </div> </div> </div> </div> </div> </section> </div> </main><!-- End #main --> <a href="#" class="back-to-top d-flex align-items-center justify-content-center"><i class="bi bi-arrow-up-short"></i></a> <!-- Vendor JS Files --> <script src="../assets/vendor/apexcharts/apexcharts.min.js"></script> <script src="../assets/vendor/bootstrap/js/bootstrap.bundle.min.js"></script> <script src="../assets/vendor/chart.js/chart.umd.js"></script> <script src="../assets/vendor/echarts/echarts.min.js"></script> <script src="../assets/vendor/quill/quill.min.js"></script> <script src="../assets/vendor/simple-datatables/simple-datatables.js"></script> <script src="../assets/vendor/tinymce/tinymce.min.js"></script> <script src="../assets/vendor/php-email-form/validate.js"></script> <script src="../assets/js/main.js"></script> </body> </html>
signup.php
Diese Datei (admin/signup.php) wird gestartet, damit ein User sich neu eintragen will. Die Userdaten befinden sich in der Tabelle "lab_tblusers".(Siehe das Tabellenschema)<?php include "../lib/Session.php"; Session::init(); include "../lib/Database.php"; include "../config/connect.php"; $db = new Database(); $uploadDirectory = __DIR__."/"; ?> <?php if(isset($_FILES["uploadFile"])){ $errors= array(); $file_name = $_FILES["uploadFile"]["name"]; $file_size = $_FILES["uploadFile"]["size"]; $file_tmp = $_FILES["uploadFile"]["tmp_name"]; $file_type = $_FILES["uploadFile"]["type"]; $file_ext=strtolower(end(explode('.',$_FILES["uploadFile"]["name"]))); $unique_pic = substr(md5(time()), 0, 10).".".$file_ext; $uploaded_pic = "uploads/user/".$unique_pic; if(empty($errors)==true) { $file_name = mysqli_real_escape_string($db->link, $file_name); $fileExists = true; while ($fileExists) { if (!file_exists($uploadDirectory.$uploaded_pic)) { $fileExists = false; } } move_uploaded_file($file_tmp,$uploadDirectory.$uploaded_pic); }else { $uploaded_pic = "uploads/user/user_pda.png"; } //$userid=Session::get("id"); $name = mysqli_real_escape_string($db->link, $_POST["name"]); $email = strtolower(mysqli_real_escape_string($db->link, $_POST["email"])); $password = mysqli_real_escape_string($db->link, $_POST["password"]); $password=md5($password); $role="subscriber"; $details=$name."\n".$email; if (empty($email) || empty($name) || empty($password)) { $error2="The username or password does not match!"; }else{ $query = "INSERT INTO lab_tblusers(name, email, password, role, pic, details) VALUES('$name','$email','$password','$role','$uploaded_pic', '$details')"; $inserted_rows = $db->insert1($query); if ($inserted_rows) { $msg="User created successfully."; }else{ $error1="not found!"; } } }else{ print_r($errors); } ?> <html lang="en"> <head> <meta charset="utf-8"> <meta content="width=device-width, initial-scale=1.0" name="viewport"> <title>Pages / Login - Shopsystem</title> <meta content="" name="description"> <meta content="" name="keywords"> <!-- Favicons --> <link href="../assets/img/favicon.png" rel="icon"> <link href="../assets/img/apple-touch-icon.png" rel="apple-touch-icon"> <!-- Google Fonts --> <link href="https://fonts.gstatic.com" rel="preconnect"> <link href="https://fonts.googleapis.com/css?family=Open+Sans:300,300i,400,400i,600,600i,700,700i|Nunito:300,300i,400,400i,600,600i,700,700i|Poppins:300,300i,400,400i,500,500i,600,600i,700,700i" rel="stylesheet"> <!-- Vendor CSS Files --> <link href="../assets/vendor/bootstrap/css/bootstrap.min.css" rel="stylesheet"> <link href="../assets/vendor/bootstrap-icons/bootstrap-icons.css" rel="stylesheet"> <link href="../assets/vendor/boxicons/css/boxicons.min.css" rel="stylesheet"> <link href="../assets/vendor/quill/quill.snow.css" rel="stylesheet"> <link href="../assets/vendor/quill/quill.bubble.css" rel="stylesheet"> <link href="../assets/vendor/remixicon/remixicon.css" rel="stylesheet"> <link href="../assets/vendor/simple-datatables/style.css" rel="stylesheet"> <link href="../assets/css/style.css" rel="stylesheet"> </head> <body> <main> <div class="container"> <section class="section register min-vh-100 d-flex flex-column align-items-center justify-content-center py-4"> <div class="container"> <div class="row justify-content-center"> <div class="col-lg-4 col-md-6 d-flex flex-column align-items-center justify-content-center"> <div class="card mb-3"> <div class="card-body"> <div class="pt-4 pb-2"> <h5 class="card-title text-center pb-0 fs-4">Create an Account</h5> <p class="text-center small">Enter your personal details to create account</p> </div> <form method="post" enctype="multipart/form-data" action="signup.php" class="row g-3 needs-validation" novalidate> <div class="col-12"> <label for="yourName" class="form-label">Your Name</label> <input type="text" name="name" class="form-control" id="yourName" required> <div class="invalid-feedback">Please, enter your name!</div> </div> <div class="col-12"> <label for="yourEmail" class="form-label">Your Email</label> <input type="email" name="email" class="form-control" id="yourEmail" required> <div class="invalid-feedback">Please enter a valid Email adddress!</div> </div> <div class="col-12"> <label for="yourPassword" class="form-label">Password</label> <input type="password" name="password" class="form-control" id="yourPassword" required> <div class="invalid-feedback">Please enter your password!</div> </div> <div class="form-group"> <label for="inputNumber" class="form-label">Image</label> <div> <input type="file" name="uploadFile" class="form-control"> </div> </div> <div class="col-12"> <div class="form-check"> <input class="form-check-input" name="terms" type="checkbox" value="" id="acceptTerms" required> <label class="form-check-label" for="acceptTerms">I agree and accept the <a href="agb.php">terms and conditions</a></label> <div class="invalid-feedback">You must agree before submitting.</div> </div> </div> <?php if (isset($msg)) { echo"<div class='alert alert-success' role='alert'> <span class='glyphicon glyphicon-exclamation-sign' aria-hidden='true'></span> <span class='sr-only'></span>".$msg."</div>"; } ?> <?php if (isset($error1)) { echo"<div class='alert alert-danger' role='alert'> <span class='glyphicon glyphicon-exclamation-sign' aria-hidden='true'></span> <span class='sr-only'></span>".$error1."</div>"; } ?> <?php if (isset($error2)) { echo"<div class='alert alert-danger' role='alert'> <span class='glyphicon glyphicon-exclamation-sign' aria-hidden='true'></span> <span class='sr-only'></span>".$error2."</div>"; } ?> <div class="form-group"> <button class="btn btn-primary w-100" type="submit">Create Account</button> </div> <div class="form-group"> <h5><p class="small mb-0">Already have an account? <a href="login.php">Log in</a></p></h5> </div> </form> </div><!-- End of card-body--> </div><!-- End of card--> </div> </div> </div> </section> </div> </main><!-- End #main --> <a href="#" class="back-to-top d-flex align-items-center justify-content-center"><i class="bi bi-arrow-up-short"></i></a> <!-- Vendor JS Files --> <script src="../assets/vendor/apexcharts/apexcharts.min.js"></script> <script src="../assets/vendor/bootstrap/js/bootstrap.bundle.min.js"></script> <script src="../assets/vendor/chart.js/chart.umd.js"></script> <script src="../assets/vendor/echarts/echarts.min.js"></script> <script src="../assets/vendor/quill/quill.min.js"></script> <script src="../assets/vendor/simple-datatables/simple-datatables.js"></script> <script src="../assets/vendor/tinymce/tinymce.min.js"></script> <script src="../assets/vendor/php-email-form/validate.js"></script> <script src="../assets/js/main.js"></script> </body> </html>
Datenbankanwendung
Für das Blogsystem nutzen wir also MySQL. Da in diesem Blogsystem die Tabellen lab_tblcategories, lab_tblsubcategories, lab_tblarticles, lab_tblusers, lab_tblorders, lab_tblorderitems, lab_tblcustomers und lab_tbldeliveries verwalten werden sollen, soll das System auch ein nutzerabhängiges Blogsystem sein. Das Tabellenschema zeigt ebenfalls die Beziehungen der Tabellen zueinander.
Tabellenschema für das Blogsystem
User-Tabelle
Die Tabelle kann man entweder mittels phpMyAdmin (Hilfsprogramm für die Verwaltung der Tabellen) erstellen oder unten stehenden SQL-Code kopieren und ausführen.Die Datenbanktabelle (lab_tblusers) hat folgende Spalten:

Datenbanktabelle lab_tblusers
Hier der SQL-Code zur Erzeugung der Datenbanktabelle (lab_tblusers).CREATE TABLE IF NOT EXISTS `lab_tblusers` (
`id` int(5) NOT NULL,
`name` varchar(50) NOT NULL,
`email` varchar(100) NOT NULL,
`password` varchar(100) NOT NULL,
`token` varchar(255) NOT NULL,
`role` varchar(20) NOT NULL,
`pic` varchar(100) NOT NULL,
`details` varchar(100) NOT NULL,
`modified` timestamp NOT NULL DEFAULT CURRENT_TIMESTAMP,
`approved` varchar(20) NOT NULL DEFAULT 'no'
) ENGINE=MyISAM AUTO_INCREMENT=1 DEFAULT CHARSET=utf8;
ALTER TABLE `lab_tblusers`
ADD PRIMARY KEY (`id`);
ALTER TABLE `lab_tblusers`
MODIFY `id` int(5) NOT NULL AUTO_INCREMENT,AUTO_INCREMENT=1;
Tabelle für die Kategorie (lab_tblcategories)
Um die Artikeldaten einem Kategorienamen bzw. Unterkategorienamen zuzuordnen, legen wir eine Tabelle mit folgenden Feldern fest:
Datenbanktabelle (lab_tblcategories)
Hier der SQL-Code zur Erzeugung der Datenbanktabelle (lab_tblcategories).CREATE TABLE IF NOT EXISTS `lab_tblcategories` (
`catid` int(3) NOT NULL,
`catname` varchar(30) NOT NULL,
`activ` tinyint(1) NOT NULL
) ENGINE=InnoDB AUTO_INCREMENT=1 DEFAULT CHARSET=latin1;
ALTER TABLE `lab_tblcategories`
ADD PRIMARY KEY (`catid`), ADD KEY `id` (`catid`);
ALTER TABLE `lab_tblcategories`
MODIFY `catid` int(3) NOT NULL AUTO_INCREMENT,AUTO_INCREMENT=1;
Tabelle für die Unterkategorie (lab_tblsubcategories)
Um die Artikeldaten einem Kategorienamen bzw. Unterkategorienamen zuzuordnen, legen wir eine Tabelle mit folgenden Feldern fest:Mit dem Feld (catid) kann die Beziehung zu der Tabelle (lab_tblcategories) hergestellt werden.

Datenbanktabelle (lab_tblsubcategories)
Hier der SQL-Code zur Erzeugung der Datenbanktabelle (lab_tblsubcategories).CREATE TABLE IF NOT EXISTS `lab_tblsubcategories` ( `subcatid` int(3) NOT NULL, `subcatname` varchar(100) CHARACTER SET latin1 NOT NULL, `catid` int(2) DEFAULT NULL, `activ` tinyint(1) NOT NULL ) ENGINE=InnoDB AUTO_INCREMENT=1 DEFAULT CHARSET=latin1 COLLATE=latin1_general_ci; ALTER TABLE `lab_tblsubcategories` ADD PRIMARY KEY (`subcatid`); ALTER TABLE `lab_tblsubcategories`
Tabelle für die Artikeldaten (lab_tblarticles)
Um die Artikeldaten zu verwalten, legen wir eine Tabelle mit folgenden Feldern fest:Mit dem Feld(subcatid) und mit dem Feld(userid) können jeweils die Beziehung zu der Tabelle (lab_tblcategories) und zu der Tabelle (lab_tblusers( hergestellt werden.
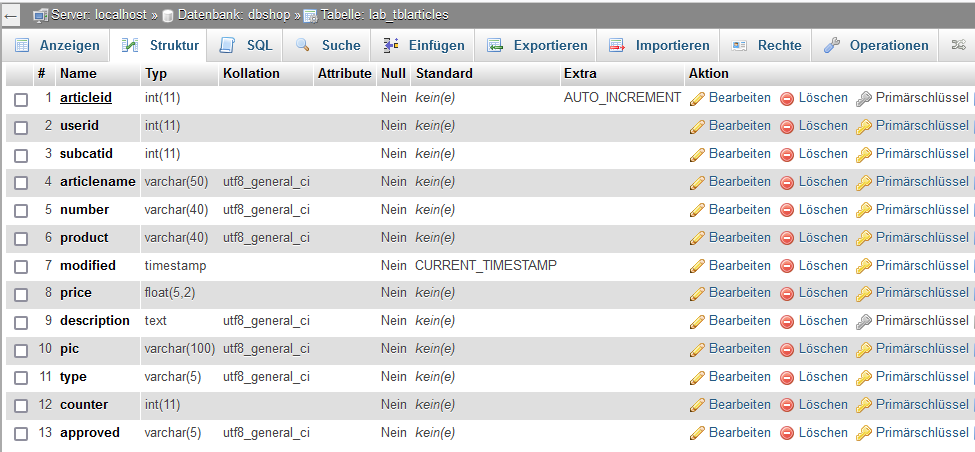
Datenbanktabelle (lab_tblarticles)
Hier der SQL-Code zur Erzeugung der Datenbanktabelle (lab_tblarticles).
CREATE TABLE IF NOT EXISTS `lab_tblarticles` (
`articleid` int(11) NOT NULL,
`userid` int(5) NOT NULL,
`subcatid` int(11) NOT NULL,
`articlename` varchar(100) NOT NULL,
`number` varchar(150) NOT NULL,
`product` varchar(100) NOT NULL,
`modified` timestamp NOT NULL DEFAULT CURRENT_TIMESTAMP,
`price` float(5,2) NOT NULL,
`description` mediumtext NOT NULL,
`pic` varchar(100) NOT NULL,
`type` varchar(5) NOT NULL,
`counter` int(11) NOT NULL,
`approved` varchar(5) NOT NULL DEFAULT 'no'
) ENGINE=MyISAM AUTO_INCREMENT=1 DEFAULT CHARSET=utf8;
ALTER TABLE `lab_tblarticles`
ADD PRIMARY KEY (`articleid`);
ALTER TABLE `lab_tblarticles`
MODIFY `articleid` int(11) NOT NULL AUTO_INCREMENT,AUTO_INCREMENT=1;
Tabelle für die Auftragsdaten (lab_tblorders)
Um die Auftragsdaten zu verwalten, legen wir eine Tabelle mit folgenden Feldern fest: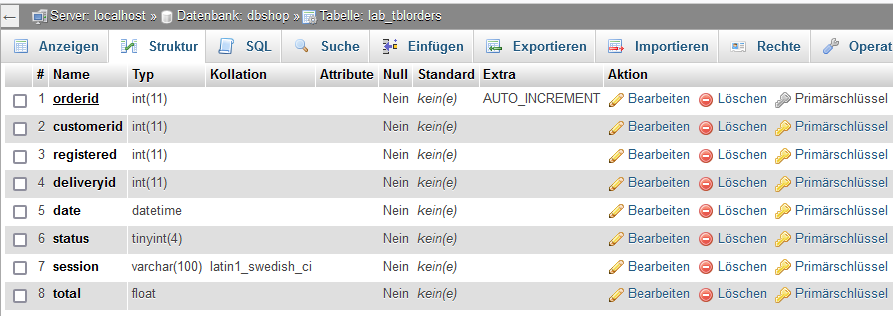
Datenbanktabelle (lab_tblorders)
Hier der SQL-Code zur Erzeugung der Datenbanktabelle (lab_tblorders).
CREATE TABLE IF NOT EXISTS `lab_tblorders` (
`orderid` int(11) NOT NULL,
`customerid` int(11) NOT NULL,
`registered` int(11) NOT NULL,
`deliveryid` int(11) NOT NULL,
`date` datetime NOT NULL,
`status` tinyint(4) NOT NULL,
`session` varchar(100) CHARACTER SET latin1 NOT NULL,
`total` float NOT NULL
) ENGINE=InnoDB AUTO_INCREMENT=1 DEFAULT CHARSET=latin1 COLLATE=latin1_general_ci;
ALTER TABLE `lab_tblorders`
ADD PRIMARY KEY (`orderid`);
ALTER TABLE `lab_tblorders`
MODIFY `orderid` int(11) NOT NULL AUTO_INCREMENT,AUTO_INCREMENT=1;
Tabelle für die Auftraglisten (lab_tblorderitems)
Um die Auftraglisten zu verwalten, legen wir eine Tabelle mit folgenden Feldern fest: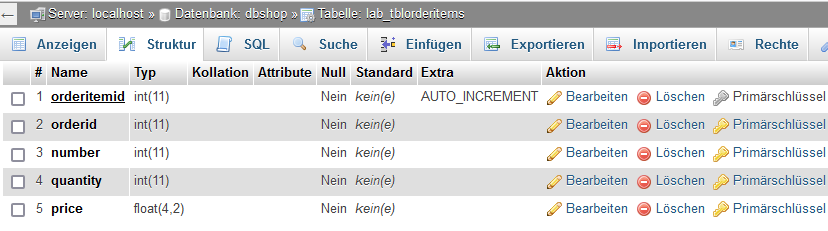
Datenbanktabelle (lab_tblorderitems)
Hier der SQL-Code zur Erzeugung der Datenbanktabelle (lab_tblorderitems).
CREATE TABLE IF NOT EXISTS `lab_tblorderitems` (
`orderitemid` int(11) NOT NULL,
`orderid` int(11) NOT NULL,
`number` int(11) NOT NULL,
`quantity` int(11) NOT NULL,
`price` float(4,2) NOT NULL
) ENGINE=InnoDB AUTO_INCREMENT=1 DEFAULT CHARSET=latin1 COLLATE=latin1_general_ci;
ALTER TABLE `lab_tblorderitems`
ADD PRIMARY KEY (`orderitemid`);
ALTER TABLE `lab_tblorderitems`
MODIFY `orderitemid` int(11) NOT NULL AUTO_INCREMENT,AUTO_INCREMENT=1;
Tabelle für die Kundendaten (lab_tblcustomers)
Um die Kundendaten zu verwalten, legen wir eine Tabelle mit folgenden Feldern fest: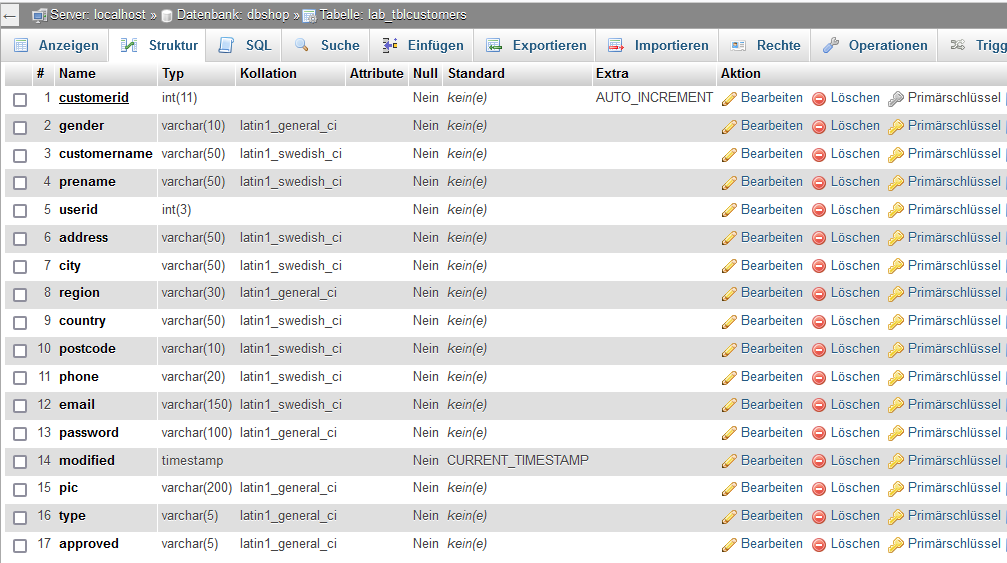
Datenbanktabelle (lab_tblcustomers)
Hier der SQL-Code zur Erzeugung der Datenbanktabelle (lab_tblcustomers).
CREATE TABLE IF NOT EXISTS `lab_tblcustomers` (
`customerid` int(11) NOT NULL,
`gender` varchar(10) COLLATE latin1_general_ci NOT NULL,
`customername` varchar(50) CHARACTER SET latin1 NOT NULL,
`prename` varchar(50) CHARACTER SET latin1 NOT NULL,
`userid` int(3) NOT NULL,
`address` varchar(50) CHARACTER SET latin1 NOT NULL,
`city` varchar(50) CHARACTER SET latin1 NOT NULL,
`region` varchar(30) COLLATE latin1_general_ci NOT NULL,
`country` varchar(50) CHARACTER SET latin1 NOT NULL,
`postcode` varchar(10) CHARACTER SET latin1 NOT NULL,
`phone` varchar(20) CHARACTER SET latin1 NOT NULL,
`email` varchar(150) CHARACTER SET latin1 NOT NULL,
`password` varchar(100) COLLATE latin1_general_ci NOT NULL,
`modified` timestamp NOT NULL DEFAULT CURRENT_TIMESTAMP,
`pic` varchar(200) COLLATE latin1_general_ci NOT NULL,
`type` varchar(5) COLLATE latin1_general_ci NOT NULL,
`approved` varchar(5) NOT NULL DEFAULT 'no'
) ENGINE=InnoDB AUTO_INCREMENT=1 DEFAULT CHARSET=latin1 COLLATE=latin1_general_ci;
ALTER TABLE `lab_tblcustomers`
ADD PRIMARY KEY (`customerid`);
ALTER TABLE `lab_tblcustomers`
MODIFY `customerid` int(11) NOT NULL AUTO_INCREMENT,AUTO_INCREMENT=1;
Tabelle für die Lieferantendaten (lab_tbldeliveries)
Um die Lieferantendaten zu verwalten, legen wir eine Tabelle mit folgenden Feldern fest:
Datenbanktabelle (lab_tbldeliveries)
Hier der SQL-Code zur Erzeugung der Datenbanktabelle (lab_tbldeliveries).
CREATE TABLE IF NOT EXISTS `lab_tbldeliveries` (
`deliveryid` int(11) NOT NULL,
`deliveryname` varchar(50) CHARACTER SET latin1 NOT NULL,
`prename` varchar(50) CHARACTER SET latin1 NOT NULL,
`gender` varchar(10) COLLATE latin1_general_ci NOT NULL,
`userid` int(3) NOT NULL,
`address` varchar(50) CHARACTER SET latin1 NOT NULL,
`city` varchar(50) CHARACTER SET latin1 NOT NULL,
`region` varchar(30) COLLATE latin1_general_ci NOT NULL,
`country` varchar(50) CHARACTER SET latin1 NOT NULL,
`postcode` varchar(10) CHARACTER SET latin1 NOT NULL,
`phone` varchar(20) CHARACTER SET latin1 NOT NULL,
`email` varchar(150) CHARACTER SET latin1 NOT NULL,
`modified` timestamp NOT NULL DEFAULT CURRENT_TIMESTAMP,
`pic` varchar(200) COLLATE latin1_general_ci NOT NULL,
`type` varchar(5) COLLATE latin1_general_ci NOT NULL,
`approved` varchar(5) NOT NULL DEFAULT 'no'
) ENGINE=InnoDB AUTO_INCREMENT=1 DEFAULT CHARSET=latin1 COLLATE=latin1_general_ci;
ALTER TABLE `lab_tbldeliveries`
ADD PRIMARY KEY (`deliveryid`);
ALTER TABLE `lab_tbldeliveries`
MODIFY `deliveryid` int(11) NOT NULL AUTO_INCREMENT,AUTO_INCREMENT=1;
Das Formular, um den Benutzer-Daten in die Datenbank hinzuzufügen (admin/adduser.php)
Hier hat nur der Hauptbenutzer Zugriff und er kann neue Datensätze in alle Tabellen u.a (lab_tblusers) hinzufügen.
Benutzer-Formular für einen neuen Datensatz
Der Inhalt der Datei (admin/adduser.php)<?php include("header.php"); include("nav.php"); $uploadDirectory = __DIR__."/"; ?> <main id="main" class="main"> <div class="pagetitle"> <nav> <ol class="breadcrumb"> <li class="breadcrumb-item"><a href="index.php">Management</a></li> <li class="breadcrumb-item">User</li> <li class="breadcrumb-item active">Add new user</li> </ol> </nav> </div><!-- End Page Title --> <section class="section"> <div class="row"> <div class="col-lg-1 col-md-1"></div> <div class="col-lg-10 col-md-10"> <div class="card"> <div class="card-body"> <h2>Add new user</h2> <?php if(isset($_FILES["uploadFile"])){ $errors= array(); $file_name = $_FILES["uploadFile"]["name"]; $file_size = $_FILES["uploadFile"]["size"]; $file_tmp = $_FILES["uploadFile"]["tmp_name"]; $file_type = $_FILES["uploadFile"]["type"]; $file_ext=strtolower(end(explode(".",$_FILES["uploadFile"]["name"]))); $unique_pic = substr(md5(time()), 0, 10).".".$file_ext; $uploaded_pic = "uploads/users/".$unique_pic; if(empty($errors)==true) { $file_name = mysqli_real_escape_string($db->link, $file_name); $fileExists = true; while ($fileExists) { if (!file_exists($uploadDirectory.$uploaded_pic)) { $fileExists = false; } } move_uploaded_file($file_tmp,$uploadDirectory.$uploaded_pic); }else { $uploaded_pic = "uploads/users/user_pda.png"; } $userid=Session::get("id"); $name = mysqli_real_escape_string($db->link, $_POST["name"]); $email = strtolower(mysqli_real_escape_string($db->link, $_POST["email"])); $password = mysqli_real_escape_string($db->link, $_POST["password"]); $password=md5($password); $role= mysqli_real_escape_string($db->link, $_POST["role"]); $details=$name.'\n'.$email; if (empty($role) || empty($email) || empty($name) || empty($password)) { echo "<span class='error'>Field must not be empty</span>"; }else{ $query = "INSERT INTO lab_tblusers(name, email, password, role, pic, details) VALUES('$name','$email','$password','$role','$uploaded_pic', '$details')"; $inserted_rows = $db->insert1($query); if ($inserted_rows) { echo'<span class="success">The new user was added successfully.</span>'; }else{ echo'<span class="success">There was an error in adding the user.</span>'; } } }else{ print_r($errors); } ?> <form class="forms-sample" action="adduser.php" method="POST" enctype="multipart/form-data"> <div class="form-group"> <label for="exampleInputName" class="form-label">Your name</label> <input type="text" name="name" class="form-control" id="exampleInputName" placeholder="Mehdi Bandegani"> </div> <div class="form-group"> <label for="exampleInputEmail" class="form-label">Email</label> <input type="email" name="email" class="form-control" id="exampleInputEmail" placeholder="mehdi@bandegani.de"> </div> <div class="form-group"> <label for="exampleInputPassword" class="form-label">Password</label> <input type="password" class="form-control" name="password" id="exampleInputPassword"> </div> <div class="form-group"> <label for="exampleInputRole" class="form-label required">Role</label> <select name="role" id="exampleInputRole" class="form-select"> <option value="0"> Select Role</option> <option value="admin">Admin</option> <option value="editor">Editor</option> <option value="subscriber">Subscriber</option> </select> </div> <div class="form-group"> <label for="inputNumber" class="col-sm-2 col-form-label">Your Image</label> <input type="file" name="uploadFile" class="form-control"> </div> <button class="btn btn-primary" type="submit" name="submit">Add user</button> </form> </div> </div><!-- Ende von Card --> </div> <div class="col-lg-1 col-md-1"></div> </div><!-- Ende von Row --> </section> </main> <?php include("footer.php"); ?>
Das Formular, um den Benutzer-Daten zu listen, zu ändern und zu löschen. (admin/userlist.php)
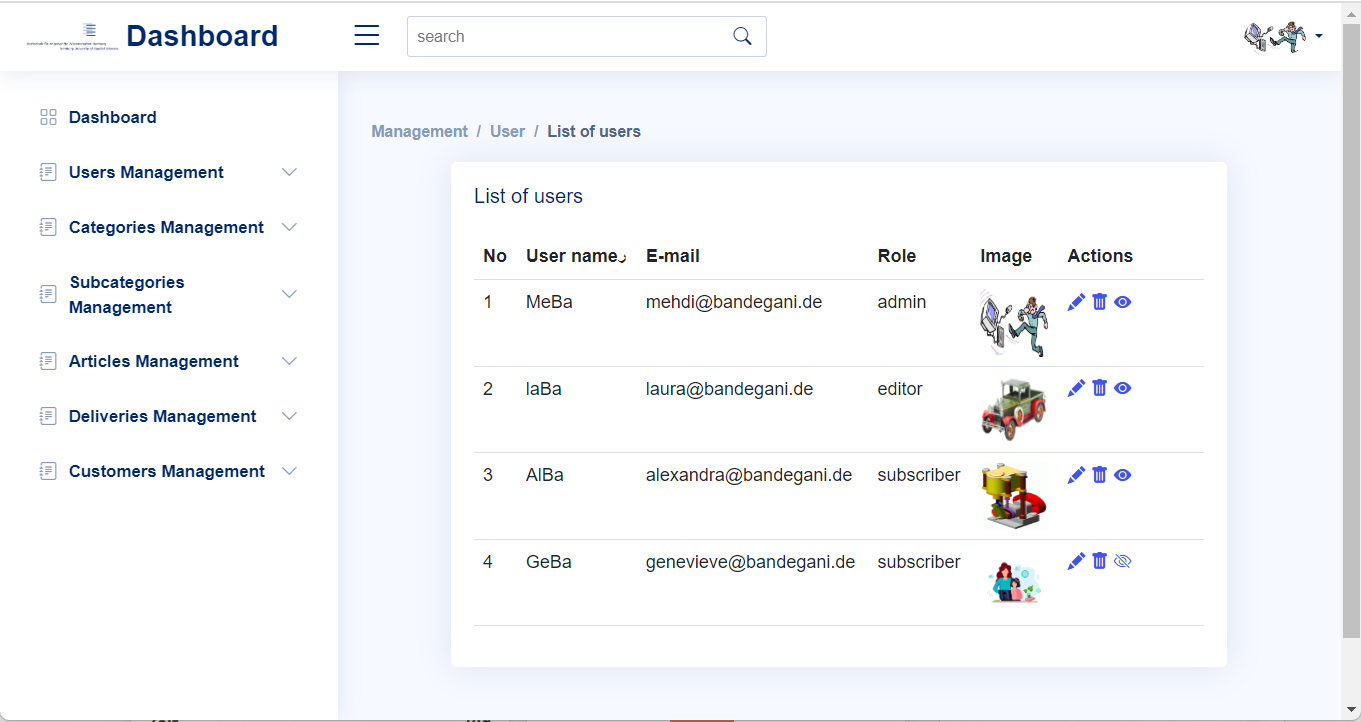
Benutzer-Formular für die Liste der Benutzer-Daten
Der Inhalt der Datei (admin/userlist.php)<?php include("header.php"); include("nav.php"); include "inc/approveanduser.php"; $uploadDirectory = __DIR__."/"; ?> <main id="main" class="main"> <div class="pagetitle"> <nav> <ol class="breadcrumb"> <li class="breadcrumb-item"><a href="index.php">Management</a></li> <li class="breadcrumb-item">User</li> <li class="breadcrumb-item active">List of users</li> </ol> </nav> </div><!-- End Page Title --> <section class="section"> <div class="row"> <div class="col-lg-1 col-md-1"></div> <div class="col-lg-10 col-md-10"> <div class="card"> <div class="card-body"> <h2 class="card-title">List of users</h2> <table class="table datatable"> <thead> <tr> <th scope="col">No</th> <th scope="col">User nameر</th> <th scope="col">E-mail</th> <th scope="col">Role</th> <th scope="col">Image</th> <th scope="col" width="20%">Actions</th> </tr> </thead> <tbody> <?php $query="SELECT * FROM lab_tblusers"; $user=$db->select($query); if($user){ while($result=$user->fetch_assoc()){ $id=$result["id"]; ?> <tr> <td scope="row"><?php echo $result["id"];?></td> <td><?php echo $result["name"];?></td> <td><?php echo $result["email"];?></td> <td><?php echo $result["role"];?></td> <td><img src="<?php echo $result["pic"];?>" style="text-align:center;height:60px; width:60px;"></td> <td> <div class="icon"> <a href="edituser.php?edituserid=<?php echo $result["id"]; ?>" data-bs-toggle="tooltip" data-bs-placement="bottom" title="Edit"> <i class="bi bi-pencil-fill"></i> </a> <a href="javascript: delete_userid(<?php echo $id;?>)" data-bs-toggle="tooltip" data-bs-placement="bottom" title="Delete"> <i class="bi bi-trash-fill"></i> </a> <?php if ($result["approved"]=="yes"){ echo '<a href="userlist.php?rejectuserid='.$id. '" data-bs-toggle="tooltip" data-bs-placement="bottom" title="Rejected"> <i class="bi bi-eye-fill"></i> </a>' ; }else{ echo '<a href="userlist.php?approveuserid='.$id.'" data-bs-toggle="tooltip" data-bs-placement="bottom" title="Approved"> <i class="bi bi-eye-slash"></i> </a>'; } ?> </td> </div> </tr> <?php } }else{ echo "There are no users to display!"; } ?> </tbody> </table> </div> </div><!-- End Card --> </div><!-- End col-10 --> <div class="col-lg-1 col-md-1"></div> </div><!-- End row--> </section> </main> <script type="text/javascript"> function delete_userid(deluserid) { if (confirm('Delete this user?' )) { window.location.href = 'userlist.php?deluserid=' + deluserid; } } </script> <?php include("footer.php"); ?>
Das Formular, um den Benutzer-Daten zu ändern. (admin/edituser.php)

Benutzer-Formular für das Ändern der Benutzer-Daten
Der Inhalt der Datei (admin/edituser.php)<?php include("header.php"); include("nav.php"); $uploadDirectory = __DIR__."/"; if(isset($_POST["userid"])){ $userid = $_POST["userid"]; }else if(!isset($_GET["edituserid"]) || $_GET["edituserid"]==NULL){ echo '<script> window.location="userlist.php"</script>'; }else{ $userid = $_GET["edituserid"]; } ?> <main id="main" class="main"> <div class="pagetitle"> <nav> <ol class="breadcrumb"> <li class="breadcrumb-item"><a href="index.php">Management</a></li> <li class="breadcrumb-item">User</li> <li class="breadcrumb-item active">Edit User </li> </ol> </nav> </div><!-- End Page Title --> <section class="section"> <div class="row"> <div class="col-lg-1 col-md-1"></div> <div class="col-lg-10 col-md-10"> <div class="card"> <div class="card-body"> <h2>Edit User </h2> <?php if ($_SERVER["REQUEST_METHOD"] == "POST" ) { $name = mysqli_real_escape_string($db->link, $_POST["name"]); $email = mysqli_real_escape_string($db->link, $_POST["email"]); $password = md5(mysqli_real_escape_string($db->link, $_POST["password"])); $role = mysqli_real_escape_string($db->link, $_POST["role"]); $picold=$_SESSION["oldpic"]; $errors= array(); $file_name = $_FILES["uploadFile"]["name"]; $file_size = $_FILES["uploadFile"]["size"]; $file_tmp = $_FILES["uploadFile"]["tmp_name"]; $file_type = $_FILES["uploadFile"]["type"]; $file_ext=strtolower(end(explode(".",$_FILES["uploadFile"]["name"]))); $unique_pic = substr(md5(time()), 0, 10).".".$file_ext; $uploaded_pic = "uploads/users/".$unique_pic; $picold=$_SESSION["oldpic"]; if(!empty($file_name)){ $file_name = mysqli_real_escape_string($db->link, $file_name); $type = pathinfo($file_name, PATHINFO_EXTENSION); $fileExists = true; while ($fileExists) { if (!file_exists($uploadDirectory.$uploaded_pic)) { $fileExists = false; } } if (picdelete($picold)==false){ ?> <div class="bg-darkred fg-white"> <span class="label label-danger">User Image not found!</span> </div> <?php } $mediaType = "something"; switch ($type) { case "jpg": case "jpeg": case "gif": case "png": default: $mediaType = "pic"; break; } move_uploaded_file($file_tmp,$uploadDirectory.$uploaded_pic); }else { $uploaded_pic = $picold; } $query = "UPDATE lab_tblusers SET name='$name', email='$email', role='$role' , password='$password', pic='$uploaded_pic' WHERE id='$userid'"; $updated_rows = $db->update1($query); if ($updated_rows) { echo "<span class='success'>A new user has been added. </span>"; }else{ echo "<span class='success'>A new user has not been added.</span>"; } }else { echo "<span class='error'>User not added!</span>"; } ?> <?php $query="SELECT * FROM lab_tblusers WHERE id= '$userid' "; $user=$db->select($query); if($user){ while($result=$user->fetch_assoc()){ $_SESSION["oldpic"]= $result["pic"]; $oldpic=$_SESSION["oldpic"]; $_SESSION["oldrole"]= $result["role"]; $oldrole=$_SESSION["oldrole"]; ?> <form class="forms-sample" action="edituser.php" method="POST" enctype="multipart/form-data"> <div class="form-group"> <input type="hidden" value="<?php echo $userid; ?>" class="form-control" name="userid"> </div> <div class="form-group"> <label for="exampleInputName" class="f-22">Your Name</label> <input type="text" value="<?php echo $result["name"];?>" class="form-control" name="name" id="exampleInputName"> </div> <div class="form-group"> <label for="exampleInputEmail" class="f-22">E-mail</label> <input type="email" value="<?php echo $result["email"];?>" class="form-control" name="email" id="exampleInputEmail"> </div> <div class="form-group"> <label for="exampleInputPassword" class="f-22">Password</label> <input type="password" value="<?php echo $result["password"];?>" class="form-control" name="password" id="exampleInputPassword"> </div> <div class="form-group"> <label for="exampleInputRole" class="form-label required">Role</label> <select name="role" id="exampleInputRole" class="form-select"> <option value="<?php echo $oldrole; ?>"> <?php echo $oldrole; ?></option> <option value="admin">Admin</option> <option value="editor">Editor</option> <option value="subscriber">Subscriber</option> </select> </div> <div class="form-group"> <img src="<?php echo $oldpic; ?>" width="200px" height="100px"><br/> <input type="file" name="uploadFile" class="file-upload-default"> </div> <button class="btn btn-success" type="submit" name="submit">Update</button> </form> <?php } }else{ echo "Failure within the system!"; } ?> </div> </div><!-- Ende von Card --> </div> <div class="col-lg-1 col-md-1"></div> </div><!-- Ende von Row --> </section> </main> <?php include("footer.php"); ?> <?php function picdelete($pic) { if (file_exists($pic)) { if( @unlink($pic) !== true ) throw new Exception('Could not delete file: ' . $pic . ' Please close all applications that are using it.'); } return true; } ?>
Der Inhalt der Datei (admin/inc/approveanduser.php)
<?php if(!isset($_GET["deluserid"]) || $_GET["deluserid"]==NULL){ }else{ $id = $_GET["deluserid"]; $query="DELETE FROM lab_tblusers WHERE id='$id'"; $user=$db->delete($query); } if(!isset($_GET["approveuserid"]) || $_GET["approveuserid"]==NULL){ } else{ $id = $_GET["approveuserid"]; $query="UPDATE lab_tblusers SET approved='yes' WHERE id='$id'"; $approved=$db->update($query); if($approved){ echo "<span style='color:#fff;'>User approved!</span>"; }else{ echo "<span style='color:#fff;'>User not approved!</span>"; } } if(!isset($_GET["rejectuserid"]) || $_GET["rejectuserid"]==NULL){ } else{ $id = $_GET["rejectuserid"]; $query="UPDATE lab_tblusers SET approved='no' WHERE id='$id'"; $approved=$db->update($query); if($approved){ echo "<span style='color:#fff;'>User rejected!. </span>"; }else{ echo "<span style='color:#fff;'>User not rejected. </span>"; } } ?>
Das Formular, um den Kategorie-Daten in die Datenbank hinzuzufügen (admin/addcat.php)
Hier haben alle Benutzer Zugriff. Sie können neue Datensätze in alle Tabellen u.a (lab_tblcategories) hinzufügen.
Kategorie-Formular für einen neuen Datensatz
Der Inhalt der Datei (admin/addcat.php)<?php include("header.php"); include("nav.php"); ?> <main id="main" class="main"> <div class="pagetitle"> <nav> <ol class="breadcrumb"> <li class="breadcrumb-item"><a href="index.php">Management</a></li> <li class="breadcrumb-item">Category</li> <li class="breadcrumb-item active">Add new category</li> </ol> </nav> </div><!-- End Page Title --> <section class="section"> <div class="row"> <div class="col-lg-1 col-md-1"></div> <div class="col-lg-10 col-md-10"> <div class="card"> <div class="card-body"> <h2>Add new category</h2> <?php if ($_SERVER["REQUEST_METHOD"] == "POST") { $catname = strtolower(mysqli_real_escape_string($db->link, $_POST['catname'])); $flag=0; $query="SELECT catname FROM lab_tblcategories WHERE 1"; $catname1=$db->select($query); if($catname1){ while($result=$catname1->fetch_assoc()){ $catname2=$result['catname']; if($catname2==$catname){ $flag=1; } } } if (empty($catname) ) { echo '<div class="alert alert-danger bg-danger text-light border-0 alert-dismissible fade show" role="alert"> The field must not be empty! </div>'; }else{ if($flag==1){ echo '<div class="alert alert-success bg-success text-light border-0 alert-dismissible fade show" role="alert"> This category is available! </div>'; }else{ if(isset($_POST["activ"])) { $activ=1; }else { $activ=0; } $query = "INSERT INTO lab_tblcategories(catname, activ) VALUES('$catname', '$activ')"; $inserted_rows = $db->insert1($query); if ($inserted_rows) { echo '<div class="alert alert-success bg-success text-light border-0 alert-dismissible fade show" role="alert"> The category has been added! </div>'; }else{ echo '<div class="alert alert-danger bg-danger text-light border-0 alert-dismissible fade show" role="alert"> An error occurred while adding the category to the system! </div>'; } } } } ?> <form class="forms-sample" action="addcat.php" method="POST" enctype="multipart/form-data"> <div class="form-group"> <label for="exampleInputName" class="f-22">Categoryname</label> <input type="text" name="catname" class="form-control" id="exampleInputName" placeholder="Woman's Clothing"> </div> <div class="form-check"> <input class="form-check-input" type="checkbox" name="activ" value="true" id="exampleInputActiv"> <label class="form-check-label" for="exampleInputActiv">Activ</label> </div> <button class="btn btn-success" type="submit" name="submit">Add category</button> </form> </div> </div><!-- Ende von Card --> </div> <div class="col-lg-1 col-md-1"></div> </div><!-- Ende von Row --> </section> </main> <?php include("footer.php"); ?>
Das Formular, um den Kategorie-Daten zu listen, zu ändern und zu löschen. (admin/catlist.php)
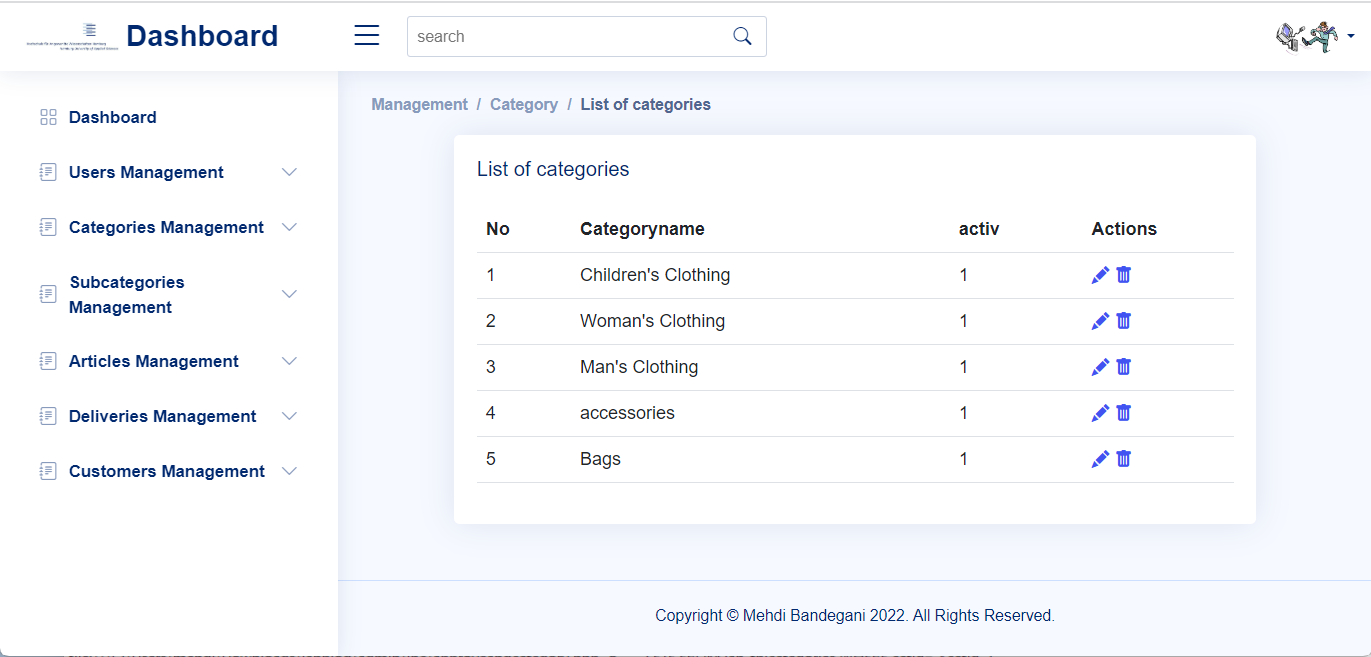
Formular für die Liste der Kategorie-Daten
Der Inhalt der Datei (admin/catlist.php)<?php include("header.php"); include("nav.php"); include ("inc/approveandcategory.php"); ?> <main id="main" class="main"> <div class="pagetitle"> <nav> <ol class="breadcrumb"> <li class="breadcrumb-item"><a href="index.php">Management</a></li> <li class="breadcrumb-item">Category</li> <li class="breadcrumb-item active">List of categories</li> </ol> </nav> </div><!-- End Page Title --> <section class="section"> <div class="row"> <div class="col-lg-1 col-md-1"></div> <div class="col-lg-10 col-md-10"> <div class="card"> <div class="card-body"> <h5 class="card-title">List of categories</h5> <table class="table datatable"> <thead> <tr> <th scope="col">No</th> <th scope="col">Categoryname</th> <th scope="col">activ</th> <th scope="col" width="20%">Actions</th> </tr> </thead> <tbody> <?php $query="SELECT * FROM lab_tblcategories"; $category=$db->select($query); if($category){ $i=0; while($result=$category->fetch_assoc()){ $i++; ?> <tr> <td><?php echo $result['catid'];?></td> <td><?php echo $result['catname'];?></td> <td><?php echo $result['activ'];?></td> <td> <div class="icon"> <a href="editcat.php?editcatid=<?php echo $result["catid"]; ?>" data-bs-toggle="tooltip" data-bs-placement="bottom" title="Edit"> <i class="bi bi-pencil-fill"></i> </a> <a href="javascript: delete_catid(<?php echo $catid;?>)" data-bs-toggle="tooltip" data-bs-placement="bottom" title="Delete"> <i class="bi bi-trash-fill"></i> </a> </div> </td> </tr> <?php } }else{ echo "There are no categories to display"; } ?> </tbody> </table> </div> </div><!-- End Card --> </div><!-- End col-10 --> <div class="col-lg-1 col-md-1"></div> </div><!-- End row--> </section> </main> <script type="text/javascript"> function delete_catid(delcatid) { if (confirm('Delete this category?' )) { window.location.href = 'catlist.php?delcatid=' + delcatid; } } </script> <?php include"footer.php"; ?>
Das Formular, um den Kategorie-Daten zu ändern. (admin/editcat.php)
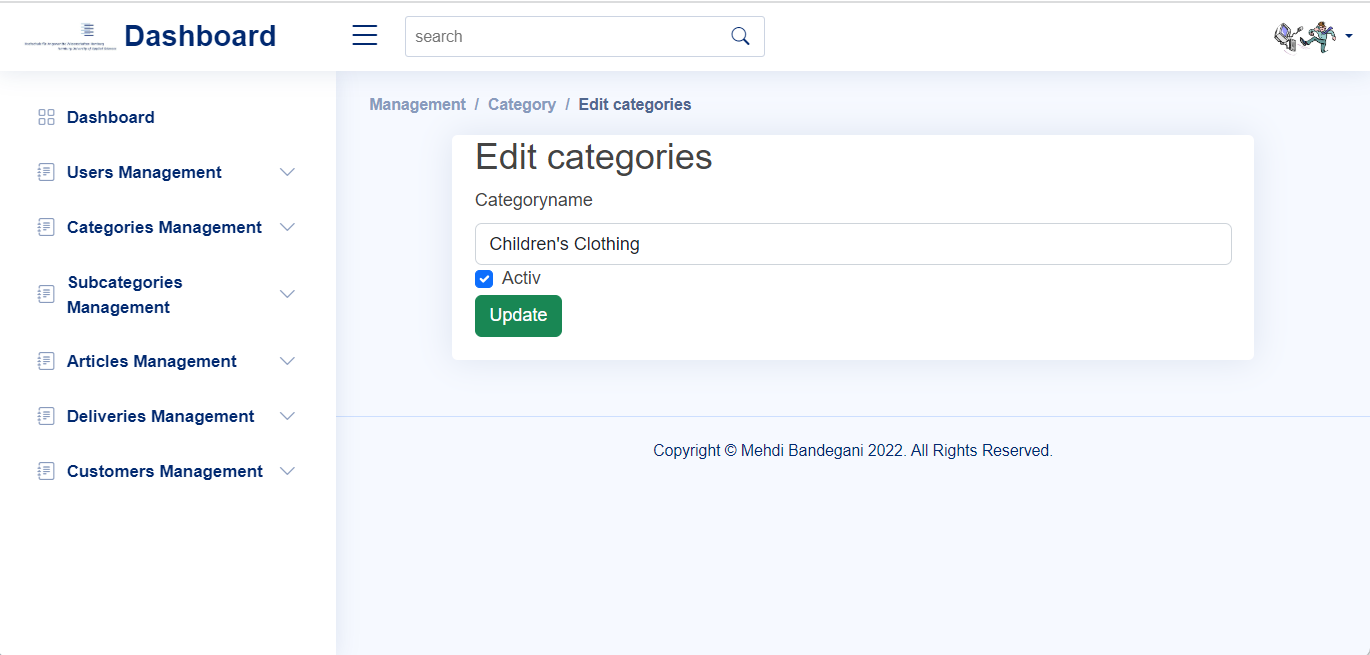
Kategorie-Formular für das Ändern der Kategorie-Daten
Der Inhalt der Datei (admin/editcat.php)<?php include("header.php"); include("nav.php"); if(isset($_POST["catid"])){ $catid = $_POST["catid"]; }elseif($_GET["editcatid"]==NULL || empty($_GET["editcatid"])){ echo '<script> window.location="catlist.php"</script>'; }else{ $catid=$_GET["editcatid"]; } ?> <main id="main" class="main"> <div class="pagetitle"> <nav> <ol class="breadcrumb"> <li class="breadcrumb-item"><a href="index.php">Management</a></li> <li class="breadcrumb-item">Category</li> <li class="breadcrumb-item active">Edit categories</li> </ol> </nav> </div><!-- End Page Title --> <section class="section"> <div class="row"> <div class="col-lg-1 col-md-1"></div> <div class="col-lg-10 col-md-10"> <div class="card"> <div class="card-body"> <h2>Edit categories</h2> <?php if ($_SERVER["REQUEST_METHOD"] == "POST") { $catname = strtolower(mysqli_real_escape_string($db->link, $_POST["catname"])); if(isset($_POST["activ"])) { $activ=1; }else { $activ=0; } $query = "UPDATE lab_tblcategories SET catname='$catname',activ='$activ' WHERE catid=$catid "; $rows = $db->update($query); if ($rows) { echo '<div class="alert alert-success bg-success text-light border-0 alert-dismissible fade show" role="alert"> The category has been updated! </div>'; }else{ echo '<div class="alert alert-danger bg-danger text-light border-0 alert-dismissible fade show" role="alert"> The category has not been updated! </div>'; } } $query1="SELECT * FROM lab_tblcategories WHERE catid='$catid'"; $rows1=$db->select($query1); if($rows1){ while($result=$rows1->fetch_assoc()){ ?> <form class="forms-sample" action="editcat.php" method="POST" enctype="multipart/form-data"> <div class="form-group"> <input type="hidden" name="catid" value="<?php echo $catid ?>" /> </div> <div class="form-group"> <label for="exampleInputName" class="form-label">Categoryname</label> <input type="text" name="catname" value ="<?php echo $result["catname"] ?>" class="form-control" id="exampleInputName"> </div> <?php if ($result["activ"] ==1){ ?> <div class="form-check"> <input class="form-check-input" type="checkbox" name="activ" id="exampleInputActiv" checked> <label class="form-check-label" for="exampleInputActiv">Activ</label> </div> <?php } else { ?> <div class="form-check"> <input class="form-check-input" type="checkbox" name="activ" id="exampleInputActiv"> <label class="form-check-label" for="exampleInputActiv">Activ</label> </div> <?php } ?> <button class="btn btn-success" type="submit" name="submit">Update</button> </form> <?php } } ?> </div> </div><!-- Ende von Card --> </div> <div class="col-lg-1 col-md-1"></div> </div><!-- Ende von Row --> </section> </main> <?php include("footer.php"); ?>
Der Inhalt der Datei (admin/inc/approveandcategory.php)
 <?php if(!isset($_GET["delcatid"]) || $_GET["delcatid"]==NULL){ }else{ $catid = $_GET["delcatid"]; $query="DELETE FROM lab_tblcategories WHERE catid='$catid'"; $category=$db->delete($query); if($category){ echo '<div class="alert alert-success bg-success text-light border-0 alert-dismissible fade show" role="alert"> The category has been deleted! </div>'; }else{ echo '<div class="alert alert-danger bg-danger text-light border-0 alert-dismissible fade show" role="alert"> Category could not be deleted! </div>'; } } ?>
Das Formular, um den Unterkategorie-Daten in die Datenbank hinzuzufügen (admin/addsubcat.php)
Hier haben alle Benutzer Zugriff. Sie können neue Datensätze in alle Tabellen u.a (lab_tblsubcategories) hinzufügen.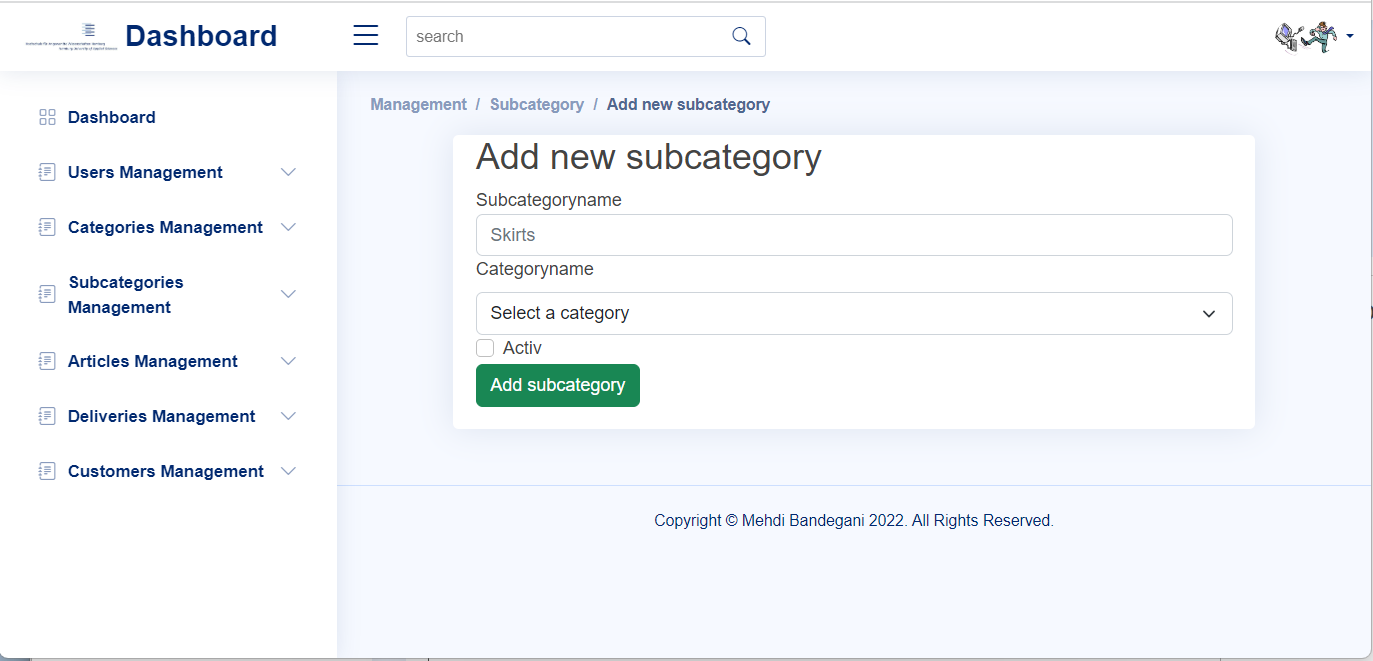
Unterkategorie-Formular für einen neuen Datensatz
Der Inhalt der Datei (admin/addsubcat.php)<?php include("header.php"); include("nav.php"); ?> <main id="main" class="main"> <div class="pagetitle"> <nav> <ol class="breadcrumb"> <li class="breadcrumb-item"><a href="index.php">Management</a></li> <li class="breadcrumb-item">Subcategory</li> <li class="breadcrumb-item active">Add new subcategory</li> </ol> </nav> </div><!-- End Page Title --> <section class="section"> <div class="row"> <div class="col-lg-1 col-md-1"></div> <div class="col-lg-10 col-md-10"> <div class="card"> <div class="card-body"> <h2>Add new subcategory</h2> <?php if ($_SERVER["REQUEST_METHOD"] == "POST") { $catname = mysqli_real_escape_string($db->link, $_POST["catname"]); $subcatname = strtoupper(mysqli_real_escape_string($db->link, $_POST["subcatname"])); $flag=0; $query="SELECT subcatname FROM lab_tblsubcategories WHERE 1"; $subcatname1=$db->select($query); if($subcatname1){ while($result=$subcatname1->fetch_assoc()){ $subcatname2=$result["subcatname"]; if($subcatname2==$subcatname){ $flag=1; } } } if (empty($catname) || empty($subcatname) ) { echo '<div class="alert alert-danger bg-danger text-light border-0 alert-dismissible fade show" role="alert"> The field must not be empty! </div>'; } else{ if($flag==1){ echo '<div class="alert alert-success bg-success text-light border-0 alert-dismissible fade show" role="alert"> This subcategory is available! </div>'; }else{ if(isset($_POST["activ"])) { $activ=1; }else { $activ=0; } $query= "INSERT INTO lab_tblsubcategories(subcatname, catid, activ) VALUES('$subcatname','$catname', '$activ')"; $inserted_rows = $db->insert1($query); if ($inserted_rows) { echo '<div class="alert alert-success bg-success text-light border-0 alert-dismissible fade show" role="alert"> The subcategory has been added! </div>'; }else{ echo '<div class="alert alert-danger bg-danger text-light border-0 alert-dismissible fade show" role="alert"> An error occurred while adding the subcategory to the system! </div>'; } } } } ?> <form class="forms-sample" action="addsubcat.php" method="POST" enctype="multipart/form-data"> <div class="form-group"> <label for="exampleInputName" class="f-22">Subcategoryname</label> <input type="text" name="subcatname" class="form-control" id="exampleInputName" placeholder="Skirts"> </div> <?php $query="SELECT catid, catname FROM lab_tblcategories WHERE 1"; $category=$db->select($query); ?> <div class="form-group"> <label for="inputCatname" class="form-label">Categoryname</label> <select class="form-select" name="catname" id="inputCatname"> <option value="0"> Select a category</option> <?php if($category){ while($result=$category->fetch_assoc()){ ?> <option value="<?php echo $result["catid"];?>"><?php echo $result["catname"];?></option> <?php }?> <?php }else{ echo "Failure within the system"; }?> </select> </div> <div class="form-check"> <input class="form-check-input" type="checkbox" name="activ" value="true" id="exampleInputActiv"> <label class="form-check-label" for="exampleInputActiv">Activ</label> </div> <button class="btn btn-success" type="submit" name="submit">Add subcategory</button> </form> </div> </div><!-- Ende von Card --> </div> <div class="col-lg-1 col-md-1"></div> </div><!-- Ende von Row --> </section> </main> <?php include("footer.php"); ?>
Das Formular, um den Unterkategorie-Daten zu listen, zu ändern und zu löschen. (admin/subcatlist.php)

Formular für die Liste der Unterkategorie-Daten
Der Inhalt der Datei (admin/subcatlist.php)<?php include("header.php"); include("nav.php"); include ("inc/approveandsubcategory.php"); ?> <main id="main" class="main"> <div class="pagetitle"> <nav> <ol class="breadcrumb"> <li class="breadcrumb-item"><a href="index.php">Management</a></li> <li class="breadcrumb-item">Subcategory</li> <li class="breadcrumb-item active">List of subcategories</li> </ol> </nav> </div><!-- End Page Title --> <section class="section"> <div class="row"> <div class="col-lg-1 col-md-1"></div> <div class="col-lg-10 col-md-10"> <div class="card"> <div class="card-body"> <h5 class="card-title">List of subcategories</h5> <table class="table datatable"> <thead> <tr></tr> <th scope="col">No</th> <th scope="col">Subcategoryname</th> <th scope="col">Categoryname</th> <th scope="col">activ</th> <th scope="col" width="20%">Actions</th> </tr> </thead> <tbody> <?php $query = "SELECT lab_tblsubcategories.subcatid, lab_tblsubcategories.subcatname, lab_tblsubcategories.catid, lab_tblsubcategories.activ, lab_tblcategories.catid , lab_tblcategories.catname FROM lab_tblsubcategories INNER JOIN lab_tblcategories ON lab_tblsubcategories.catid=lab_tblcategories.catid ORDER BY lab_tblsubcategories.subcatid " . "ASC"; $category=$db->select($query); if($category){ while($result=$category->fetch_assoc()){ ?> <tr> <td scope="row"><?php echo $result["subcatid"]; ?></td> <td><?php echo $result["subcatname"]; ?></td> <td><?php echo $result["catname"]; ?></td> <td><?php echo $result["activ"]; ?></td> <td> <div class="icon"> <a href="editsubcat.php?editsubcatid=<?php echo $result["subcatid"]; ?>" data-bs-toggle="tooltip" data-bs-placement="bottom" title="Edit"> <i class="bi bi-pencil-fill"></i> </a> <a href="javascript: delete_subcatid(<?php echo $result["subcatid"];?>)" data-bs-toggle="tooltip" data-bs-placement="bottom" title="Delete"> <i class="bi bi-trash-fill"></i> </a> </div> </td> </tr> <?php } }else{ echo "There are no subcategories to display"; } ?> </tbody> </table> </div> </div><!-- End Card --> </div><!-- End col-10 --> <div class="col-lg-1 col-md-1"></div> </div><!-- End row--> </section> </main> <script type="text/javascript"> function delete_subcatid(delsubcatid) { if (confirm('Delete this subcategory?' )) { window.location.href = 'subcatlist.php?delsubcatid=' + delsubcatid; } } </script> <?php include("footer.php"); ?>
Das Formular, um den Unterkategorie-Daten zu ändern. (admin/editsubcat.php)
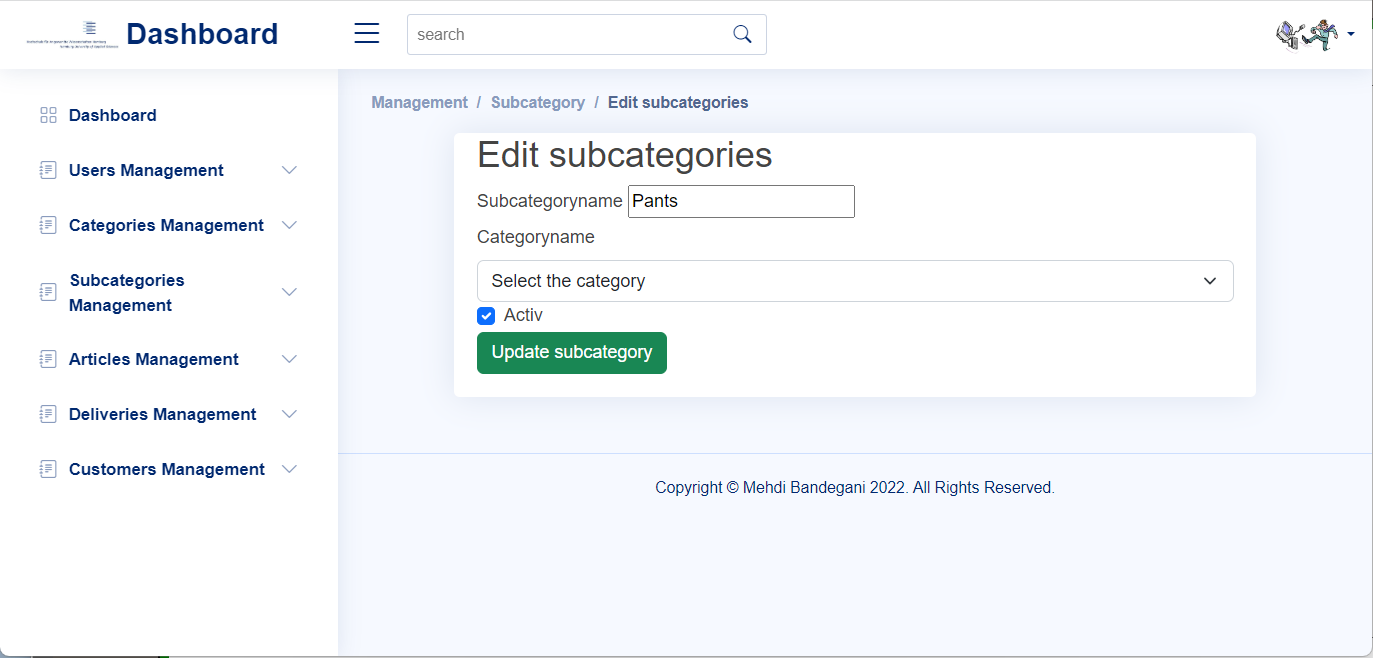
Unterkategorie-Formular für das Ändern der Unterkategorie-Daten
Der Inhalt der Datei (admin/editsubcat.php)<?php include("header.php"); include("nav.php"); if(isset($_POST["subcatid"])){ $subcatid = $_POST["subcatid"]; }elseif($_GET["editsubcatid"]==NULL || empty($_GET["editsubcatid"])){ echo '<script> window.location="subcatlist.php"</script>'; }else{ $subcatid=$_GET["editsubcatid"]; } ?> <main id="main" class="main"> <div class="pagetitle"> <nav> <ol class="breadcrumb"> <li class="breadcrumb-item"><a href="index.php">Management</a></li> <li class="breadcrumb-item">Subcategory</li> <li class="breadcrumb-item active">Edit subcategories</li> </ol> </nav> </div><!-- End Page Title --> <section class="section"> <div class="row"> <div class="col-lg-1 col-md-1"></div> <div class="col-lg-10 col-md-10"> <div class="card"> <div class="card-body"> <h2>Edit subcategories </h2> <?php if ($_SERVER["REQUEST_METHOD"] == "POST") { $catname = mysqli_real_escape_string($db->link, $_POST["catname"]); if($catname<1){ $catname=$_SESSION["catid"]; } if(isset($_POST["activ"])) { $activ=1; }else { $activ=0; } $subcatname = strtoupper(mysqli_real_escape_string($db->link, $_POST["subcatname"])); $querycatname = "UPDATE lab_tblsubcategories SET subcatname='$subcatname', catid='$catname',activ='$activ' WHERE subcatid='$subcatid'"; $updated_rows = $db->update($querycatname); if ($updated_rows) { echo '<div class="alert alert-success bg-success text-light border-0 alert-dismissible fade show" role="alert"> The category has been updated! </div>'; }else{ echo '<div class="alert alert-danger bg-danger text-light border-0 alert-dismissible fade show" role="alert"> The category has not been updated! </div>'; } } ?> <?php $query="SELECT * FROM lab_tblsubcategories WHERE subcatid='$subcatid' "; $subcatquery=$db->select($query); if($subcatquery){ while($subcatresult=$subcatquery->fetch_assoc()){ $_SESSION["catid"]=$subcatresult["catid"]; ?> <form class="forms-sample" action="editsubcat.php" method="POST" enctype="multipart/form-data"> <input type="hidden" name="subcatid" value="<?php echo $subcatid ?>" /> <div class="form-group"> <label for="exampleInputSubname" class="form-label">Subcategoryname</label> <input type="text" name="subcatname" id="exampleInputSubname" value="<?php echo $subcatresult["subcatname"];?>" /> </div> <?php $query="SELECT catid, catname FROM lab_tblcategories WHERE 1"; $category=$db->select($query); ?> <div class="form-group"> <label for="" class="form-label">Categoryname</label> <select class="form-select" name="catname"> <option value="0"> Select the category</option> <?php if($category){ while($result=$category->fetch_assoc()){ ?> <option value="<?php echo $result["catid"];?>"><?php echo $result["catname"];?></option> <?php }?> <?php }else{ echo "Failure within the system"; }?> </select> </div> <?php if ($subcatresult["activ"] ==1){ ?> <div class="form-check"> <input class="form-check-input" type="checkbox" name="activ" id="exampleInputActiv" checked> <label class="form-check-label" for="exampleInputActiv">Activ</label> </div> <?php } else { ?> <div class="form-check"> <input class="form-check-input" type="checkbox" name="activ" id="exampleInputActiv"> <label class="form-check-label" for="exampleInputActiv">Activ</label> </div> <?php } ?> <button class="btn btn-success" type="submit" name="submit">Update subcategory</button> </form> <?php } } ?> </div> </div><!-- Ende von Card --> </div> <div class="col-lg-1 col-md-1"></div> </div><!-- Ende von Row --> </section> </main> <?php include("footer.php"); ?>
Der Inhalt der Datei (admin/inc/approveandsubcategory.php)
 <?php if(!isset($_GET["delsubcatid"]) || $_GET["delsubcatid"]==NULL){ }else{ $subcatid = $_GET["delsubcatid"]; $query="DELETE FROM lab_tblsubcategories WHERE subcatid='$subcatid'"; $subcategory=$db->delete($query); if($subcategory){ echo '<div class="alert alert-success bg-success text-light border-0 alert-dismissible fade show" role="alert"> The subcategory has been deleted! </div>'; }else{ echo '<div class="alert alert-danger bg-danger text-light border-0 alert-dismissible fade show" role="alert"> Category could not be deleted! </div>'; } } ?>
Das Formular, um den Artikel-Daten in die Datenbank hinzuzufügen (admin/addarticle.php)
Hier haben alle Benutzer Zugriff. Sie können neue Datensätze in alle Tabellen u.a (lab_tblarticles) hinzufügen.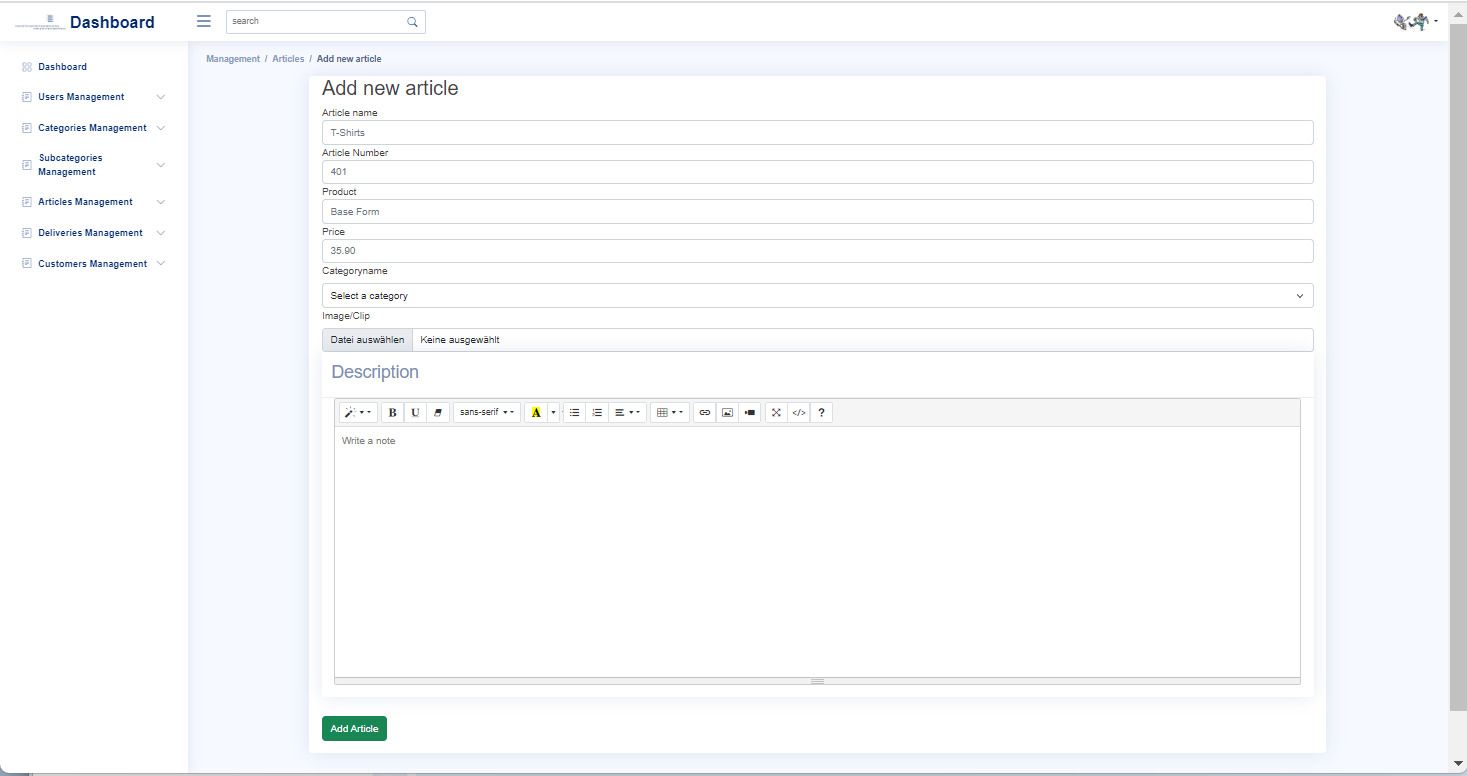
Artikel-Formular für einen neuen Datensatz
Der Inhalt der Datei (admin/addarticle.php)<?php include("header.php"); include("nav.php"); $uploadDirectory = __DIR__."/"; ?> <main id="main" class="main"> <div class="pagename"> <nav> <ol class="breadcrumb"> <li class="breadcrumb-item"><a href="index.php">Management</a></li> <li class="breadcrumb-item">Articles</li> <li class="breadcrumb-item active">Add new article</li> </ol> </nav> </div><!-- End Page Title --> <section class="section"> <div class="row"> <div class="col-lg-1 col-md-1"></div> <div class="col-lg-10 col-md-10"> <div class="card"> <div class="card-body"> <h2>Add new article</h2> <?php if(isset($_FILES["uploadFile"])){ $errors= array(); $file_name = $_FILES["uploadFile"]["name"]; $file_size = $_FILES["uploadFile"]["size"]; $file_tmp = $_FILES["uploadFile"]["tmp_name"]; $file_type = $_FILES["uploadFile"]["type"]; $file_ext=strtolower(end(explode(".",$_FILES["uploadFile"]["name"]))); $unique_pic = substr(md5(time()), 0, 10).".".$file_ext; $uploaded_pic = "uploads/articles/".$unique_pic; if(!empty($file_name)){ if(empty($errors)==true) { $file_name = mysqli_real_escape_string($db->link, $file_name); $type = pathinfo($file_name, PATHINFO_EXTENSION); $fileExists = true; while ($fileExists) { if (!file_exists($uploadDirectory.$uploaded_pic)) { $fileExists = false; } } move_uploaded_file($file_tmp,$uploadDirectory.$uploaded_pic); $mediaType = "something"; switch ($type) { case "mp4": case "mkv": case "mov": case "ogg": case "webm": $mediaType = "video"; break; case "jpg": case "jpeg": case "gif": case "png": default: $mediaType = "image"; break; } } }else { $uploaded_pic = "uploads/articles/shop_logo.gif"; $mediaType = "pic"; } $userid=Session::get("id"); $articlename= mysqli_real_escape_string($db->link, $_POST["articlename"]); $number = mysqli_real_escape_string($db->link, $_POST["number"]); $product = mysqli_real_escape_string($db->link, $_POST["product"]); $price = mysqli_real_escape_string($db->link, $_POST["price"]); $description = mysqli_real_escape_string($db->link, $_POST["description"]); $subcatid = mysqli_real_escape_string($db->link, $_POST["subcatname"]); $query = "INSERT INTO lab_tblarticles (articlename, number, product, price, description, userid, pic, type, modified, subcatid) VALUES('$articlename', '$number', '$product', '$price', '$description', '$userid','$uploaded_pic', '$mediaType', NOW(), '$subcatid')"; $inserted_rows = $db->insert($query); if ($inserted_rows) { echo '<div class="alert alert-success bg-success text-light border-0 alert-dismissible fade show" role="alert"> The Article has been added! </div>'; }else { echo '<div class="alert alert-danger bg-danger text-light border-0 alert-dismissible fade show" role="alert"> An error occurred while adding the Article to the system! </div>'; } }else{ print_r($errors); } ?> <form class="forms-sample" action="addarticle.php" method="POST" enctype="multipart/form-data"> <div class="form-group"> <label for="exampleInputname">Article name</label> <input type="text" name="articlename" class="form-control" id="exampleInputname" placeholder="T-Shirts"> </div> <div class="form-group"> <label for="exampleInputnumber">Article Number</label> <input type="text" name="number" class="form-control" id="exampleInputnumber" placeholder="401"> </div> <div class="form-group"> <label for="exampleInputproduct">Product</label> <input type="text" name="product" class="form-control" id="exampleInputproduct" placeholder="Base Form"> </div> <div class="form-group"> <label for="exampleInputproduct">Price</label> <input type="text" name="price" class="form-control" id="exampleInputproduct" placeholder="35.90"> </div> <?php $query="SELECT subcatid, subcatname FROM lab_tblsubcategories WHERE 1"; $category=$db->select($query); ?> <div class="form-group"> <label for="inputCatname" class="form-label">Categoryname</label> <select class="form-select" name="subcatname" id="inputCatname"> <option value="0"> Select a category</option> <?php if($category){ while($result=$category->fetch_assoc()){ ?> <option value="<?php echo $result["subcatid"];?>"><?php echo $result["subcatname"];?></option> <?php }?> <?php }else{ echo "Failure within the system"; }?> </select> </div> <div> <label for="inputNumber" class="form-label">Image/Clip</label> <div> <input type="file" name="uploadFile" class="form-control"> </div> </div> <div class="card"> <div class="card-header"><h3>Description</h3></div> <div class="card-body"> <textarea name="description" id="summernote"></textarea> </div> </div> <button class="btn btn-success" type="submit" name="submit">Add Article</button> </form> </div> </div><!-- Ende von Card --> </div> <div class="col-lg-1 col-md-1"></div> </div><!-- Ende von Row --> </section> </main> <?php include("footer.php"); ?>
Das Formular, um den Artikel-Daten zu listen, zu ändern und zu löschen. (admin/articleslist.php)
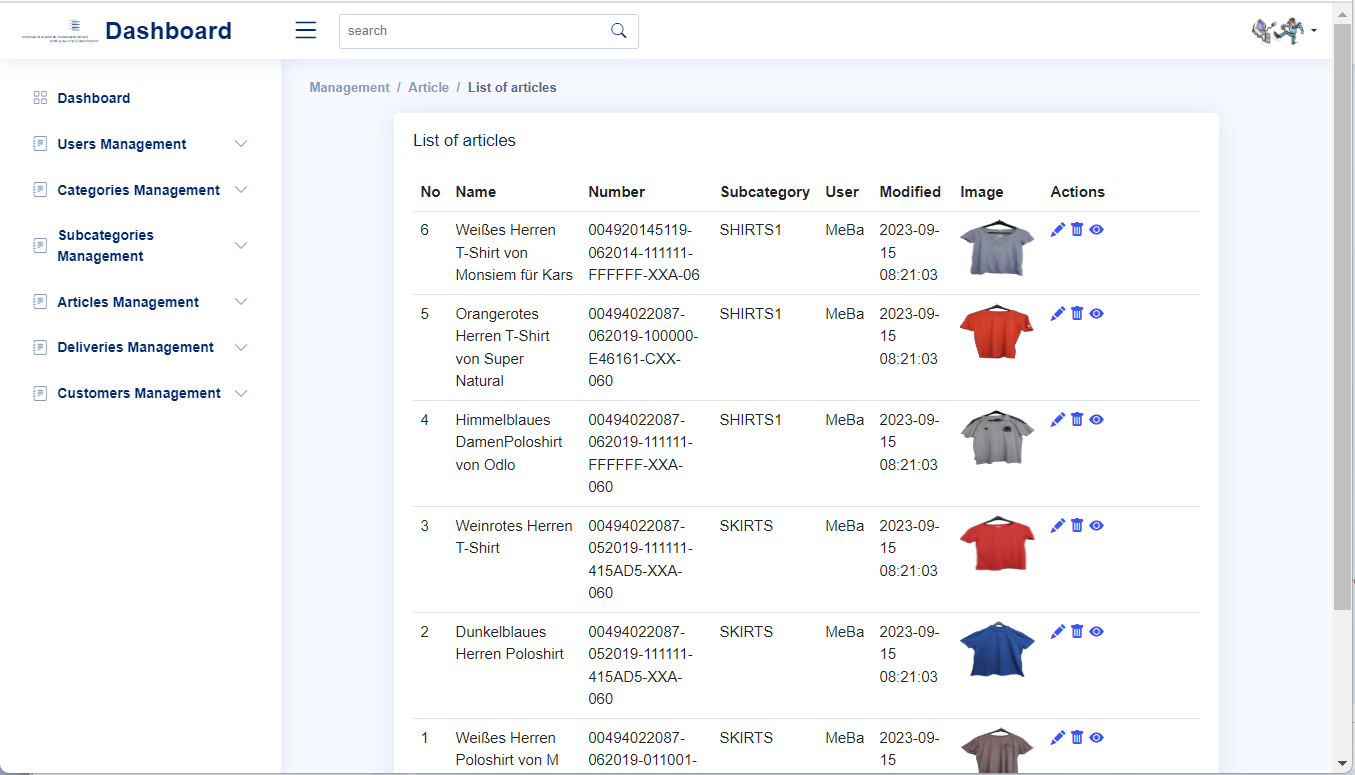
Formular für die Liste der Artikel-Daten
Der Inhalt der Datei (admin/articleslist.php)<?php include("header.php"); include("nav.php"); include ("inc/approveandarticles.php"); $uploadDirectory = __DIR__."/"; ?> <main id="main" class="main"> <div class="pagetitle"> <nav> <ol class="breadcrumb"> <li class="breadcrumb-item"><a href="index.php">Management</a></li> <li class="breadcrumb-item">Article</li> <li class="breadcrumb-item active">List of articles</li> </ol> </nav> </div><!-- End Page Title --> <section class="section"> <div class="row"> <div class="col-lg-1 col-md-1"></div> <div class="col-lg-10 col-md-10"> <div class="card"> <div class="card-body"> <h5 class="card-title">List of articles</h5> <table class="table datatable"> <thead> <tr> <th scope="col">No</th> <th scope="col">Name</th> <th scope="col">Number</th> <th scope="col">Subcategory</th> <th scope="col">User</th> <th scope="col">Modified</th> <th scope="col">Image</th> <th scope="col" width="20%">Actions</th> </tr> </thead> <tbody> <?php $editor=Session::get("role"); $userid=Session::get("id"); $query="SELECT lab_tblarticles.articleid, lab_tblarticles.articlename, lab_tblarticles.number, lab_tblarticles.userid, lab_tblarticles.modified, lab_tblarticles.pic, lab_tblarticles.type, lab_tblarticles.approved, lab_tblusers.name, lab_tblsubcategories.subcatname, lab_tblsubcategories.subcatid FROM lab_tblusers JOIN lab_tblarticles JOIN lab_tblsubcategories WHERE lab_tblusers.id= lab_tblarticles.userid AND lab_tblsubcategories.subcatid=lab_tblarticles.subcatid ORDER BY lab_tblarticles.articleid DESC"; $article=$db->select($query); if($article){ while($result=$article->fetch_assoc()){ ?> <tr> <td scope="row"><?php echo $result["articleid"]; ?></td> <td><?php echo $result["articlename"]; ?></td> <td><?php echo $result["number"]; ?></td> <td class="center"><?php echo $result["subcatname"]; ?> </td> <td class="center"><?php echo $result["name"]; ?> </td> <td class="center"><?php echo $result["modified"]; ?> </td> <?php if ($result["type"] == "image") { echo "<td><img src='".$result["pic"]."' style='text-align:center;height:60px; width:80px;'></td>"; } else if ($result["type"] == "video") { echo "<td><video src='".$result["pic"]."' style='text-align:center;height:60px; width:80px;' controls/></td>"; } ?> <td> <div class="icon"> <a href="editarticles.php?editarticleid=<?php echo $result["articleid"]; ?>" data-bs-toggle="tooltip" data-bs-placement="bottom" title="Edit"> <i class="bi bi-pencil-fill"></i> </a> <?php $editoraccess= Session::get("role"); $articleid2=$result["articleid"]; if ($editoraccess=="admin" or $editoraccess=="editor"){ ?> <a href="javascript: delete_articleid(<?php echo $articleid2;?>)" data-bs-toggle="tooltip" data-bs-placement="bottom" title="Delete"> <i class="bi bi-trash-fill"></i> </a> <?php if ($result["approved"]=="yes"){ echo '<a href="articleslist.php?rejectarticleid='.$articleid2. '" data-bs-toggle="tooltip" data-bs-placement="bottom" title="Approved"> <i class="bi bi-eye-fill"></i> </a>' ; }else{ echo '<a href="articleslist.php?approvearticleid='.$articleid2.'" data-bs-toggle="tooltip" data-bs-placement="bottom" title="Rejected"> <i class="bi bi-eye-slash"></i> </a>'; } } ?> </div> </td> </tr> <?php } }else{ echo "There are no article to display"; } ?> </tbody> </table> </div> </div><!-- End Card --> </div><!-- End col-10 --> <div class="col-lg-1 col-md-1"></div> </div><!-- End row--> </section> </main> <script type="text/javascript"> function delete_articleid(delarticleid) { if (confirm('Delete this article?' )) { window.location.href = 'articleslist.php?delarticleid=' + delarticleid; } } </script> <?php include("footer.php"); ?>
Das Formular, um den Artikel-Daten zu ändern. (admin/editarticles.php)
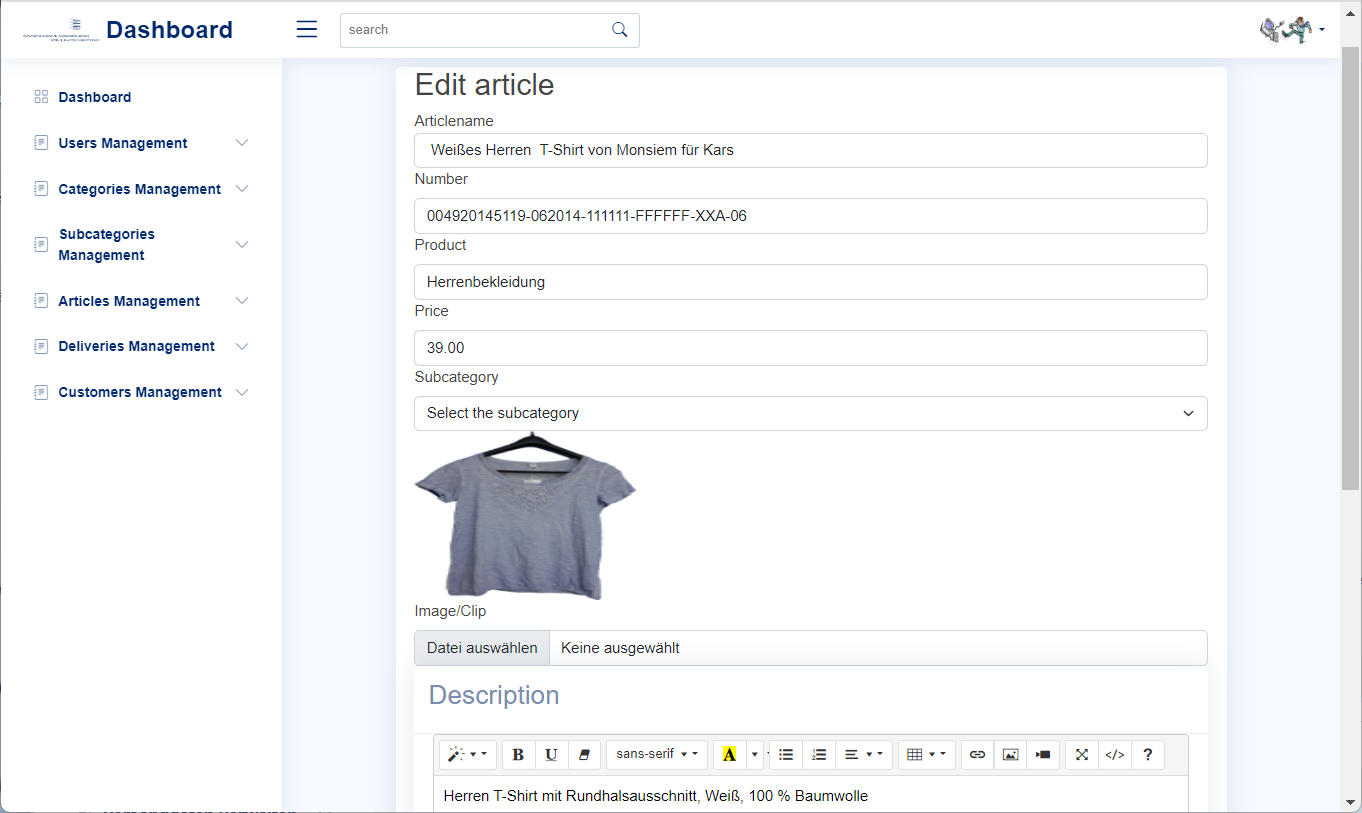
Artikel-Formular für das Ändern der Artikel-Daten
Der Inhalt der Datei (admin/editarticles.php)<?php include("header.php"); include("nav.php"); $uploadDirectory = __DIR__."/"; if(isset($_POST["articleid"])){ $articleid = $_POST["articleid"]; }else if(!isset($_GET["editarticleid"]) || $_GET["editarticleid"]==NULL){ echo '<script> window.location="articleslist.php"</script>'; }else{ $articleid = $_GET["editarticleid"]; } ?> <main id="main" class="main"> <div class="pagearticlename"> <nav> <ol class="breadcrumb"> <li class="breadcrumb-item"><a href="index.php">Management</a></li> <li class="breadcrumb-item">Article</li> <li class="breadcrumb-item active">Edit article</li> </ol> </nav> </div><!-- End Page Title --> <section class="section"> <div class="row"> <div class="col-lg-1 col-md-1"></div> <div class="col-lg-10 col-md-10"> <div class="card"> <div class="card-body"> <h2>Edit article </h2> <?php if ($_SERVER["REQUEST_METHOD"] == "POST" ) { $articlename = mysqli_real_escape_string($db->link, $_POST["articlename"]); $number = mysqli_real_escape_string($db->link, $_POST["number"]); $product = mysqli_real_escape_string($db->link, $_POST["product"]); $price = mysqli_real_escape_string($db->link, $_POST["price"]); $subcatid = mysqli_real_escape_string($db->link, $_POST["subcatname"]); $description = mysqli_real_escape_string($db->link, $_POST["description"]); $userid=Session::get("id"); if($subcatid<1){ $subcatid=$_SESSION["subcatid"]; } $errors= array(); $file_name = $_FILES["uploadFile"]["name"]; $file_size = $_FILES["uploadFile"]["size"]; $file_tmp = $_FILES["uploadFile"]["tmp_name"]; $file_type = $_FILES["uploadFile"]["type"]; $file_ext=strtolower(end(explode(".",$_FILES["uploadFile"]["name"]))); $unique_pic = substr(md5(time()), 0, 10).".".$file_ext; $uploaded_pic = "uploads/articles/".$unique_pic; if(!empty($file_name)){ $file_name = mysqli_real_escape_string($db->link, $file_name); $type = pathinfo($file_name, PATHINFO_EXTENSION); $fileExists = true; while ($fileExists) { if (!file_exists($uploadDirectory.$uploaded_pic)) { $fileExists = false; } } $picold=$_SESSION["oldpic"]; if (picdelete($picold)==false){ ?> <div id="page-wrapper"> <div class="container-fluid"> <div class="bg-darkred fg-white"> <span class="label label-danger">Image file not found!</span> </div> </div> </div> <?php } move_uploaded_file($file_tmp,$uploadDirectory.$uploaded_pic); $mediaType = "something"; switch ($type) { case "mp4": case "mkv": case "mov": case "ogg": case "webm": $mediaType = "video"; break; case "jpg": case "jpeg": case "gif": case "png": default: $mediaType = "image"; break; } $query = "UPDATE lab_tblarticles SET articlename='$articlename', number='$number', product='$product', userid='$userid', subcatid='$subcatid', description='$description', pic='$uploaded_pic', type='$mediaType', price='$price' WHERE articleid='$articleid'"; $updated_rows = $db->update1($query); if ($updated_rows) { echo '<div class="alert alert-success bg-success text-light border-0 alert-dismissible fade show" role="alert"> The article has been updated! </div>'; }else { echo '<div class="alert alert-danger bg-danger text-light border-0 alert-dismissible fade show" role="alert"> The article has not been updated! </div>'; } }else{ $query = "UPDATE lab_tblarticles SET articlename='$articlename', number='$number', product='$product', userid='$userid', subcatid='$subcatid', description='$description', price='$price' WHERE articleid='$articleid'"; $updated_rows = $db->update1($query); if ($updated_rows) { echo '<div class="alert alert-success bg-success text-light border-0 alert-dismissible fade show" role="alert"> The article has been updated! </div>'; }else{ echo '<div class="alert alert-danger bg-danger text-light border-0 alert-dismissible fade show" role="alert"> The article has not been updated! </div>'; } } } ?> <?php $query="SELECT lab_tblarticles.articlename, lab_tblarticles.number, lab_tblarticles.product, lab_tblarticles.description, lab_tblarticles.pic, lab_tblarticles.type, lab_tblarticles.price, lab_tblarticles.subcatid, lab_tblarticles.userid FROM lab_tblarticles JOIN lab_tblsubcategories WHERE lab_tblsubcategories.subcatid=lab_tblarticles.subcatid AND lab_tblarticles.articleid= '$articleid'"; $article=$db->select($query); if($article){ while($result_article=$article->fetch_assoc()){ $_SESSION['subcatid']=$result_article['subcatid']; $_SESSION["oldpic"]= $result_article['pic']; $oldpic=$_SESSION["oldpic"]; ?> <form class="forms-sample" action="editarticles.php" method="POST" enctype="multipart/form-data"> <input type='hidden' name="articleid" value='<?php echo $articleid ?>' /> <div class="form-group"> <label for="exampleInputarticlename" class="form-label2">Articlename</label> <input type="text" name="articlename" class="form-control" id="exampleInputarticlename" value="<?php echo $result_article['articlename'];?>"> </div> <div class="form-group"> <label for="exampleInputnumber" class="form-label">Number</label> <input type="text" name="number" class="form-control" id="exampleInputnumber" value="<?php echo $result_article['number'];?>"> </div> <div class="form-group"> <label for="exampleInputproduct" class="form-label">Product</label> <input type="text" name="product" class="form-control" id="exampleInputproduct" value="<?php echo $result_article['product'];?>"> </div> <div class="form-group"> <label for="exampleInputPrice" class="form-label">Price</label> <input type="text" name="price" class="form-control" id="exampleInputPrice" value="<?php echo $result_article['price'];?>"> </div> <?php $query="SELECT subcatid, subcatname FROM lab_tblsubcategories WHERE 1"; $subcategory=$db->select($query); ?> <div class="form-group"> <label for="" class="form-label">Subcategory</label> <select class="form-select" name="subcatname"> <?php echo "<option value='0'> Select the subcategory</option>"; if($subcategory){ while($result=$subcategory->fetch_assoc()){ echo "<option value='".$result['subcatid']."'>".$result['subcatname']."</option>"; } }else{ echo "Failure within the system"; } ?> </select> </div> <div> <img src='<?php echo $result_article['pic']; ?>' width='240px' height='180px'><br/> <label for="inputNumber" class="form-label">Image/Clip</label> <div> <input type="file" name="uploadFile" class="form-control"> </div> </div> <div class="card"> <div class="card-header"><h3>Description </h3></div> <div class="card-body"> <textarea name="description" id="summernote" dir="rtl"> <?php echo $result_article['description']; ?></textarea> </div> </div> <button class="btn btn-success" type="submit" name="submit">Update article </button> </form> <?php } }else{ echo "Failure within the system"; } ?> </div> </div><!-- Ende von Card --> </div> <div class="col-lg-1 col-md-1"></div> </div><!-- Ende von Row --> </section> </main> <?php include("footer.php"); ?> <?php function picdelete($pic) { if (file_exists($pic)) { if( @unlink($pic) !== true ) throw new Exception('Could not delete file: ' . $pic . ' Please close all applications that are using it.'); } return true; } ?>
Der Inhalt der Datei (admin/inc/approveandarticles.php)
 <?php if(!isset($_GET["delarticleid"]) || $_GET["delarticleid"]==NULL){ }else{ $id = $_GET["delarticleid"]; $query="DELETE FROM lab_tblarticles WHERE articleid='$id'"; $article=$db->delete($query); echo $article; } if(!isset($_GET["approvearticleid"]) || $_GET["approvearticleid"]==NULL){ } else{ $id = $_GET["approvearticleid"]; $query="UPDATE lab_tblarticles SET approved='yes' WHERE articleid='$id'"; $approved=$db->update($query); if($approved){ echo "<span style='color:#fff;'>Article message approvedد </span>"; }else{ echo "<span style='color:#fff;'>Article message not approved</span>"; } } if(!isset($_GET["rejectarticleid"]) || $_GET["rejectarticleid"]==NULL){ } else{ $id = $_GET["rejectarticleid"]; $query="UPDATE lab_tblarticles SET approved='no' WHERE articleid='$id'"; $approved=$db->update($query); if($approved){ echo "<span style='color:#fff;'>Article message rejected. </span>"; }else{ echo "<span style='color:#fff;'>Article message not rejected. </span>"; } } ?>
Das Formular, um den Kunden-Daten in die Datenbank hinzuzufügen (admin/addcustomer.php)
Hier haben alle Benutzer Zugriff. Sie können neue Datensätze in alle Tabellen u.a (lab_tbladdcustomers) hinzufügen.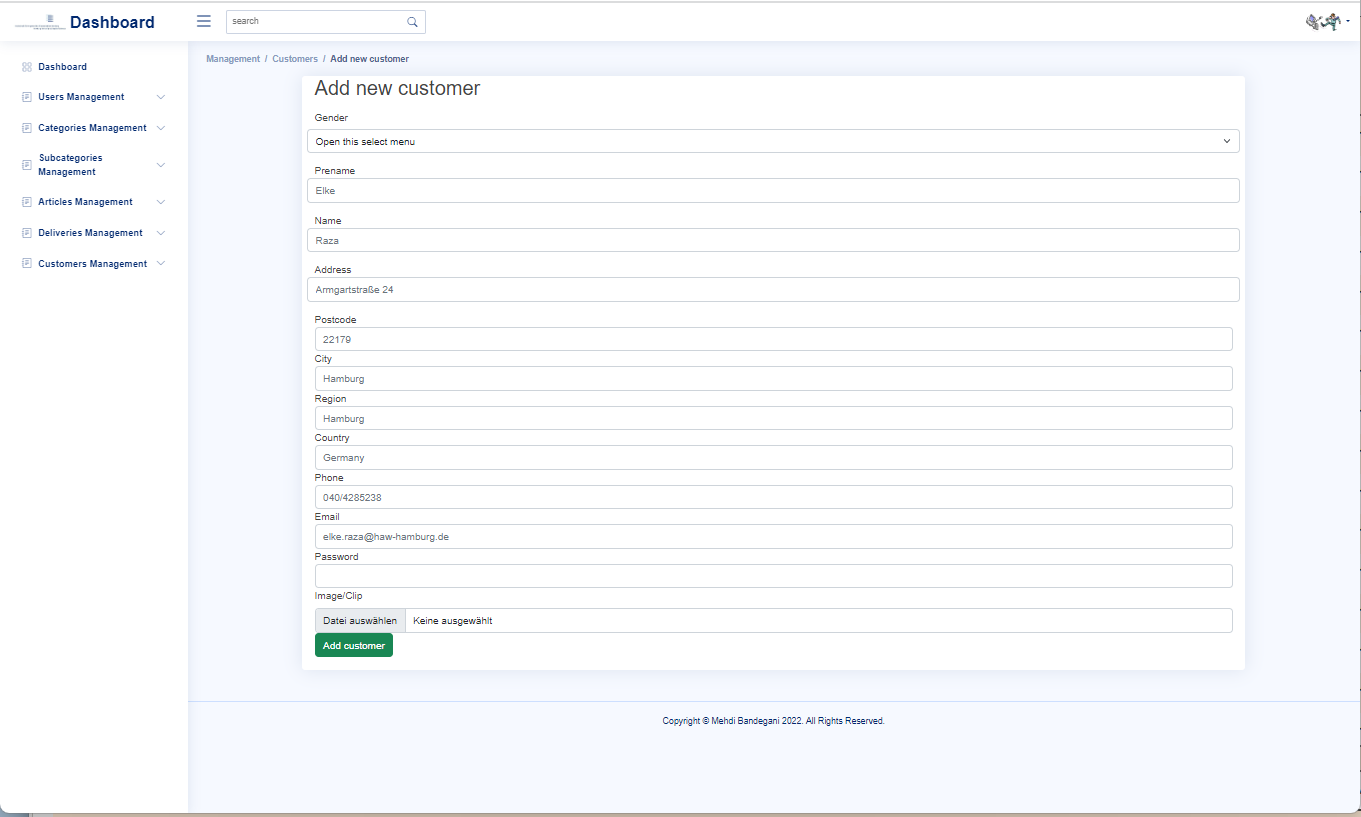
Kunden-Formular für einen neuen Datensatz
Der Inhalt der Datei (admin/addcustomer.php)<?php include("header.php"); include("nav.php"); $uploadDirectory = __DIR__."/"; ?> <main id="main" class="main"> <div class="pagename"> <nav> <ol class="breadcrumb"> <li class="breadcrumb-item"><a href="index.php">Management</a></li> <li class="breadcrumb-item">Customers</li> <li class="breadcrumb-item active">Add new customer</li> </ol> </nav> </div><!-- End Page Title --> <section class="section"> <div class="row"> <div class="col-lg-1 col-md-1"></div> <div class="col-lg-10 col-md-10"> <div class="card"> <div class="card-body"> <h2>Add new customer</h2> <?php if(isset($_FILES["uploadFile"])){ $errors= array(); $file_name = $_FILES["uploadFile"]["name"]; $file_size = $_FILES["uploadFile"]["size"]; $file_tmp = $_FILES["uploadFile"]["tmp_name"]; $file_type = $_FILES["uploadFile"]["type"]; $file_ext=strtolower(end(explode(".",$_FILES["uploadFile"]["name"]))); $unique_pic = substr(md5(time()), 0, 10).".".$file_ext; $uploaded_pic = "uploads/customers/".$unique_pic; if(!empty($file_name)){ if(empty($errors)==true) { $file_name = mysqli_real_escape_string($db->link, $file_name); $type = pathinfo($file_name, PATHINFO_EXTENSION); $fileExists = true; while ($fileExists) { if (!file_exists($uploadDirectory.$uploaded_pic)) { $fileExists = false; } } move_uploaded_file($file_tmp,$uploadDirectory.$uploaded_pic); $mediaType = "something"; switch ($type) { case "mp4": case "mkv": case "mov": case "ogg": case "webm": $mediaType = "video"; break; case "jpg": case "jpeg": case "gif": case "png": default: $mediaType = "image"; break; } } }else { $uploaded_pic = "uploads/customers/shop_logo.gif"; $mediaType = "pic"; } $userid=Session::get("id"); $prename= mysqli_real_escape_string($db->link, $_POST["prename"]); $customername = mysqli_real_escape_string($db->link, $_POST["customername"]); $gender = mysqli_real_escape_string($db->link, $_POST["gender"]); $address = mysqli_real_escape_string($db->link, $_POST["address"]); $city = mysqli_real_escape_string($db->link, $_POST["city"]); $region = mysqli_real_escape_string($db->link, $_POST["region"]); $country = mysqli_real_escape_string($db->link, $_POST["country"]); $postcode = mysqli_real_escape_string($db->link, $_POST["postcode"]); $phone = mysqli_real_escape_string($db->link, $_POST["phone"]); $email = mysqli_real_escape_string($db->link, $_POST["email"]); $password = MD5(mysqli_real_escape_string($db->link, $_POST["password"])); $query = "INSERT INTO lab_tblcustomers (prename, customername, gender, address, city, region, country, postcode, phone, email, password,pic, type, modified, userid) VALUES('$prename', '$customername', '$gender', '$address', '$city', '$region', '$country', '$postcode', '$phone', '$email', '$password', '$uploaded_pic', '$mediaType', NOW() , '$userid')"; $inserted_rows = $db->insert($query); if ($inserted_rows) { echo '<div class="alert alert-success bg-success text-light border-0 alert-dismissible fade show" role="alert"> The Customerhas been added! </div>'; }else { echo '<div class="alert alert-danger bg-danger text-light border-0 alert-dismissible fade show" role="alert"> An error occurred while adding the Customer to the system! </div>'; } }else{ print_r($errors); } ?> <form class="forms-sample" action="addcustomer.php" method="POST" enctype="multipart/form-data"> <div class="form-group row mb-3"> <label class="col-sm-2 col-form-label">Gender</label> <select class="form-select" name="gender" aria-label="Floating label select example"> <option selected>Open this select menu</option> <option value="girl">Girl</option> <option value="boy">Boy</option> <option value="woman">Woman</option> <option value="gentleman">Gentleman</option> </select> </div> <div class="form-group row mb-3"> <label for="exampleInputprename">Prename</label> <input type="text" name="prename" class="form-control" id="exampleInputprename" placeholder="Elke"> </div> <div class="form-group row mb-3"> <label for="exampleInputname">Name</label> <input type="text" name="customername" class="form-control" id="exampleInputname" placeholder="Raza"> </div> <div class="form-group row mb-3"> <label for="exampleInputaddress">Address</label> <input type="text" name="address" class="form-control" id="exampleInputaddress" placeholder="Armgartstraße 24"> </div> <div class="form-group"> <label for="exampleInputpostcode">Postcode</label> <input type="text" name="postcode" class="form-control" id="exampleInputpostcode" placeholder="22179"> </div> <div class="form-group"> <label for="exampleInputcity">City</label> <input type="text" name="city" class="form-control" id="exampleInputcity" placeholder="Hamburg"> </div> <div class="form-group"> <label for="exampleInputregion">Region</label> <input type="text" name="region" class="form-control" id="exampleInputregion" placeholder="Hamburg"> </div> <div class="form-group"> <label for="exampleInputcountry">Country</label> <input type="text" name="country" class="form-control" id="exampleInputcountry" placeholder="Germany"> </div> <div class="form-group"> <label for="exampleInputphone">Phone</label> <input type="text" name="phone" class="form-control" id="exampleInputphone" placeholder="040/4285238"> </div> <div class="form-group"> <label for="exampleInputemail">Email</label> <input type="email" name="email" class="form-control" id="exampleInputemail" placeholder="elke.raza@haw-hamburg.de"> </div> <div class="form-group"> <label for="exampleInputpassword">Password</label> <input type="password" name="password" class="form-control" id="exampleInputpassword"> </div> <div> <label class="form-label">Image/Clip</label> <div> <input type="file" name="uploadFile" class="form-control"> </div> </div> <button class="btn btn-success" type="submit" name="submit">Add customer</button> </form> </div> </div><!-- Ende von Card --> </div> <div class="col-lg-1 col-md-1"></div> </div><!-- Ende von Row --> </section> </main> <?php include("footer.php"); ?>
Das Formular, um den Kunden-Daten zu listen, zu ändern und zu löschen. (admin/customerslist.php)

Formular für die Liste der Kunden-Daten
Der Inhalt der Datei (admin/customerslist.php)<?php include("header.php"); include("nav.php"); include ("inc/approveandcustomers.php"); $uploadDirectory = __DIR__."/"; ?> <main id="main" class="main"> <div class="pagetitle"> <nav> <ol class="breadcrumb"> <li class="breadcrumb-item"><a href="index.php">Management</a></li> <li class="breadcrumb-item">Customer</li> <li class="breadcrumb-item active">List of customers</li> </ol> </nav> </div><!-- End Page Title --> <section class="section"> <div class="row"> <div class="col-lg-1 col-md-1"></div> <div class="col-lg-10 col-md-10"> <div class="card"> <div class="card-body"> <h5 class="card-title">List of customers</h5> <table class="table datatable"> <thead> <tr> <th scope="col">No</th> <th scope="col">Preame</th> <th scope="col">Name</th> <th scope="col">Address</th> <th scope="col">User</th> <th scope="col">Modified</th> <th scope="col">Image</th> <th scope="col" width="20%">Actions</th> </tr> </thead> <tbody> <?php $editor=Session::get("role"); $userid=Session::get("id"); $query="SELECT lab_tblcustomers.customerid, lab_tblcustomers.customername, lab_tblcustomers.prename, lab_tblcustomers.userid, lab_tblcustomers.address, lab_tblcustomers.modified, lab_tblcustomers.pic, lab_tblcustomers.type, lab_tblcustomers.approved, lab_tblusers.name FROM lab_tblusers JOIN lab_tblcustomers WHERE lab_tblusers.id= lab_tblcustomers.userid ORDER BY lab_tblcustomers.customerid DESC"; $customer=$db->select($query); if($customer){ while($result=$customer->fetch_assoc()){ ?> <tr> <td scope="row"><?php echo $result["customerid"]; ?></td> <td><?php echo $result["prename"]; ?></td> <td><?php echo $result["customername"]; ?></td> <td><?php echo $result["address"]; ?></td> <td class="center"><?php echo $result["name"]; ?> </td> <td class="center"><?php echo $result["modified"]; ?> </td> <?php if ($result["type"] == "image") { echo "<td><img src='".$result["pic"]."' style='text-align:center;height:60px; width:80px;'></td>"; } else if ($result["type"] == "video") { echo "<td><video src='".$result["pic"]."' style='text-align:center;height:60px; width:80px;' controls/></td>"; } ?> <td> <div class="icon"> <a href="editcustomers.php?editcustomerid=<?php echo $result["customerid"]; ?>" data-bs-toggle="tooltip" data-bs-placement="bottom" title="Edit"> <i class="bi bi-pencil-fill"></i> </a> <?php $editoraccess= Session::get("role"); $customerid2=$result["customerid"]; if ($editoraccess=="admin" or $editoraccess=="editor"){ ?> <a href="javascript: delete_customerid(<?php echo $customerid2;?>)" data-bs-toggle="tooltip" data-bs-placement="bottom" title="Delete"> <i class="bi bi-trash-fill"></i> </a> <?php if ($result["approved"]=="yes"){ echo '<a href="customerslist.php?rejectcustomerid='.$customerid2. '" data-bs-toggle="tooltip" data-bs-placement="bottom" title="Approved"> <i class="bi bi-eye-fill"></i> </a>' ; }else{ echo '<a href="customerslist.php?approvecustomerid='.$customerid2.'" data-bs-toggle="tooltip" data-bs-placement="bottom" title="Rejected"> <i class="bi bi-eye-slash"></i> </a>'; } } ?> </div> </td> </tr> <?php } }else{ echo "There are no customer to display"; } ?> </tbody> </table> </div> </div><!-- End Card --> </div><!-- End col-10 --> <div class="col-lg-1 col-md-1"></div> </div><!-- End row--> </section> </main> <script type="text/javascript"> function delete_customerid(delcustomerid) { if (confirm('Delete this customer?' )) { window.location.href = 'customerslist.php?delcustomerid=' + delcustomerid; } } </script> <?php include("footer.php"); ?>
Das Formular, um den Kunden-Daten zu ändern. (admin/editcustomers.php)
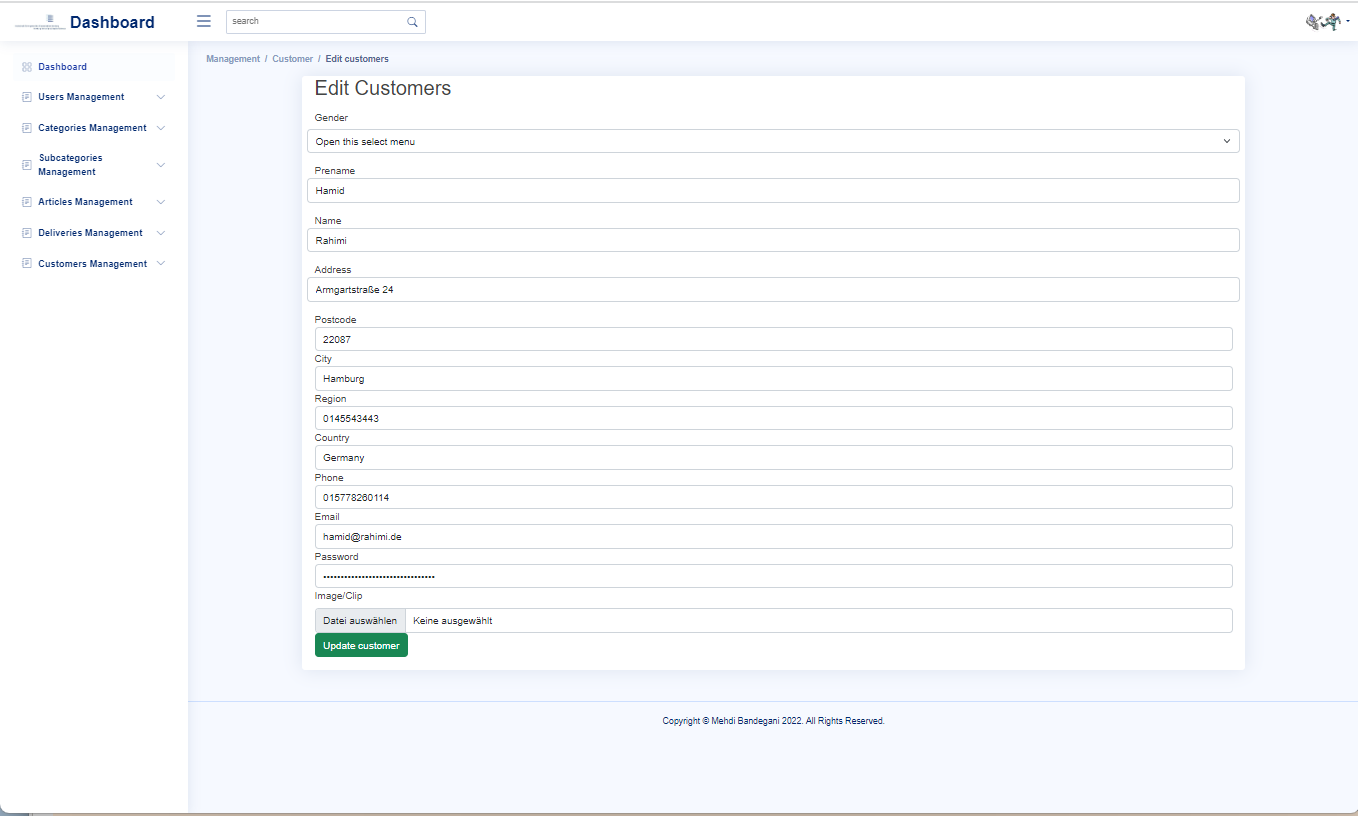
Kunden-Formular für das Ändern der Kunden-Daten
Der Inhalt der Datei (admin/editcustomers.php)<?php include("header.php"); include("nav.php"); $uploadDirectory = __DIR__."/"; if(isset($_POST["customerid"])){ $customerid = $_POST["customerid"]; }else if(!isset($_GET["editcustomerid"]) || $_GET["editcustomerid"]==NULL){ echo '<script> window.location="postlist.php"</script>'; }else{ $customerid = $_GET["editcustomerid"]; } ?> <main id="main" class="main"> <div class="pagearticlename"> <nav> <ol class="breadcrumb"> <li class="breadcrumb-item"><a href="index.php">Management</a></li> <li class="breadcrumb-item">Customer</li> <li class="breadcrumb-item active">Edit customers</li> </ol> </nav> </div><!-- End Page Title --> <section class="section"> <div class="row"> <div class="col-lg-1 col-md-1"></div> <div class="col-lg-10 col-md-10"> <div class="card"> <div class="card-body"> <h2>Edit Customers </h2> <?php if ($_SERVER["REQUEST_METHOD"] == "POST" ) { $prename= mysqli_real_escape_string($db->link, $_POST["prename"]); $customername = mysqli_real_escape_string($db->link, $_POST["customername"]); $gender = mysqli_real_escape_string($db->link, $_POST["gender"]); $address = mysqli_real_escape_string($db->link, $_POST["address"]); $city = mysqli_real_escape_string($db->link, $_POST["city"]); $region = mysqli_real_escape_string($db->link, $_POST["region"]); $country = mysqli_real_escape_string($db->link, $_POST["country"]); $postcode = mysqli_real_escape_string($db->link, $_POST["postcode"]); $phone = mysqli_real_escape_string($db->link, $_POST["phone"]); $email = mysqli_real_escape_string($db->link, $_POST["email"]); $password = MD5(mysqli_real_escape_string($db->link, $_POST["password"])); if($gender<1){ $gender=$_SESSION["gender"]; } $userid=Session::get("id"); $errors= array(); $file_name = $_FILES["uploadFile"]["name"]; $file_size = $_FILES["uploadFile"]["size"]; $file_tmp = $_FILES["uploadFile"]["tmp_name"]; $file_type = $_FILES["uploadFile"]["type"]; $file_ext=strtolower(end(explode(".",$_FILES["uploadFile"]["name"]))); $unique_pic = substr(md5(time()), 0, 10).".".$file_ext; $uploaded_pic = "uploads/customers/".$unique_pic; if(!empty($file_name)){ $file_name = mysqli_real_escape_string($db->link, $file_name); $type = pathinfo($file_name, PATHINFO_EXTENSION); $fileExists = true; while ($fileExists) { if (!file_exists($uploadDirectory.$uploaded_pic)) { $fileExists = false; } } $picold=$_SESSION["oldpic"]; if (picdelete($picold)==false){ ?> <div id="page-wrapper"> <div class="container-fluid"> <div class="bg-darkred fg-white"> <span class="label label-danger">Image file not found!</span> </div> </div> </div> <?php } move_uploaded_file($file_tmp,$uploadDirectory.$uploaded_pic); $mediaType = "something"; switch ($type) { case "mp4": case "mkv": case "mov": case "ogg": case "webm": $mediaType = "video"; break; case "jpg": case "jpeg": case "gif": case "png": default: $mediaType = "image"; break; } $query = "UPDATE lab_tblcustomers SET prename='$prename', customername='$customername', gender='$gender', userid='$userid', address='$address', city='$city', pic='$uploaded_pic', type='$mediaType', phone='$phone', password='$password', region='$region', country='$country', country='$country', postcode='$postcode', email='$email' WHERE customerid='$customerid'"; $updated_rows = $db->update1($query); if ($updated_rows) { echo '<div class="alert alert-success bg-success text-light border-0 alert-dismissible fade show" role="alert"> The article has been updated! </div>'; }else { echo '<div class="alert alert-danger bg-danger text-light border-0 alert-dismissible fade show" role="alert"> The article has not been updated! </div>'; } }else{ $query = "UPDATE lab_tblcustomers SET prename='$prename', customername='$customername', gender='$gender', userid='$userid', address='$address', city='$city', phone='$phone', password='$password', region='$region', country='$country', country='$country', postcode='$postcode', email='$email' WHERE customerid='$customerid'"; $updated_rows = $db->update1($query); if ($updated_rows) { echo '<div class="alert alert-success bg-success text-light border-0 alert-dismissible fade show" role="alert"> The article has been updated! </div>'; }else{ echo '<div class="alert alert-danger bg-danger text-light border-0 alert-dismissible fade show" role="alert"> The article has not been updated! </div>'; } } } ?> <?php $query="SELECT * FROM lab_tblcustomers WHERE customerid= '$customerid'"; $customer=$db->select($query); if($customer){ while($result=$customer->fetch_assoc()){ $_SESSION["oldpic"]= $result['pic']; $_SESSION["gender"]= $result['gender']; $oldpic=$_SESSION["oldpic"]; ?> <form class="forms-sample" action="editcustomers.php" method="POST" enctype="multipart/form-data"> <input type='hidden' name="customerid" value='<?php echo $customerid ?>' /> <div class="form-group row mb-3"> <label class="col-sm-2 col-form-label">Gender</label> <select class="form-select" name="gender" aria-label="Floating label select example"> <option selected>Open this select menu</option> <option value="girl">Girl</option> <option value="boy">Boy</option> <option value="woman">Woman</option> <option value="gentleman">Gentleman</option> </select> </div> <div class="form-group row mb-3"> <label for="exampleInputprename">Prename</label> <input type="text" name="prename" class="form-control" id="exampleInputprename" value="<?php echo $result['prename'];?>"> </div> <div class="form-group row mb-3"> <label for="exampleInputname">Name</label> <input type="text" name="customername" class="form-control" id="exampleInputname" value="<?php echo $result['customername'];?>"> </div> <div class="form-group row mb-3"> <label for="exampleInputaddress">Address</label> <input type="text" name="address" class="form-control" id="exampleInputaddress" value="<?php echo $result['address'];?>"> </div> <div class="form-group"> <label for="exampleInputpostcode">Postcode</label> <input type="text" name="postcode" class="form-control" id="exampleInputpostcode" value="<?php echo $result['postcode'];?>"> </div> <div class="form-group"> <label for="exampleInputcity">City</label> <input type="text" name="city" class="form-control" id="exampleInputcity" value="<?php echo $result['city'];?>"> </div> <div class="form-group"> <label for="exampleInputregion">Region</label> <input type="text" name="region" class="form-control" id="exampleInputregion" value="<?php echo $result['region'];?>"> </div> <div class="form-group"> <label for="exampleInputcountry">Country</label> <input type="text" name="country" class="form-control" id="exampleInputcountry" value="<?php echo $result['country'];?>"> </div> <div class="form-group"> <label for="exampleInputphone">Phone</label> <input type="text" name="phone" class="form-control" id="exampleInputphone" value="<?php echo $result['phone'];?>"> </div> <div class="form-group"> <label for="exampleInputemail">Email</label> <input type="email" name="email" class="form-control" id="exampleInputemail" value="<?php echo $result['email'];?>"> </div> <div class="form-group"> <label for="exampleInputpassword">Password</label> <input type="password" name="password" class="form-control" id="exampleInputpassword" value="<?php echo $result['password'];?>"> </div> <div> <label class="form-label">Image/Clip</label> <div> <input type="file" name="uploadFile" class="form-control"> </div> </div> <button class="btn btn-success" type="submit" name="submit">Update customer </button> </form> <?php } }else{ echo "Failure within the system"; } ?> </div> </div><!-- Ende von Card --> </div> <div class="col-lg-1 col-md-1"></div> </div><!-- Ende von Row --> </section> </main> <?php include("footer.php"); ?> <?php function picdelete($pic) { if (file_exists($pic)) { if( @unlink($pic) !== true ) throw new Exception('Could not delete file: ' . $pic . ' Please close all applications that are using it.'); } return true; } ?>
Der Inhalt der Datei (admin/inc/approveandcustomers.php)
 <?php if(!isset($_GET["delcustomerid"]) || $_GET["delcustomerid"]==NULL){ }else{ $id = $_GET["delcustomerid"]; $query="DELETE FROM lab_tblcustomers WHERE customerid='$id'"; $customer=$db->delete($query); echo $customer; } if(!isset($_GET["approvecustomerid"]) || $_GET["approvecustomerid"]==NULL){ } else{ $id = $_GET["approvecustomerid"]; $query="UPDATE lab_tblcustomers SET approved='yes' WHERE customerid='$id'"; $approved=$db->update($query); if($approved){ echo "<span style='color:#fff;'>Customer message approvedد </span>"; }else{ echo "<span style='color:#fff;'>Customer message not approved</span>"; } } if(!isset($_GET["rejectcustomerid"]) || $_GET["rejectcustomerid"]==NULL){ } else{ $id = $_GET["rejectcustomerid"]; $query="UPDATE lab_tblcustomers SET approved='no' WHERE customerid='$id'"; $approved=$db->update($query); if($approved){ echo "<span style='color:#fff;'>Customer message rejected. </span>"; }else{ echo "<span style='color:#fff;'>Customer message not rejected. </span>"; } } ?>
Das Formular, um den Lieferanten-Daten in die Datenbank hinzuzufügen (admin/adddelivery.php)
Hier haben alle Benutzer Zugriff. Sie können neue Datensätze in alle Tabellen u.a (lab_tbladddeliveries) hinzufügen.
Lieferanten-Formular für einen neuen Datensatz
Der Inhalt der Datei (admin/adddelivery.php)<?php include("header.php"); include("nav.php"); $uploadDirectory = __DIR__."/"; ?> <main id="main" class="main"> <div class="pagename"> <nav> <ol class="breadcrumb"> <li class="breadcrumb-item"><a href="index.php">Management</a></li> <li class="breadcrumb-item">Deliveries</li> <li class="breadcrumb-item active">Add new Delivery</li> </ol> </nav> </div><!-- End Page Title --> <section class="section"> <div class="row"> <div class="col-lg-1 col-md-1"></div> <div class="col-lg-10 col-md-10"> <div class="card"> <div class="card-body"> <h2>Add new Delivery</h2> <?php if(isset($_FILES["uploadFile"])){ $errors= array(); $file_name = $_FILES["uploadFile"]["name"]; $file_size = $_FILES["uploadFile"]["size"]; $file_tmp = $_FILES["uploadFile"]["tmp_name"]; $file_type = $_FILES["uploadFile"]["type"]; $file_ext=strtolower(end(explode(".",$_FILES["uploadFile"]["name"]))); $unique_pic = substr(md5(time()), 0, 10).".".$file_ext; $uploaded_pic = "uploads/deliveries/".$unique_pic; if(!empty($file_name)){ if(empty($errors)==true) { $file_name = mysqli_real_escape_string($db->link, $file_name); $type = pathinfo($file_name, PATHINFO_EXTENSION); $fileExists = true; while ($fileExists) { if (!file_exists($uploadDirectory.$uploaded_pic)) { $fileExists = false; } } move_uploaded_file($file_tmp,$uploadDirectory.$uploaded_pic); $mediaType = "something"; switch ($type) { case "mp4": case "mkv": case "mov": case "ogg": case "webm": $mediaType = "video"; break; case "jpg": case "jpeg": case "gif": case "png": default: $mediaType = "image"; break; } } }else { $uploaded_pic = "uploads/deliveries/shop_logo.gif"; $mediaType = "pic"; } $userid=Session::get("id"); $prename= mysqli_real_escape_string($db->link, $_POST["prename"]); $customername = mysqli_real_escape_string($db->link, $_POST["deliveryname"]); $gender = mysqli_real_escape_string($db->link, $_POST["gender"]); $address = mysqli_real_escape_string($db->link, $_POST["address"]); $city = mysqli_real_escape_string($db->link, $_POST["city"]); $region = mysqli_real_escape_string($db->link, $_POST["region"]); $country = mysqli_real_escape_string($db->link, $_POST["country"]); $postcode = mysqli_real_escape_string($db->link, $_POST["postcode"]); $phone = mysqli_real_escape_string($db->link, $_POST["phone"]); $email = mysqli_real_escape_string($db->link, $_POST["email"]); $query = "INSERT INTO lab_tbldeliveries (prename, deliveryname, gender, address, city, region, country, postcode, phone, email, pic, type, modified, userid) VALUES('$prename', '$customername', '$gender', '$address', '$city', '$region', '$country', '$postcode', '$phone', '$email', '$uploaded_pic', '$mediaType', NOW() , '$userid')"; $inserted_rows = $db->insert($query); if ($inserted_rows) { echo '<div class="alert alert-success bg-success text-light border-0 alert-dismissible fade show" role="alert"> The Delivery has been added! </div>'; }else { echo '<div class="alert alert-danger bg-danger text-light border-0 alert-dismissible fade show" role="alert"> An error occurred while adding the Delivery to the system! </div>'; } }else{ print_r($errors); } ?> <form class="forms-sample" action="adddelivery.php" method="POST" enctype="multipart/form-data"> <div class="form-group row mb-3"> <label class="col-sm-2 col-form-label">Gender</label> <select class="form-select" name="gender" aria-label="Floating label select example"> <option selected>Open this select menu</option> <option value="girl">Girl</option> <option value="boy">Boy</option> <option value="woman">Woman</option> <option value="gentleman">Gentleman</option> </select> </div> <div class="form-group row mb-3"> <label for="exampleInputprename">Prename</label> <input type="text" name="prename" class="form-control" id="exampleInputprename" placeholder="Elke"> </div> <div class="form-group row mb-3"> <label for="exampleInputname">Name</label> <input type="text" name="deliveryname" class="form-control" id="exampleInputname" placeholder="Raza"> </div> <div class="form-group row mb-3"> <label for="exampleInputaddress">Address</label> <input type="text" name="address" class="form-control" id="exampleInputaddress" placeholder="Armgartstraße 24"> </div> <div class="form-group"> <label for="exampleInputpostcode">Postcode</label> <input type="text" name="postcode" class="form-control" id="exampleInputpostcode" placeholder="22179"> </div> <div class="form-group"> <label for="exampleInputcity">City</label> <input type="text" name="city" class="form-control" id="exampleInputcity" placeholder="Hamburg"> </div> <div class="form-group"> <label for="exampleInputregion">Region</label> <input type="text" name="region" class="form-control" id="exampleInputregion" placeholder="Hamburg"> </div> <div class="form-group"> <label for="exampleInputcountry">Country</label> <input type="text" name="country" class="form-control" id="exampleInputcountry" placeholder="Germany"> </div> <div class="form-group"> <label for="exampleInputphone">Phone</label> <input type="text" name="phone" class="form-control" id="exampleInputphone" placeholder="040/4285238"> </div> <div class="form-group"> <label for="exampleInputemail">Email</label> <input type="email" name="email" class="form-control" id="exampleInputemail" placeholder="elke.raza@haw-hamburg.de"> </div> <div> <label class="form-label">Image/Clip</label> <div> <input type="file" name="uploadFile" class="form-control"> </div> </div> <button class="btn btn-success" type="submit" name="submit">Add Delivery</button> </form> </div> </div><!-- Ende von Card --> </div> <div class="col-lg-1 col-md-1"></div> </div><!-- Ende von Row --> </section> </main> <?php include("footer.php"); ?>
Das Formular, um den Lieferanten-Daten zu listen, zu ändern und zu löschen. (admin/deliverieslist.php)

Formular für die Liste der Lieferanten-Daten
Der Inhalt der Datei (admin/deliverieslist.php)<?php include("header.php"); include("nav.php"); include ("inc/approveanddeliveries.php"); ?> <main id="main" class="main"> <div class="pagetitle"> <nav> <ol class="breadcrumb"> <li class="breadcrumb-item"><a href="index.php">Management</a></li> <li class="breadcrumb-item">Delivery</li> <li class="breadcrumb-item active">List of deliveries</li> </ol> </nav> </div><!-- End Page Title --> <section class="section"> <div class="row"> <div class="col-lg-1 col-md-1"></div> <div class="col-lg-10 col-md-10"> <div class="card"> <div class="card-body"> <h5 class="card-title">List of deliveries</h5> <table class="table datatable"> <thead> <tr> <th scope="col">No</th> <th scope="col">Preame</th> <th scope="col">Name</th> <th scope="col">Address</th> <th scope="col">User</th> <th scope="col">Modified</th> <th scope="col" width="20%">Actions</th> </tr> </thead> <tbody> <?php $editor=Session::get("role"); $userid=Session::get("id"); $query="SELECT lab_tbldeliveries.deliveryid, lab_tbldeliveries.deliveryname, lab_tbldeliveries.prename, lab_tbldeliveries.userid, lab_tbldeliveries.address, lab_tbldeliveries.modified, lab_tbldeliveries.approved, lab_tblusers.name FROM lab_tblusers JOIN lab_tbldeliveries WHERE lab_tblusers.id= lab_tbldeliveries.userid ORDER BY lab_tbldeliveries.deliveryid DESC"; $customer=$db->select($query); if($customer){ while($result=$customer->fetch_assoc()){ ?> <tr> <td scope="row"><?php echo $result["deliveryid"]; ?></td> <td><?php echo $result["prename"]; ?></td> <td><?php echo $result["deliveryname"]; ?></td> <td><?php echo $result["address"]; ?></td> <td class="center"><?php echo $result["name"]; ?> </td> <td class="center"><?php echo $result["modified"]; ?> </td> <td> <div class="icon"> <a href="editdeliveries.php?editdeliveryid=<?php echo $result["deliveryid"]; ?>" data-bs-toggle="tooltip" data-bs-placement="bottom" title="Edit"> <i class="bi bi-pencil-fill"></i> </a> <?php $editoraccess= Session::get("role"); $deliveryid2=$result["deliveryid"]; if ($editoraccess=="admin" or $editoraccess=="editor"){ ?> <a href="javascript: delete_deliveryid(<?php echo $deliveryid2;?>)" data-bs-toggle="tooltip" data-bs-placement="bottom" title="Delete"> <i class="bi bi-trash-fill"></i> </a> <?php if ($result["approved"]=="yes"){ echo '<a href="deliverieslist.php?rejectdeliveryid='.$deliveryid2. '" data-bs-toggle="tooltip" data-bs-placement="bottom" title="Approved"> <i class="bi bi-eye-fill"></i> </a>' ; }else{ echo '<a href="deliverieslist.php?approvedeliveryid='.$deliveryid2.'" data-bs-toggle="tooltip" data-bs-placement="bottom" title="Rejected"> <i class="bi bi-eye-slash"></i> </a>'; } } ?> </div> </td> </tr> <?php } }else{ echo "There are no Delivery to display"; } ?> </tbody> </table> </div> </div><!-- End Card --> </div><!-- End col-10 --> <div class="col-lg-1 col-md-1"></div> </div><!-- End row--> </section> </main> <script type="text/javascript"> function delete_deliveryid(deldeliveryid) { if (confirm('Delete this delivery?' )) { window.location.href = 'deliverieslist.php?deldeliveryid=' + deldeliveryid; } } </script> <?php include("footer.php"); ?>
Das Formular, um den Lieferanten-Daten zu ändern. (admin/editdeliveries.php)
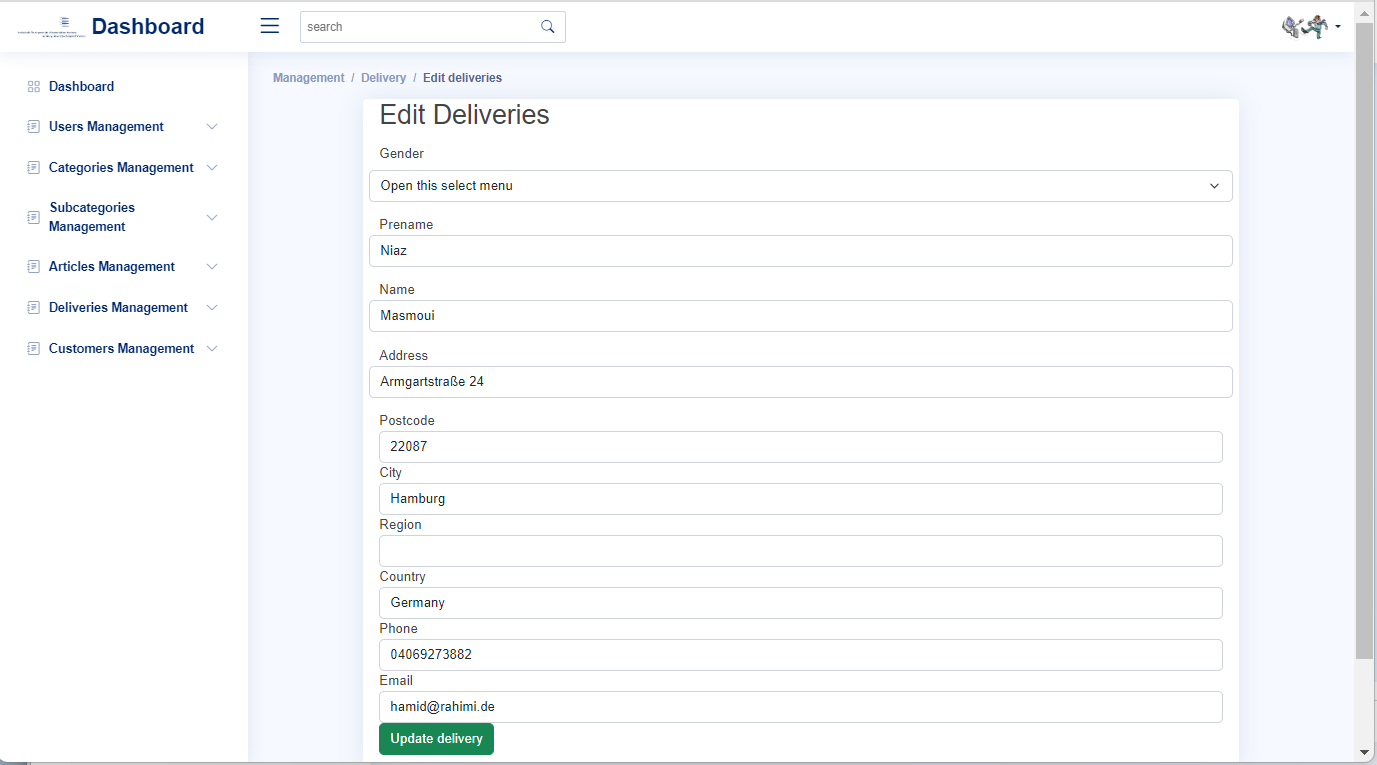
Lieferanten-Formular für das Ändern der Lieferanten-Daten
Der Inhalt der Datei (admin/editdeliveries.php)<?php include("header.php"); include("nav.php"); $uploadDirectory = __DIR__."/"; if(isset($_POST["deliveryid"])){ $deliveryid = $_POST["deliveryid"]; }else if(!isset($_GET["editdeliveryid"]) || $_GET["editdeliveryid"]==NULL){ echo '<script> window.location="postlist.php"</script>'; }else{ $deliveryid = $_GET["editdeliveryid"]; } ?> <main id="main" class="main"> <div class="pagearticlename"> <nav> <ol class="breadcrumb"> <li class="breadcrumb-item"><a href="index.php">Management</a></li> <li class="breadcrumb-item">Customer</li> <li class="breadcrumb-item active">Edit deliveries</li> </ol> </nav> </div><!-- End Page Title --> <section class="section"> <div class="row"> <div class="col-lg-1 col-md-1"></div> <div class="col-lg-10 col-md-10"> <div class="card"> <div class="card-body"> <h2>Edit Customers </h2> <?php if ($_SERVER["REQUEST_METHOD"] == "POST" ) { $prename= mysqli_real_escape_string($db->link, $_POST["prename"]); $deliveryname = mysqli_real_escape_string($db->link, $_POST["deliveryname"]); $gender = mysqli_real_escape_string($db->link, $_POST["gender"]); $address = mysqli_real_escape_string($db->link, $_POST["address"]); $city = mysqli_real_escape_string($db->link, $_POST["city"]); $region = mysqli_real_escape_string($db->link, $_POST["region"]); $country = mysqli_real_escape_string($db->link, $_POST["country"]); $postcode = mysqli_real_escape_string($db->link, $_POST["postcode"]); $phone = mysqli_real_escape_string($db->link, $_POST["phone"]); $email = mysqli_real_escape_string($db->link, $_POST["email"]); if($gender<1){ $gender=$_SESSION["gender"]; } $userid=Session::get("id"); $errors= array(); $file_name = $_FILES["uploadFile"]["name"]; $file_size = $_FILES["uploadFile"]["size"]; $file_tmp = $_FILES["uploadFile"]["tmp_name"]; $file_type = $_FILES["uploadFile"]["type"]; $file_ext=strtolower(end(explode(".",$_FILES["uploadFile"]["name"]))); $unique_pic = substr(md5(time()), 0, 10).".".$file_ext; $uploaded_pic = "uploads/deliveries/".$unique_pic; if(!empty($file_name)){ $file_name = mysqli_real_escape_string($db->link, $file_name); $type = pathinfo($file_name, PATHINFO_EXTENSION); $fileExists = true; while ($fileExists) { if (!file_exists($uploadDirectory.$uploaded_pic)) { $fileExists = false; } } $picold=$_SESSION["oldpic"]; if (picdelete($picold)==false){ ?> <div id="page-wrapper"> <div class="container-fluid"> <div class="bg-darkred fg-white"> <span class="label label-danger">Image file not found!</span> </div> </div> </div> <?php } move_uploaded_file($file_tmp,$uploadDirectory.$uploaded_pic); $mediaType = "something"; switch ($type) { case "mp4": case "mkv": case "mov": case "ogg": case "webm": $mediaType = "video"; break; case "jpg": case "jpeg": case "gif": case "png": default: $mediaType = "image"; break; } $query = "UPDATE lab_tbldeliveries SET prename='$prename', deliveryname='$deliveryname', gender='$gender', userid='$userid', address='$address', city='$city', pic='$uploaded_pic', type='$mediaType', phone='$phone', region='$region', country='$country', country='$country', postcode='$postcode', email='$email' WHERE deliveryid='$deliveryid'"; $updated_rows = $db->update1($query); if ($updated_rows) { echo '<div class="alert alert-success bg-success text-light border-0 alert-dismissible fade show" role="alert"> The article has been updated! </div>'; }else { echo '<div class="alert alert-danger bg-danger text-light border-0 alert-dismissible fade show" role="alert"> The article has not been updated! </div>'; } }else{ $query = "UPDATE lab_tbldeliveries SET prename='$prename', deliveryname='$deliveryname', gender='$gender', userid='$userid', address='$address', city='$city', phone='$phone', region='$region', country='$country', country='$country', postcode='$postcode', email='$email' WHERE deliveryid='$deliveryid'"; $updated_rows = $db->update1($query); if ($updated_rows) { echo '<div class="alert alert-success bg-success text-light border-0 alert-dismissible fade show" role="alert"> The article has been updated! </div>'; }else{ echo '<div class="alert alert-danger bg-danger text-light border-0 alert-dismissible fade show" role="alert"> The article has not been updated! </div>'; } } } ?> <?php $query="SELECT * FROM lab_tbldeliveries WHERE deliveryid= '$deliveryid'"; $delivery=$db->select($query); if($delivery){ while($result=$delivery->fetch_assoc()){ $_SESSION["oldpic"]= $result['pic']; $_SESSION["gender"]= $result['gender']; $oldpic=$_SESSION["oldpic"]; ?> <form class="forms-sample" action="editdeliveries.php" method="POST" enctype="multipart/form-data"> <input type='hidden' name="deliveryid" value='<?php echo $deliveryid ?>' /> <div class="form-group row mb-3"> <label class="col-sm-2 col-form-label">Gender</label> <select class="form-select" name="gender" aria-label="Floating label select example"> <option selected>Open this select menu</option> <option value="girl">Girl</option> <option value="boy">Boy</option> <option value="woman">Woman</option> <option value="gentleman">Gentleman</option> </select> </div> <div class="form-group row mb-3"> <label for="exampleInputprename">Prename</label> <input type="text" name="prename" class="form-control" id="exampleInputprename" value="<?php echo $result['prename'];?>"> </div> <div class="form-group row mb-3"> <label for="exampleInputname">Name</label> <input type="text" name="deliveryname" class="form-control" id="exampleInputname" value="<?php echo $result['deliveryname'];?>"> </div> <div class="form-group row mb-3"> <label for="exampleInputaddress">Address</label> <input type="text" name="address" class="form-control" id="exampleInputaddress" value="<?php echo $result['address'];?>"> </div> <div class="form-group"> <label for="exampleInputpostcode">Postcode</label> <input type="text" name="postcode" class="form-control" id="exampleInputpostcode" value="<?php echo $result['postcode'];?>"> </div> <div class="form-group"> <label for="exampleInputcity">City</label> <input type="text" name="city" class="form-control" id="exampleInputcity" value="<?php echo $result['city'];?>"> </div> <div class="form-group"> <label for="exampleInputregion">Region</label> <input type="text" name="region" class="form-control" id="exampleInputregion" value="<?php echo $result['region'];?>"> </div> <div class="form-group"> <label for="exampleInputcountry">Country</label> <input type="text" name="country" class="form-control" id="exampleInputcountry" value="<?php echo $result['country'];?>"> </div> <div class="form-group"> <label for="exampleInputphone">Phone</label> <input type="text" name="phone" class="form-control" id="exampleInputphone" value="<?php echo $result['phone'];?>"> </div> <div class="form-group"> <label for="exampleInputemail">Email</label> <input type="email" name="email" class="form-control" id="exampleInputemail" value="<?php echo $result['email'];?>"> </div> <div> <label class="form-label">Image/Clip</label> <div> <input type="file" name="uploadFile" class="form-control"> </div> </div> <button class="btn btn-success" type="submit" name="submit">Update delivery </button> </form> <?php } }else{ echo "Failure within the system"; } ?> </div> </div><!-- Ende von Card --> </div> <div class="col-lg-1 col-md-1"></div> </div><!-- Ende von Row --> </section> </main> <?php include("footer.php"); ?> <?php function picdelete($pic) { if (file_exists($pic)) { if( @unlink($pic) !== true ) throw new Exception('Could not delete file: ' . $pic . ' Please close all applications that are using it.'); } return true; } ?>
Der Inhalt der Datei (admin/inc/approveanddeliveries.php)
 <?php if(!isset($_GET["deldeliveryid"]) || $_GET["deldeliveryid"]==NULL){ }else{ $id = $_GET["deldeliveryid"]; $query="DELETE FROM lab_tbldeliveries WHERE deliveryid='$id'"; $delivery=$db->delete($query); echo $delivery; } if(!isset($_GET["approvedeliveryid"]) || $_GET["approvedeliveryid"]==NULL){ } else{ $id = $_GET["approvedeliveryid"]; $query="UPDATE lab_tbldeliveries SET approved='yes' WHERE deliveryid='$id'"; $approved=$db->update($query); if($approved){ echo "<span style='color:#fff;'>Delivery message approvedد </span>"; }else{ echo "<span style='color:#fff;'>Delivery message not approved</span>"; } } if(!isset($_GET["rejectdeliveryid"]) || $_GET["rejectdeliveryid"]==NULL){ } else{ $id = $_GET["rejectdeliveryid"]; $query="UPDATE lab_tbldeliveries SET approved='no' WHERE deliveryid='$id'"; $approved=$db->update($query); if($approved){ echo "<span style='color:#fff;'>Delivery message rejected. </span>"; }else{ echo "<span style='color:#fff;'>Delivery message not rejected. </span>"; } } ?>
config.php
Diese Datei (config.php) beinhaltet den Code für Herstellung zur Datenbank.<?php mysql_connect("localhost", "dbshop", "dbshop_password"); mysql_select_db("dbshop"); function db_connect() { $result = new mysqli("localhost", "dbshop", "dbshop_password", "dbshop"); if (!$result) return FALSE; $result->autocommit(TRUE); return $result; } ?>
header.php
Diese Datei (header.php) beinhaltet das HTML-Grundgerüst, das Einbinden von CSS-, JS- und PHP-Dateien.<?php session_start(); include ("config/config.php"); if(!$_SESSION["items"]) $_SESSION["items"] = "0"; if(!$_SESSION["totalprice"]) $_SESSION["totalprice"] = "0.00"; ?> <!DOCTYPE html> <html lang="en"> <head> <meta charset="utf-8"> <meta content="width=device-width, initial-scale=1.0" name="viewport"> <title>Blogsystem V2.0</title> <meta content="" name="description"> <meta content="" name="keywords"> <!-- Favicons --> <link href="assets/img/favicon.png" rel="icon"> <link href="assets/img/peywand-icon.png" rel="apple-touch-icon"> <!-- Google Fonts --> <link rel="preconnect" href="https://fonts.googleapis.com"> <link rel="preconnect" href="https://fonts.gstatic.com" crossorigin> <link href="https://fonts.googleapis.com/css2?family=EB+Garamond:wght@400;500&family=Inter:wght@400;500&family=Playfair+Display:ital,wght@0,400;0,700;1,400;1,700&display=swap" rel="stylesheet"> <!-- Vendor CSS Files --> <link href="./assets/vendor/bootstrap/css/bootstrap.min.css" rel="stylesheet"> <link href="./assets/vendor/bootstrap-icons/bootstrap-icons.css" rel="stylesheet"> <link href="./assets/vendor/boxicons/css/boxicons.min.css" rel="stylesheet"> <link href="./assets/vendor/quill/quill.snow.css" rel="stylesheet"> <link href="./assets/vendor/quill/quill.bubble.css" rel="stylesheet"> <link href="./assets/vendor/remixicon/remixicon.css" rel="stylesheet"> <link href="https://cdn.jsdelivr.net/npm/summernote@0.8.20/dist/summernote-lite.min.css" rel="stylesheet"> <link href="./assets/vendor/datatables/style.css" rel="stylesheet" type="text/css"> <!-- range filter --> <link href="./assets/vendor/range/jquery-ui.css" rel = "stylesheet"> <script src="./assets/vendor/range/jquery-1.10.2.min.js"></script> <script src="./assets/vendor/range/jquery-ui.js"></script> <!-- Template Main CSS File --> <link href="./assets/css/maingallery.css" rel="stylesheet"> <link href="./assets/css/variables.css" rel="stylesheet"> <link href="./assets/css/main.css" rel="stylesheet"> </head> <body>
nav.php
Diese Datei (nav.php) beinhaltet den Code für die Navigation von Besucher.<!-- ======= Header ======= --> <header id="header" class="header d-flex align-items-center fixed-top"> <div class="container-fluid container-xl d-flex align-items-center justify-content-between"> <a href="index.php" class="logo d-flex align-items-center"> <!-- Uncomment the line below if you also wish to use an image logo --> <img src="assets/img/haw-logo.jpg" alt="Shopsystem"> <!--h1>LabBlog</h1--> </a> <nav id="navbar" class="navbar"> <ul> <li><a href="index.php"><span class="bi bi-house mx-1"></span> Home</a></li> <?php $sqlstr_cat="SELECT catid, catname FROM lab_tblcategories WHERE 1"; $res_cat = mysql_query($sqlstr_cat) or die ("no connection to the database ".mysql_error()); ?> <?php while ($row_cat = mysql_fetch_assoc($res_cat)) { ?> <li class="dropdown"> <a href="#"><span><?php echo $row_cat["catname"]; ?></span><i class="bi bi-chevron-down dropdown-indicator"></i></a> <ul> <?php $sqlstr_subcat="SELECT subcatid, catid, subcatname FROM lab_tblsubcategories WHERE catid=".$row_cat["catid"]; $res_subcat = mysql_query($sqlstr_subcat) or die ("no connection to the database ".mysql_error()); while ($row_subcat = mysql_fetch_assoc($res_subcat)) { echo "<li class='"; if($row_subcat["catid"]==3){ echo "'><a href='articles.php?subcatid=".$row_subcat["subcatid"]."'> ".$row_subcat["subcatname"]."</a></li>"; }else { echo "'><a href='articles.php?subcatid=".$row_subcat["subcatid"]."'> ".$row_subcat["subcatname"]."</a></li>"; } } ?> </ul> </li> <?php } mysql_free_result($res_cat); mysql_free_result($res_subcat); ?> <?php if ($_SESSION["customer_user"]){ ?> <li><a href="clogout.php" style="padding-top: 0px;"><span class="dropdown-heading fa fa-user"> LOGOUT </span></a></li> <?php } else { ?> <li><a href="clogin.php" style="padding-top: 0px;"><span class="dropdown-heading fa fa-user-plus"> LOGIN </span></a></li> <?php } ?> </ul> </nav><!-- .navbar --> <div class="position-relative"> <a href="cart.php" class="cart"><span><small> <?php echo $_SESSION['items']; ?></small><i class="bi bi-cart"></i></span></a> <a href="#" class="mx-2 js-search-open"><span class="bi-search"></span></a> <i class="bi bi-list mobile-nav-toggle"></i> <!-- ======= Search Form ======= --> <div class="search-form-wrap js-search-form-wrap"> <form action="search-result.html" class="search-form"> <span class="icon bi-search"></span> <input type="text" placeholder="Search" class="form-control"> <button class="btn js-search-close"><span class="bi-x"></span></button> </form> </div><!-- End Search Form --> </div> </div> </header><!-- End Header -->
footer.php
Diese Datei (footer.php) beinhaltet den Fußbereich und das Einbinden von JS-Dateien.<footer id="footer" class="footer"> <div class="container"> <div class="row justify-content-between"> <div class="col-md-12 text-center text-md-start mb-3 mb-md-0"> <div class="copyright"> <h4><b><a style="color:white; font-weight:900;" href="contact.php">Contact</a></b></h3> <span class="text-center text-sm-left d-md-inline-block">HAW Hamburg</span> </div> </div> </div> </div> </footer> <a href="#" class="scroll-top d-flex align-items-center justify-content-center" style="background: #68e8ea; color:#fff;"><i class="bi bi-arrow-up-short"></i></a> <script src="./assets/vendor/javascripts/jquery-3.3.1.min.js"></script> <script src="./assets/vendor/bootstrap/js/bootstrap.min.js"></script> <script src="./assets/vendor/apexcharts/apexcharts.min.js"></script> <script src="./assets/vendor/bootstrap/js/bootstrap.bundle.min.js"></script> <script src="./assets/vendor/chart.js/chart.umd.js"></script> <script src="./assets/vendor/echarts/echarts.min.js"></script> <script src="./assets/vendor/quill/quill.min.js"></script> <script src="./assets/vendor/summernote/summernote-lite.min.js"></script> <script src="./assets/vendor/summernote/lang/summernote-fa-IR.js"></script> <script src="./assets/vendor/datatables/datatables.js"></script> <script src="./assets/vendor/tinymce/tinymce.min.js"></script> <script src="./assets/vendor/php-email-form/validate.js"></script> <!-- Template Main JS File --> <script src="./assets/js/thismain.js"></script> <script> $('#summernote').summernote({ placeholder: 'Write a note', tabsize: 2, height: 400, lang: 'de-DE', // default: 'en-US' }); </script> </body> </html>
home.php
Diese Datei (home.php) beinhaltet Home-Seite.<?php include("header.php"); session_start(); ?> <header id="header" class="header d-flex align-items-center fixed-top"> <div class="container-fluid container-xl d-flex align-items-center justify-content-between"> <a href="index.php" class="logo d-flex align-items-center"> <!-- Uncomment the line below if you also wish to use an image logo --> <img src="assets/img/haw-logo.jpg" alt="Shopsystem"> <!--h1>ZenBlog</h1--> </a> <nav id="navbar" class="navbar"> <ul> <li><a href="home.php"><span class="fa fa-home"></span> HOME</a></li> <li><a href="description.php"><span class="fa fa-news"></span> DESCRIPTION</a></li> <li><a href="index.php"><span class="fa fa-cart"></span> CART</a></li> </ul> </nav><!-- .navbar --> </div> </header> <main id="main"> <!-- ======= Search Results ======= --> <section id="gallery" class="gallery"> <div class="container" data-aos="fade-up"> <div ="page-title"> <nav> <h3>Blogsystem - <?php echo date("l j F Y"); ?></h3> <h4><a href="home.php">Home</a></h4> <hr> </nav> </div><!-- End Page Title --> <div class="row"> <div class="col-md-1"></div> <div class="col-md-10"> <p class="lead">Lernform</p> Die Inhalte stehen als Online-Kurs (<a href="http://www.bandegani.de/itkurs">http://www.bandegani.de/itkurs/</a>) zum Selbststudium zur Verfügung. Die einzelnen Konzepte werden Schritt für Schritt aufeinander aufbauend in kleinen, überschaubaren Wissensbausteinen/Kapiteln vermittelt. Besonderer Wert wird auf jeweils vollständige Beispiele gelegt, die selbst weiterentwickelt werden können. Selbsttestaufgaben mit Musterlösungen erlauben es, die erworbenen Programmierfähigkeiten zu überprüfen. Am Anfang und am Ende jedes Wissensbausteins können die bereits vorhandenen Fähigkeiten oder die im Wissensbaustein erworbenen Fähigkeiten anhand von Tests und Aufgaben in dem privat zugelassenen Bereich überprüft werden. <hr> <p class="lead">Berufsfeldorientierung</p> Die Studierenden werden auf typische Problemstellungen in der beruflichen Praxis des Entwurfs und der Anwendungsentwicklung wie ein Content Management System für Online-Shops mit PHP und MySQL vorbereitet. <hr> <p class="lead">Webbasierte Datenbank</p> Diese Website (www.bandegani.de/itkurs) steht zum Selbststudium und zur eigenen Entwicklung zur Verfügung. Zur Selbstentwicklung muss man den Zugriff auf den eigenen Bereich, das FTP-Programm und die MYSQL-Datenbank haben. Diese Zugriffe werden nach dem Einschreiben in den Kurs ermöglicht und erlischen nach vier Jahren. Danach werden auch alle dort gespeicherten Daten aus dem System entfernt. <hr> <p class="lead">Mögliche Themen für die Hausarbeit</p> <ul> <li>Online-Shop im Bereich Bekleidungstechnik,</li> <li>Protokollierung der Arbeitsvorgänge in einem Unternehmen,</li> <li>Einkauf von Rohmaterialien bei verschiedenen Lieferanten,</li> <li>Lagerbestandssystem für die Materialien,</li> <li>Erstellung von Vor- und Nachkalkulationen für die Materialien, Maschinen und Personalien,</li> <li>Auswertung für die Zufriedenheit der Mitarbeiter, Kunden, usw.,</li> <li>Datenbanksysteme für Stoffe, Veredlungen, Zubehör, Kleidung, Lieferanten, Kunden, Mitarbeiter, Maßtabellen, Passformklassen, Gehälter usw.</li> </ul> <hr> <p class="lead">Hilfestellung</p> Sie können für die Realisation Ihrer Hausarbeit auch ein beliebiges Open Source System benutzen, das auf PHP5, MySQL und JavaScript basiert ist. Vor der Installation muss aber das Open Source System von der Kursleiterin oder dem Kursleiter genehmigt werden. <hr> <p class="lead">Organisation, Voraussetzung und Gewichtung</p> In der Lehrveranstaltung werden Konzept, Systemstruktur, Hilfsmittel, Vorlagen und wesentliche Informationen zu der Entwicklung von einem Online-System besprochen. Die Studierenden müssen dem Kursleiter das Thema der Hausarbeit spätestens 5 Wochen vor dem Prüfungstermin bekannt geben. Die Studierenden müssen ihre Hausarbeit am Prüfungstermin präsentiert. An dem Termin sollen alle eingeschriebene Studierenden teilnehmen und die Präsentationen anhören. Die Präsentation und die Anwesenheit der Studierenden fließen mit in die Note ein. Für das Bestehen des Kurses erhalten Sie 5 CP's. </div><!-- End col-lg-10 --> <div class="col-md-1"></div> </div><!-- End row --> </div><!-- End container --> </section> <!-- End Search Result --> </main><!-- End #main --> <?php include("footer.php"); ?>
index.php
Diese Datei (index.php) beinhaltet Startseite.<?php session_start(); include("header.php"); include("nav.php"); if (isset($_GET["pageno"])) { $pageno = $_GET["pageno"]; } else { $pageno = 1; } $no_of_records_per_page = 10; $offset = ($pageno-1) * $no_of_records_per_page; ?> <main id="main"> <!-- ======= Search Results ======= --> <section id="gallery" class="gallery"> <div class="container" data-aos="fade-up"> <div ="page-title"> <nav> <h3>Blogsystem - <?php echo date("l j F Y"); ?></h3> <h4><a href="home.php">Home</a></h4> <hr> </nav> </div><!-- End Page Title --> <div class="row"> <div class="col-md-2"> <div class="card"> <div class="card-body pb-0"> <h4 class="card-title">Meistgesehen</h4> <hr> <div class="news"> <?php $sqlstr="SELECT * FROM lab_tblarticles where approved='yes' ORDER BY counter DESC LIMIT 5"; $res = mysql_query($sqlstr) or die ("no connection to the database ".mysql_error()); while ($result = mysql_fetch_assoc($res)) { ?> <div class="post-item clearfix"> <?php if ($result["type"] == "image") { if($result["pic"]=="assets/img/logo_shop.png"){?> <?php } else { ?> <img src="admin/<?php echo $result["pic"]; ?>" class="img-fluid"> <?php } } else if ($result["type"] == "video") { ?> <video src="admin/<?php echo $result["pic"]; ?>" type="video/mp4" controls/></video> <?php } ?> <h5><a href="articlesdetails.php?articleid=<?php echo $result["articleid"];?>"> <?php echo $result["articlename"];?></a></h5> </div> <?php } mysql_free_result($res); ?> </div> </div> </div> </div><!-- Ende von rechte Seite --> <div class="col-md-8"> <div class="row filter_data"> <?php $sqlstr = "SELECT COUNT(*) FROM lab_tblarticles"; $res = mysql_query($sqlstr) or die ("no connection to the database ".mysql_error()); $total_rows = mysql_fetch_array($res)[0]; $total_pages = ceil($total_rows / $no_of_records_per_page); $sqlstr_limit = "SELECT * FROM lab_tblarticles WHERE approved='yes' ORDER BY modified DESC LIMIT $offset, $no_of_records_per_page"; $res= mysql_query($sqlstr_limit) or die ("no connection to the database ".mysql_error()); $i=0; while ($result = mysql_fetch_assoc($res)) { $i=$i+1; if($i<=1){ // 2 Rows muss big ?> <div class="col-md-12"> <div class="gallery-item h-100"> <?php if ($result['type'] == "image") { ?> <img src="admin/<?php echo $result['pic']; ?>" class="img-fluid"> <?php } else if ($result['type'] == "video") { ?> <video src="admin/<?php echo $result['pic']; ?>" type='video/mp4' controls/></video> <?php }?> <div class="gallery-links d-flex align-items-center justify-content-center"> <a href="articlesdetails.php?articleid=<?php echo $result['articleid'];?>" class="details-link"><i class="bi bi-link-45deg"></i></a> </div> <div class="post-meta"> <span class="date"><?php echo $result['modified'];?></span> <span class="mx-2">•</span> <span class="category"> <?php echo $result['counter']; ?><i class="bi bi-eye-fill"></i> </span> </div> <h4><?php echo shortText($result['articlename'], 150); ?></h4> </div> </div> <?php } else { ?> <div class="col-md-4"> <div class="gallery-item h-100"> <?php if ($result['type'] == "image") { ?> <img src="admin/<?php echo $result['pic']; ?>" class="img-fluid"> <?php } else if ($result['type'] == "video") { ?> <video src="admin/<?php echo $result['pic']; ?>" type='video/mp4' controls/></video> <?php }?> <div class="gallery-links d-flex align-items-center justify-content-center"> <a href="articlesdetails.php?articleid=<?php echo $result['articleid'];?>" class="details-link"><i class="bi bi-link-45deg"></i></a> </div> <div class="post-meta"> <span class="date"><?php echo $result['modified'];?></span> <span class="mx-2">•</span> <span class="category"> <?php echo $result['counter']; ?><i class="bi bi-eye-fill"></i> </span> </div> <h4><?php echo shortText($result['articlename'], 150); ?></h4> </div> </div> <?php } // End of rest } mysql_free_result($res); ?> </div><!-- Ende von Row in Conent --> <hr> <div class="row"> <!-- Pagination with icons --> <nav aria-label="Page navigation example"> <ul class="pagination"> <li class="page-item"> <a class="page-link" href="?pageno=1" aria-label="Previous"> <span aria-hidden="true">«</span> </a> </li> <li class="page-item" class="<?php if($pageno <= 1){ echo 'disabled'; } ?>"> <a class="page-link" href="<?php if($pageno <= 1){ echo '#'; } else { echo "?pageno=".($pageno - 1); } ?>"> <?php echo $pageno; ?></a></li> <li class="page-item" class="<?php if($pageno >= $total_pages){ echo 'disabled'; } ?>"> <a class="page-link" href="<?php if($pageno >= $total_pages){ echo '#'; } else { echo "?pageno=".($pageno + 1); } ?>"> <?php echo ($pageno+1); ?></a></li> <li class="page-item"> <a class="page-link" href="?pageno=<?php echo $total_pages; ?>" aria-label="Next"> <span aria-hidden="true">»</span> </a> </li> </ul> </nav><!-- End Pagination with icons --> </div> </div><!-- end col-8 --> <div class="col-md-2 sm-padding"> <div class="card"> <div class="card-body pb-0"> <h4 class="card-title">Neu eingetroffen</h4> <hr> <div class="news"> <?php $sqlstr="SELECT * FROM lab_tblarticles where approved='yes' ORDER BY modified DESC LIMIT 5"; $res = mysql_query($sqlstr) or die ("no connection to the database ".mysql_error()); while ($result = mysql_fetch_assoc($res)) { ?> <div class="post-item clearfix"> <?php if ($result['type'] == "image") { if($result['pic']=="assets/img/logo_shop.png"){?> <?php } else { ?> <img src="admin/<?php echo $result['pic']; ?>" class="img-fluid"> <?php } } else if ($result['type'] == "video") { ?> <video src="admin/<?php echo $result['pic']; ?>" type="video/mp4" controls/></video> <?php } ?> <h5><a href="articlesdetails.php?articleid=<?php echo $result['articleid'];?>"> <?php echo $result['articlename'];?></a></h5> </div> <?php } mysql_free_result($res); ?> </div> </div><!-- End of card -body --> </div><!-- End of card --> </div><!-- End col-lg-2 --> </div><!-- End row --> </div><!-- End container --> </section> <!-- End Search Result --> </main><!-- End #main --> <?php function shortText($string,$lenght) { if(strlen($string) > $lenght) { $string = substr($string,0,$lenght)."..."; $string_ende = strrchr($string, " "); $string = str_replace($string_ende," ...", $string); } return $string; } ?> <?php include("footer.php"); ?>
articles.php
Diese Datei (articles.php) zeigt alle Artikeln in Abhängigkeit von einer Unterkategorie.<?php session_start(); include("header.php"); include("nav.php"); $subcatid = $_GET["subcatid"]; if ($subcatid<=0 ) { $subcatid=1; } $_SESSION["subcatid"] = $subcatid; if (isset($_GET["pageno"])) { $pageno = $_GET["pageno"]; } else { $pageno = 1; } $no_of_records_per_page = 10; $offset = ($pageno-1) * $no_of_records_per_page; ?> <main id="main"> <!-- ======= Search Results ======= --> <section id="gallery" class="gallery"> <div class="container" data-aos="fade-up"> <?php $sqlstr="SELECT * FROM lab_tblsubcategories WHERE subcatid = ". $subcatid; $res = mysql_query($sqlstr) or die ("no connection to the database ".mysql_error()); $result = mysql_fetch_array($res); ?> <div ="page-title"> <nav> <h3>Blogsystem - <?php echo date("l j F Y"); ?></h3> <h4><a href="index.php">Home</a> / <a href="articles.php?subcatid=<?php echo $result["subcatid"]; ?>"><?php echo $result["subcatname"]; ?></a></h4> <hr> </nav> </div><!-- End Page Title --> <?php mysql_free_result($res); ?> <div class="row"> <div class="col-md-2"> <div class="card"> <div class="card-body pb-0"> <h4 class="card-title">Meistgesehen</h4> <hr> <div class="news"> <?php $sqlstr="SELECT * FROM lab_tblarticles where approved='yes' ORDER BY counter DESC LIMIT 5"; $res = mysql_query($sqlstr) or die ("no connection to the database ".mysql_error()); while ($result = mysql_fetch_assoc($res)) { ?> <div class="post-item clearfix"> <?php if ($result["type"] == "image") { if($result["pic"]=="assets/img/logo_shop.png"){?> <?php } else { ?> <img src="admin/<?php echo $result["pic"]; ?>" class="img-fluid"> <?php } } else if ($result["type"] == "video") { ?> <video src="admin/<?php echo $result["pic"]; ?>" type="video/mp4" controls/></video> <?php } ?> <h5><a href="articlesdetails.php?articleid=<?php echo $result["articleid"];?>"> <?php echo $result["articlename"];?></a></h5> </div> <?php } mysql_free_result($res); ?> </div> </div> </div> </div><!-- Ende von rechte Seite --> <div class="col-md-9"> <div class="row filter_data"> <?php $sqlstr = "SELECT COUNT(*) FROM lab_tblarticles"; $res = mysql_query($sqlstr) or die ("no connection to the database ".mysql_error()); $total_rows = mysql_fetch_array($res)[0]; $total_pages = ceil($total_rows / $no_of_records_per_page); mysql_free_result($res); $sqlstr_limit = "SELECT * FROM lab_tblarticles WHERE approved='yes' ORDER BY modified DESC LIMIT $offset, $no_of_records_per_page"; $res= mysql_query($sqlstr_limit) or die ("no connection to the database ".mysql_error()); while ($result = mysql_fetch_assoc($res)) { ?> <div class="col-md-4"> <div class="gallery-item h-100"> <?php if ($result["type"] == "image") { ?> <img src="admin/<?php echo $result["pic"]; ?>" class="img-fluid"> <?php } else if ($result["type"] == "video") { ?> <video src="admin/<?php echo $result["pic"]; ?>" type="video/mp4" controls/></video> <?php }?> <div class="gallery-links d-flex align-items-center justify-content-center"> <a href="articlesdetails.php?articleid=<?php echo $result["articleid"];?>" class="details-link"><i class="bi bi-link-45deg"></i></a> </div> <div class="post-meta"> <span class="date"><?php echo $result["modified"];?></span> <span class="mx-2">•</span> <span class="category"> <?php echo $result["counter"]; ?><i class="bi bi-eye-fill"></i> </span> </div> <h4><?php echo shortText($result["articlename"], 150); ?></h4> </div> </div> <?php } mysql_free_result($res); ?> </div><!-- Ende von Row in Conent --> <hr> <div class="row"> <!-- Pagination with icons --> <nav aria-label="Page navigation example"> <ul class="pagination"> <li class="page-item"> <a class="page-link" href="?pageno=1" aria-label="Previous"> <span aria-hidden="true">«</span> </a> </li> <li class="page-item" class="<?php if($pageno <= 1){ echo 'disabled'; } ?>"> <a class="page-link" href="<?php if($pageno <= 1){ echo '#'; } else { echo "?pageno=".($pageno - 1); } ?>"> <?php echo $pageno; ?></a></li> <li class="page-item" class="<?php if($pageno >= $total_pages){ echo 'disabled'; } ?>"> <a class="page-link" href="<?php if($pageno >= $total_pages){ echo '#'; } else { echo "?pageno=".($pageno + 1); } ?>"> <?php echo ($pageno+1); ?></a></li> <li class="page-item"> <a class="page-link" href="?pageno=<?php echo $total_pages; ?>" aria-label="Next"> <span aria-hidden="true">»</span> </a> </li> </ul> </nav><!-- End Pagination with icons --> </div> </div><!-- end col-8 --> <div class="col-md-1"></div> </div><!-- End row --> </div><!-- End container --> </section> <!-- End Search Result --> </main><!-- End #main --> <?php include("footer.php"); ?> <?php function shortText($string,$lenght) { if(strlen($string) > $lenght) { $string = substr($string,0,$lenght)."..."; $string_ende = strrchr($string, " "); $string = str_replace($string_ende," ...", $string); } return $string; } ?>
articlesdetails.php
Diese Datei (articlesdetails.php) stellt die Dtails von einem Artikel.<?php session_start(); include("header.php"); include("nav.php"); // Set session variables that we want to access within the function if(!$_SESSION['items']) $_SESSION['items'] = '0'; if(!$_SESSION['totalprice']) $_SESSION['totalprice'] = '0.00'; if(isset($_POST['articleid'])){ $articleid = $_POST['articleid']; }elseif(!isset($_GET['articleid']) ||$_GET['articleid']==NULL ){ }else{ $articleid=$_GET['articleid']; } $subcatid = $_GET['subcatid']; if ($subcatid<=0 ) { $subcatid=1; } $_SESSION['subcatid'] = $subcatid; ?> <main id="main"> <!-- ======= Search Results ======= --> <section id="gallery" class="gallery"> <div class="container" data-aos="fade-up"> <?php $sqlstr="SELECT * FROM lab_tblsubcategories WHERE subcatid = ". $subcatid; $res = mysql_query($sqlstr) or die ("no connection to the database ".mysql_error()); $result = mysql_fetch_array($res); ?> <div ="page-title"> <nav> <h4><a href="index.php">Home</a> / <a href="articlesdetails.php?subcatid=<?php echo $result['subcatid']; ?>"><?php echo $result['subcatname']; ?></a></h4> <hr> </nav> </div><!-- End Page Title --> <?php mysql_free_result($res); ?> <div class="row gy-4 justify-content-center"> <div class="col-md-2 sm-padding"> <div class="card"> <div class="card-body pb-0"> <h4 class="card-title">Neu eingetroffen</h4> <hr> <div class="news"> <?php $sqlstr="SELECT * FROM lab_tblarticles where approved='yes' ORDER BY modified DESC LIMIT 5"; $res = mysql_query($sqlstr) or die ("no connection to the database ".mysql_error()); while ($result = mysql_fetch_assoc($res)) { ?> <div class="post-item clearfix"> <?php if ($result['type'] == "image") { if($result['pic']=="assets/img/logo_shop.png"){?> <?php } else { ?> <img src="admin/<?php echo $result['pic']; ?>" class="img-fluid"> <?php } } else if ($result['type'] == "video") { ?> <video src="admin/<?php echo $result['pic']; ?>" type="video/mp4" controls/></video> <?php } ?> <h5><a href="articlesdetails.php?articleid=<?php echo $result["articleid"];?>"> <?php echo $result['articlename'];?></a></h5> </div> <?php } mysql_free_result($res); ?> </div> </div><!-- End of card -body --> </div><!-- End of card --> </div><!-- End col-lg-2 --> <?php $sqlstr="SELECT * FROM lab_tblarticles WHERE approved='yes' AND articleid=$articleid"; $res = mysql_query($sqlstr) or die ("no connection to the database ".mysql_error()); $result = mysql_fetch_array($res); ?> <div class="col-md-10"> <div class="card"> <div class="card-header"> <h5 class="card-title">Card with titles, buttons, and links</h5> </div> <div class="card-body"> <span style="color:black; font-size:16px;font-weight:bold;"><?php echo $result['articlename']; ?></span> <p style="color:red; font-size:20px;font-weight:bold;"><?php echo $result['number']; ?></p> <?php if ($result['type'] == "image") { ?> <img src="admin/<?php echo $result['pic']; ?>" style="float:right;margin-left:10px; width:30%; height:auto;"> <?php } else if ($result['type'] == "video") { ?> <video src="admin/<?php echo $result['pic']; ?>" type='video/mp4' style="float:right;margin-left:10px; width:30%; height:auto;" controls/></video> <?php } ?> <?php echo $result['description']; ?> <hr> <p class="card-text"><a href="cart.php?new=<?php echo $result['articleid'];?>" class="card-link">Shopping</a> <a href="index.php" class="card-link">add shopping</a></p> </div> </div> </div> <?php mysql_free_result($res); ?> </div><!-- End of row --> </div><!-- End container --> </section> <!-- End Search Result --> </main> <?php include("footer.php"); ?>
cart.php
Diese Datei (cart.php) stellt den Warenkorb dar.<?php session_start(); include("header.php"); include("nav.php"); if(isset($_POST["articleid"])){ $articleid = $_POST["articleid"]; }elseif(!isset($_GET["articleid"]) ||$_GET["articleid"]==NULL ){ header("Location:articlesdetails.php"); }else{ $articleid=$_GET["articleid"]; } $subcatid=$_SESSION["subcatid"]; @ $new = $_GET["new"]; if ($new) { // new article has been selected if (!isset($_SESSION["cart"])) { $_SESSION["cart"] = array(); $_SESSION["items"] = 0; $_SESSION["totalprice"] = "0.00"; } if (isset($_SESSION["cart"][$new])) $_SESSION["cart"][$new]++; else $_SESSION["cart"][$new] = 1; $_SESSION["totalprice"] = calculate_price($_SESSION["cart"]); $_SESSION["items"] = calculate_items($_SESSION["cart"]); } if (isset($_POST["save"])) { foreach ($_SESSION["cart"] as $nummer => $qty) { if ($_POST[$nummer] == "0") unset($_SESSION["cart"][$nummer]); else $_SESSION["cart"][$nummer] = $_POST[$nummer]; } $_SESSION["totalprice"] = calculate_price($_SESSION["cart"]); $_SESSION["items"] = calculate_items($_SESSION["cart"]); } ?> <main id="main"> <!-- ======= Search Results ======= --> <section id="gallery" class="gallery"> <div class="container" data-aos="fade-up"> <div ="page-title"> <nav> <h3>Blogsystem - <?php echo date("l j F Y"); ?></h3> <h4><a href="home.php">Home</a></h4> <hr> </nav> </div><!-- End Page Title --> <div class="row"> <div class="col-lg-2 col-md-2 col-sm-12 col-xs-12"> <div class="card"> <div class="card-body pb-0"> <h4 class="card-title">Meistgesehen</h4> <hr> <div class="news"> <?php $sqlstr="SELECT * FROM lab_tblarticles where approved='yes' ORDER BY counter DESC LIMIT 5"; $res = mysql_query($sqlstr) or die ("no connection to the database ".mysql_error()); while ($result = mysql_fetch_assoc($res)) { ?> <div class="post-item clearfix"> <?php if ($result['type'] == "image") { if($result['pic']=="assets/img/logo_shop.png"){?> <?php } else { ?> <img src="admin/<?php echo $result['pic']; ?>" class="img-fluid"> <?php } } else if ($result['type'] == "video") { ?> <video src="admin/<?php echo $result['pic']; ?>" type="video/mp4" controls/></video> <?php } ?> <h5><a href="articlesdetails.php?articleid=<?php echo $result['articleid'];?>"> <?php echo $result['articlename'];?></a></h5> </div> <?php } mysql_free_result($res); ?> </div> </div> </div> </div><!-- Ende von rechte Seite --> <div class="col-lg-10 col-md-10 col-sm-12 col-xs-12"> <?php if ($_SESSION["cart"] && array_count_values($_SESSION["cart"])) display_cart($_SESSION["cart"]); else {?> <div id="page-wrapper"><div class="container-fluid"> <div class="bg-darkred fg-white"> This selected article was not accepted! </div> </div></div> <?php } if ($new) { $details = get_articlesdetails($new); if ($details["subcatid"]) $url = "articles.php?subcatid=" . $details["subcatid"]; } ?> </div><!-- end col --> </div><!-- end row --> </div><!-- end container --> </section><!-- end section --> </main><!-- End #main --> <?php include("footer.php"); ?> <?php function display_cart($cart, $change = TRUE) {?> <div class="table-responsive"> <table class="table table-bordered"> <form method="post" enctype="multipart/form-data" action="cart.php"> <thead> <tr><th>Pos.</th><th>Bild</th><th>Name</th><th>Beschreibung</th><th>Preis</th> <th>Anzahl</th><th>Gesamt</th></tr> </thead> <tbody> <?php $i=0; foreach ($cart as $articleid => $qty) { $i=$i+1; $article = get_articlesdetails($articleid); ?> <tr><td><?php echo $i; ?> </td> <td> <?php if ($article["type"] == "image") { if($article["pic"]=="assets/img/logo_shop.png"){?> <?php } else { ?> <img src="admin/<?php echo $article["pic"]; ?>" width="auto" height="64px"> <?php } } else if ($article["type"] == "video") { ?> <video src="admin/<?php echo $article["pic"]; ?>" type="video/mp4" width="auto" height="64px" controls/></video> <?php } ?> </td> <?php $articleurl = "articlesdetails.php?articleid=" . $articleid; ?> <td><a href = "<?php echo $articleurl; ?>"><?php echo $article["articlename"]; ?></a></td> <td><?php echo $article["description"]; ?></td> <td><?php echo number_format($article["price"], 2); ?></td> <?php // If we allow changes, sets are in text boxes if ($change == TRUE) echo "<td><input type='text' name=$articleid value=$qty size=3></td>"; else echo $qty; echo "<td align='center'>" . number_format($article["price"] * $qty, 2) . "</td>"; ?> </tr> <?php } ?> <!-- Show line with total --> </tbody> <thead> <tr> <th colspan = "<?php echo (4 + $images); ?>"></th> <th><?php echo $_SESSION["items"]; ?></th> <th><?php echo number_format($_SESSION["totalprice"], 2); ?></th> </tr> </thead> <!--save changes Button --> <?php if ($change == TRUE) { ?> <tr> <td colspan = "<?php echo (4 + $images); ?>"></td> <td> <input type = "hidden" name = "save" value = true> <button type="image" name="save Changes" class="btn btn-primary"> <span class="fa fa-save "></span> Änderung speichern</button> </td> <td></td> </tr> <?php } ?> <a href="articles.php?subcatid=<?php echo $subcatid; ?>" class="button"> <span class="fa fa-shopping-cart"></span> add shopping</a> <a href="clogin.php" class="button"><span class="fa fa-paypal"></span> Continue to pay</a> </form> </table> </div> <?php } function get_articles($subcatid) { // Query database for the articles of a category if (!$subcatid || $subcatid == "") return FALSE; $sqlstr = "select * from lab_tblarticles where subcatid='$subcatid'"; $res = mysql_query($sqlstr) or die ("no connection to the database ".mysql_error()); $num = mysql_num_rows($res); if ($num == 0) return FALSE; for ($count = 0; $row=mysql_fetch_assoc($res); $count++) $res_array[$count] = $row; return $res_array; } function calculate_price($cart) { // Calculate the total price for all items in the shopping cart $price = 0.0; if (is_array($cart)) { $conn = db_connect(); foreach($cart as $articleid => $qty) { $sqlstr = "select price from lab_tblarticles where articleid='$articleid'"; $res = $conn->query($sqlstr); if ($res) { $item = $res->fetch_object(); $item_price = $item->price; $price += $item_price * $qty; } } } return $price; } function calculate_items($cart) { // Calculate the number of items in your cart $items = 0; if (is_array($cart)) { foreach($cart as $articleid => $qty) { $items += $qty; } } return $items; } function get_articlesdetails($articleid) { if (!$articleid || $articleid == "") return FALSE; $conn = db_connect(); $sqlstr = "select * from lab_tblarticles where articleid='$articleid'"; $res = @$conn->query($sqlstr); if (!$res) return FALSE; $res = @$res->fetch_assoc(); return $res; } ?>
clogin.php
Diese Datei (clogin.php) wird aufgerufen, wenn der Kunde sich anmeldet, um die ausgewählten Artikeln zu bestellen.<?php session_start(); include("header.php"); include("nav.php"); ?> <main id="main"> <!-- ======= Search Results ======= --> <section id="gallery" class="gallery"> <div class="container" data-aos="fade-up"> <div ="page-title"> <nav> <h3>Blogsystem - <?php echo date("l j F Y"); ?></h3> <h4><a href="home.php">Home</a></h4> <hr> </nav> </div><!-- End Page Title --> <div class="row"> <div class="col-lg-3 col-md-3 col-sm-12 col-xs-12"></div><!-- end col --> <div class="col-lg-6 col-md-6 col-sm-12 col-xs-12"> <div class="panel panel-primary"><!-- Begin Panel --> <div class="panel-heading" style="background: #f8f8f8; color:#696969;"> <h2 class="panel-title">Customer Login - Formular</h2> </div> <div class="panel-body"> <form enctype="multipart/form-data" method="post" action="clogin.php"> <div class="form-group"> <label class="control-label" for="inputUsername">Username:</label> <input type="text" name="email" class="form-control" id="inputUsername" required> <div style="height: 15px;"></div> </div> <div class="form-group"> <label class="control-label" for="inputPassword">Password:</label> <input type="Password" name="password" class="form-control" id="inputPassword" required> <div style="height: 15px;"></div> </div> <div class="form-group"> <button type="submit" name="send" class="btn btn-success"> <span class="fa fa-lock"></span> Login</button> </div> <div class="form-group"> <a style="color:#696969" href="cregister.php" class="button"><span class="fa fa-user-plus"></span>create Account</a> </div> </form> </div> </div><!-- End Panel --> <?php if(isset($_POST["send"])) { if ($_POST["email"] && $_POST["password"]) { $email = $_POST["email"]; $password=MD5($_POST["password"]); $sqlstr = "select * from lab_tblcustomers where email='$email' and password = '$password'"; $res = mysql_query($sqlstr) or die ("no connection to the database ".mysql_error()); $num = mysql_fetch_array($res); if ($num["email"]==$email && $num["password"]==$password){ $_SESSION["customer_user"] = $email; $_SESSION["customerid"] = $num["customerid"]; //header("Location:cart.php"); ?> <p><a class="btn btn-success" href="cart.php">Click to see your cart</a></p> <small class="text-muted">You are logged in now</small> <?php } else { ?> <h4 style="color:red;">You could not be logged in!</h4> <?php } } else {?> <h4 style="color:red;">Your username or password is incorrect!</h4> <?php } mysql_free_result($res); } ?> </div><!-- end col --> <div class="col-lg-3 col-md-3 col-sm-12 col-xs-12"></div><!-- end col --> </div><!-- end row --> </div><!-- end container --> </section><!-- end section --> </main> <?php include("footer.php"); ?>
clogout.php
Diese Datei (clogout.php) wird aufgerufen, wenn der Kunde sich abmelden will.<?php session_start(); $old_user = $_SESSION["customer_user"]; unset($_SESSION["customer_user"]); session_destroy(); if (!empty($old_user)) { require_once("header.php"); require_once("nav.php"); ?> <div id="page-wrapper"><div class="container-fluid"> <div class="row"> <div class="col-md-2 col-lg-2 col-sm-12 col-xs-12"></div> <span class="label label-success">You are Logout!</span> </div> </div> </div> <?php require_once("footer.php"); } else { header("Location: clogin.php"); } ?>
cregister.php
Diese Datei (cregister.php) wird aufgerufen, wenn der Kunde sich neu registrieren will.<?php session_start(); include("header.php"); include("nav.php"); $uploadDirectory = __DIR__."/"; ?> <main id="main" class="main"> <div class="pagename"> <nav> <ol class="breadcrumb"> <li class="breadcrumb-item"><a href="index.php">Home</a></li> <li class="breadcrumb-item">Register</li> <li class="breadcrumb-item active">Add new customer</li> </ol> </nav> </div><!-- End Page Title --> <section class="section"> <div class="row"> <div class="col-lg-3 col-md-3"></div> <div class="col-lg-6 col-md-6"> <div class="card"> <div class="card-body"> <h2>Add new customer</h2> <?php if(isset($_FILES["uploadFile"])){ $errors= array(); $file_name = $_FILES["uploadFile"]["name"]; $file_size = $_FILES["uploadFile"]["size"]; $file_tmp = $_FILES["uploadFile"]["tmp_name"]; $file_type = $_FILES["uploadFile"]["type"]; $file_ext=strtolower(end(explode(".",$_FILES["uploadFile"]["name"]))); $unique_pic = substr(md5(time()), 0, 10).".".$file_ext; $uploaded_pic = "admin/uploads/customers/".$unique_pic; if(!empty($file_name)){ if(empty($errors)==true) { $type = pathinfo($file_name, PATHINFO_EXTENSION); $fileExists = true; while ($fileExists) { if (!file_exists($uploadDirectory.$uploaded_pic)) { $fileExists = false; } } move_uploaded_file($file_tmp,$uploadDirectory.$uploaded_pic); $mediaType = "something"; switch ($type) { case "mp4": case "mkv": case "mov": case "ogg": case "webm": $mediaType = "video"; break; case "jpg": case "jpeg": case "gif": case "png": default: $mediaType = "image"; break; } } }else { $uploaded_pic = "admin/uploads/customers/shop_logo.gif"; $mediaType = "pic"; } $userid=1; $approved='yes'; $prename= $_POST["prename"]; $customername = $_POST["customername"]; $gender = $_POST["gender"]; $address = $_POST["address"]; $city = $_POST["city"]; $region = $_POST["region"]; $country = $_POST["country"]; $postcode = $_POST["postcode"]; $phone = $_POST["phone"]; $email = $_POST["email"]; $password = MD5($_POST["password"]); $sqlstr = "INSERT INTO lab_tblcustomers (prename, customername, gender, address, city, region, country, postcode, phone, email, password,pic, type, modified, userid, approved) VALUES('$prename', '$customername', '$gender', '$address', '$city', '$region', '$country', '$postcode', '$phone', '$email', '$password', '$uploaded_pic', '$mediaType', NOW() , '$userid', '$approved')"; $res = mysql_query($sqlstr) or die ("no connection to the database ".mysql_error()); $num = mysql_affected_rows(); if ($num>0) { echo '<div class="alert alert-success bg-success text-light border-0 alert-dismissible fade show" role="alert"> The Customerhas been added! </div>'; }else { echo '<div class="alert alert-danger bg-danger text-light border-0 alert-dismissible fade show" role="alert"> An error occurred while adding the Customer to the system! </div>'; } }else{ print_r($errors); } ?> <form class="forms-sample" action="cregister.php" method="POST" enctype="multipart/form-data"> <div class="form-group row mb-3"> <label class="col-sm-2 col-form-label">Gender</label> <select class="form-select" name="gender" aria-label="Floating label select example"> <option selected>Open this select menu</option> <option value="girl">Girl</option> <option value="boy">Boy</option> <option value="woman">Woman</option> <option value="gentleman">Gentleman</option> </select> </div> <div class="form-group row mb-3"> <label for="exampleInputprename">Prename</label> <input type="text" name="prename" class="form-control" id="exampleInputprename" placeholder="Elke"> </div> <div class="form-group row mb-3"> <label for="exampleInputname">Name</label> <input type="text" name="customername" class="form-control" id="exampleInputname" placeholder="Raza"> </div> <div class="form-group row mb-3"> <label for="exampleInputaddress">Address</label> <input type="text" name="address" class="form-control" id="exampleInputaddress" placeholder="Armgartstraße 24"> </div> <div class="form-group"> <label for="exampleInputpostcode">Postcode</label> <input type="text" name="postcode" class="form-control" id="exampleInputpostcode" placeholder="22179"> </div> <div class="form-group"> <label for="exampleInputcity">City</label> <input type="text" name="city" class="form-control" id="exampleInputcity" placeholder="Hamburg"> </div> <div class="form-group"> <label for="exampleInputregion">Region</label> <input type="text" name="region" class="form-control" id="exampleInputregion" placeholder="Hamburg"> </div> <div class="form-group"> <label for="exampleInputcountry">Country</label> <input type="text" name="country" class="form-control" id="exampleInputcountry" placeholder="Germany"> </div> <div class="form-group"> <label for="exampleInputphone">Phone</label> <input type="text" name="phone" class="form-control" id="exampleInputphone" placeholder="040/4285238"> </div> <div class="form-group"> <label for="exampleInputemail">Email</label> <input type="email" name="email" class="form-control" id="exampleInputemail" placeholder="elke.raza@haw-hamburg.de"> </div> <div class="form-group"> <label for="exampleInputpassword">Password</label> <input type="password" name="password" class="form-control" id="exampleInputpassword"> </div> <div> <label class="form-label">Image/Clip</label> <div> <input type="file" name="uploadFile" class="form-control"> </div> </div> <button class="btn btn-success" type="submit" name="submit">Add customer</button> </form> </div> </div><!-- Ende von Card --> </div> <div class="col-lg-3 col-md-3"></div> </div><!-- Ende von Row --> </section> </main> <?php include("footer.php"); ?>
Vorbereitung für den Einsatz von einem 2. Template
Wenn Sie in oben beschriebenen Blogsystem einen Ordner für "admin" festgelegt haben,sollen Sie auf dem gleichen Ebene einen weiteren Ordner mit dem Namen z.B. "template2" erstellen. Laden Sie dann die CSS- und JS-Dateien static.zip runter. Dann entpacken Sie die Datei "static.zip" auf Ihren lokalen Rechner. Dann müssen alle Dateien bzw. den Ordner "static" in dem Ordner "template2" hochgeladen werden. Damit der vorgegebene Code für die Einbindung der Dateien stimmen kann, müssen die Ordner "admin" und "template2" auf dem gleichen Ebene sein. Alle Dateien, die unten codiert sind, müssen dann in Ordner "template2" gespeichert werden.
Verzeichnisstruktur für Template2 - Basissystems Blogsystem
Cart-Tabelle
Die Tabelle kann man entweder mittels phpMyAdmin (Hilfsprogramm für die Verwaltung der Tabellen) erstellen oder unten stehenden SQL-Code kopieren und ausführen.Die Datenbanktabelle (lab_tblcart) hat folgende Spalten:
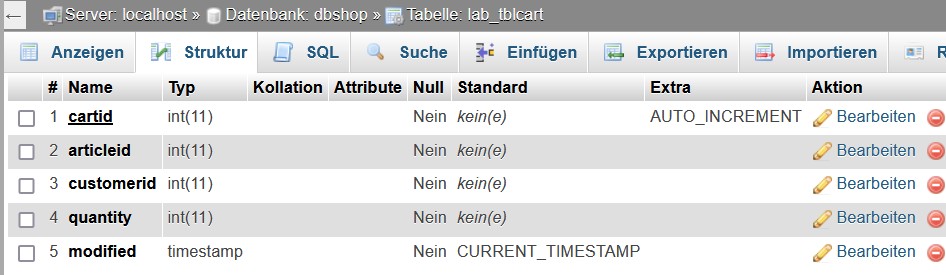
Datenbanktabelle lab_tblcart
Hier der SQL-Code zur Erzeugung der Datenbanktabelle (lab_tblcart).
CREATE TABLE IF NOT EXISTS `lab_tblcart` (
`cartid` int(11) NOT NULL,
`articleid` int(11) NOT NULL,
`customerid` int(11) NOT NULL,
`quantity` int(11) NOT NULL,
`modified` timestamp NOT NULL DEFAULT CURRENT_TIMESTAMP
) ENGINE=InnoDB AUTO_INCREMENT=1 DEFAULT CHARSET=latin1;
ALTER TABLE `lab_tblcart`
ADD PRIMARY KEY (`cartid`);
ALTER TABLE `lab_tblcart`
MODIFY `cartid` int(11) NOT NULL AUTO_INCREMENT,AUTO_INCREMENT=1;
config.php
Diese Datei (config.php) beinhaltet den Code für Herstellung zur Datenbank.<?php mysql_connect("localhost", "dbshop", "dbshop_password"); mysql_select_db("dbshop"); function db_connect() { $result = new mysqli("localhost", "dbshop", "dbshop_password", "dbshop"); if (!$result) return FALSE; $result->autocommit(TRUE); return $result; } ?>
header.php
Diese Datei (header.php) beinhaltet das HTML-Grundgerüst, das Einbinden von CSS-, JS- und PHP-Dateien.<?php session_start(); include("config.php"); ?> <!DOCTYPE html> <html lang="en"> <head> <meta charset="UTF-8"> <meta http-equiv="X-UA-Compatible" content="IE=edge"> <meta name="viewport" content="width=device-width, initial-scale=1.0"> <title>Labblog by Bandegani</title> <!-- CSS only --> <link href="https://cdn.jsdelivr.net/npm/bootstrap@5.2.2/dist/css/bootstrap.min.css" rel="stylesheet" integrity="sha384-Zenh87qX5JnK2Jl0vWa8Ck2rdkQ2Bzep5IDxbcnCeuOxjzrPF/et3URy9Bv1WTRi" crossorigin="anonymous"> <link class="favicon" rel="shortcut icon" href="https://imgs.search.brave.com/ElF6Rl1nG5Uo7fMOxeZQ1yQA_e0iuclCbXNmuJWcL34/rs:fit:475:225:1/g:ce/aHR0cHM6Ly90c2Uy/Lm1tLmJpbmcubmV0/L3RoP2lkPU9JUC5V/UVpOWFczTkM4OUhl/REZBb2hyNHp3SGFI/WiZwaWQ9QXBp" type="image/x-icon"> <link rel="stylesheet" href="https://cdnjs.cloudflare.com/ajax/libs/font-awesome/4.7.0/css/font-awesome.min.css"> <link rel="stylesheet" href="./static/user_profile.css"> <link rel="stylesheet" href="./static/auth.css"> <link rel="stylesheet" href="./static/cart.css"> </head> <body>
nav.php
Diese Datei (nav.php) beinhaltet den Code für die Navigation von Besucher.<!-- navbar --> <header class="header-navbar"> <div class="px-3 py-2 border-bottom mb-0" style="background-color: #E4E4E4;"> <div class="container"> <div class="d-flex flex-wrap align-items-center justify-content-center justify-content-lg-start"> <form action="results.php" method="get" target="_blank" class="d-flex me-lg-auto" role="search"> </form> <ul class="nav col-12 col-lg-auto my-2 justify-content-center my-md-0 text-small float-end"> <li><a href="results.php" class="nav-link text-dark"><i class="fa fa-search" aria-hidden="true"></i></a></li> <li><a href="cart.php" class="nav-link text-dark"><i class="fa fa-shopping-cart" aria-hidden="true"></i></a></li> <?php if(isset($_SESSION["customer_user"])){ ?> <li><a href="cprofile.php" class="nav-link text-dark" ><i class="fa fa-user" aria-hidden="true"></i></a></li> <li><a href="clogout.php" class="nav-link text-bg-dark">Logout</a></li> <?php } else { ?> <li><a href="clogin.php" class="nav-link text-dark" ><i class="fa fa-user" aria-hidden="true"></i></a></li> <li><a href="clogin.php" class="nav-link text-bg-dark">Log In</a></li> <?php } ?> </ul> </div> </div> </div> <div class="px-3 py-2 text-bg-dark"> <div class="container"> <header class="d-flex justify-content-center py-3"> <ul class="nav nav-pills"> <li class="px-5 py-0 nav-item "><a href="#" class="nav-link nav-elem text-light fs-5 text-uppercase fw-light disabled me-5">LAB Fashion</a></li> <li class="nav-item "><a href="index.php" class="nav-link nav-elem text-white mt-1 mx-5" aria-current="page">Home</a></li> <?php $sqlstr_cat="SELECT catid, catname FROM lab_tblcategories WHERE 1"; $res_cat = mysql_query($sqlstr_cat) or die ("no connection to the database ".mysql_error()); ?> <?php while ($row_cat = mysql_fetch_assoc($res_cat)) { ?> <li class="nav-item dropdown"> <a href="#" class="nav-link nav-elem dropdown-toggle text-white mt-1 mx-3" role="button" data-bs-toggle="dropdown" aria-expanded="false" > <?php echo $row_cat["catname"]; ?></a> <ul class="dropdown-menu text-bg-dark"> <?php $sqlstr_subcat="SELECT subcatid, catid, subcatname FROM lab_tblsubcategories WHERE catid=".$row_cat["catid"]; $res_subcat = mysql_query($sqlstr_subcat) or die ("no connection to the database ".mysql_error()); while ($row_subcat = mysql_fetch_assoc($res_subcat)) { echo "<li class='"; if($row_subcat["catid"]==3){ echo "'><a href='articles.php?subcatid=".$row_subcat["subcatid"]."' class='dropdown-item text-white link-dark'> ".$row_subcat["subcatname"]."</a></li>"; }else { echo "'><a href='articles.php?subcatid=".$row_subcat["subcatid"]."' class='dropdown-item text-white link-dark'> ".$row_subcat["subcatname"]."</a></li>"; } } ?> </ul> </li> <?php } mysql_free_result($res_cat); mysql_free_result($res_subcat); ?> </ul> </header> </div> </div> </header>
footer.php
Diese Datei (footer.php) beinhaltet den Fußbereich und das Einbinden von JS-Dateien.<!-- footer --> <div class="footer" style="background-color: #E4E4E4; margin-top: 5em;"> <footer class="py-3"> <ul class="nav justify-content-center pb-3 mb-3"> <li class="nav-item"><a href="home.php" class="nav-link px-2 text-muted">Home</a></li> <li class="nav-item"><a href="collection.php?collection=men" class="nav-link px-2 text-muted">Men's Fashion</a></li> <li class="nav-item"><a href="collection.php?collection=women" class="nav-link px-2 text-muted">Women's Fashion</a></li> <li class="nav-item"><a href="collection.php?collection=accessories" class="nav-link px-2 text-muted">Accessories</a></li> <li class="nav-item"><a href="lookbook.php" class="nav-link px-2 text-muted">Lookbook</a></li> <li class="nav-item"><a href="customer_care.php" class="nav-link px-2 text-muted">Customer Care</a></li> <?php if(isset($_SESSION["customer_user"])){ ?> <li class="nav-item"><a href="customer_profile.php" class="nav-link px-2 text-muted" >Profile</a></li> <?php } else { ?> <li class="nav-item"><a href="clogin.php" class="nav-link px-2 text-muted" >Profile</a></li> <?php } ?> </ul> <p class="text-center text-muted"><a class="text-success fw-bold text-decoration-none" href="http://bandegani.de/itkurs/labblog/home.php">© LAB FASHION</a></p> </footer> </div> <!-- JavaScript Bundle with Popper --> <script src="https://cdn.jsdelivr.net/npm/bootstrap@5.2.2/dist/js/bootstrap.bundle.min.js" integrity="sha384-OERcA2EqjJCMA+/3y+gxIOqMEjwtxJY7qPCqsdltbNJuaOe923+mo//f6V8Qbsw3" crossorigin="anonymous"></script> <script src="./static/form-validation.js"></script> </body> </html>
index.php
Diese Datei (index.php) beinhaltet Startseite.<?php session_start(); include("header.php"); include("nav.php"); if (isset($_GET["pageno"])) { $pageno = $_GET["pageno"]; } else { $pageno = 1; } $no_of_records_per_page = 10; $offset = ($pageno-1) * $no_of_records_per_page; ?> <div class="container cont_new col-md-12"> <h2 class="h2_cont_new text-center">Home</h2> <div class="cont-card row"> <?php $sqlstr = "SELECT COUNT(*) FROM lab_tblarticles"; $res = mysql_query($sqlstr) or die ("no connection to the database ".mysql_error()); $total_rows = mysql_fetch_array($res)[0]; $total_pages = ceil($total_rows / $no_of_records_per_page); $sqlstr_limit = "SELECT * FROM lab_tblarticles WHERE approved='yes' ORDER BY modified DESC LIMIT $offset, $no_of_records_per_page"; $res= mysql_query($sqlstr_limit) or die ("no connection to the database ".mysql_error()); $i=0; while ($result = mysql_fetch_assoc($res)) { $i=$i+1; ?> <div class="card border-0 col-md-3 mb-2" style="width: 18rem;"> <?php if ($result["type"] == "image") { ?> <img src="../admin/<?php echo $result["pic"]; ?>" style="width: 18rem; height: 18rem;" class="card-img-top" alt=""> <?php } else if ($result["type"] == "video") { ?> <video src="../admin/<?php echo $result["pic"]; ?>" type="video/mp4" controls/></video> <?php }?> <div class="card-body"> <p class="card-head text-center"><?php echo shortText($result["articlename"], 150); ?></p> <p class="card-head text-center">$ <?php echo $result["price"]; ?></p> <a href="articlesdetails.php?articleid=<?php echo $result["articleid"];?>" type="button" class="btn btn-dark w-100">Quick View</a> </div> </div><!-- Ende von col in Content --> <?php } mysql_free_result($res); ?> </div><!-- Ende von Row in Conent --> <hr> <div class="cont-card row"> <!-- Pagination with icons --> <nav aria-label="Page navigation example"> <ul class="pagination"> <li class="page-item"> <a class="page-link" href="?pageno=1" aria-label="Previous"> <span aria-hidden="true">«</span> </a> </li> <li class="page-item" class="<?php if($pageno <= 1){ echo "disabled"; } ?>"> <a class="page-link" href="<?php if($pageno <= 1){ echo "#"; } else { echo "?pageno=".($pageno - 1); } ?>"> <?php echo $pageno; ?></a></li> <li class="page-item" class="<?php if($pageno >= $total_pages){ echo "disabled"; } ?>"> <a class="page-link" href="<?php if($pageno >= $total_pages){ echo "#"; } else { echo "?pageno=".($pageno + 1); } ?>"> <?php echo ($pageno+1); ?></a></li> <li class="page-item"> <a class="page-link" href="?pageno=<?php echo $total_pages; ?>" aria-label="Next"> <span aria-hidden="true">»</span> </a> </li> </ul> </nav><!-- End Pagination with icons --> </div> </div><!-- End container --> <?php function shortText($string,$lenght) { if(strlen($string) > $lenght) { $string = substr($string,0,$lenght)."..."; $string_ende = strrchr($string, " "); $string = str_replace($string_ende," ...", $string); } return $string; } ?> <?php include("footer.php"); ?>
home.php
Diese Datei (home.php) beinhaltet Home-Seite.<?php include("header.php"); session_start(); ?> <header id="header" class="header d-flex align-items-center fixed-top"> <div class="container-fluid container-xl d-flex align-items-center justify-content-between"> <a href="index.php" class="logo d-flex align-items-center"> <!-- Uncomment the line below if you also wish to use an image logo --> <img src="assets/img/haw-logo.jpg" alt="Shopsystem"> <!--h1>ZenBlog</h1--> </a> <nav id="navbar" class="navbar"> <ul> <li><a href="home.php"><span class="fa fa-home"></span> HOME</a></li> <li><a href="description.php"><span class="fa fa-news"></span> DESCRIPTION</a></li> <li><a href="index.php"><span class="fa fa-cart"></span> CART</a></li> </ul> </nav><!-- .navbar --> </div> </header> <main id="main"> <!-- ======= Search Results ======= --> <section id="gallery" class="gallery"> <div class="container" data-aos="fade-up"> <div ="page-title"> <nav> <h3>Blogsystem - <?php echo date("l j F Y"); ?></h3> <h4><a href="home.php">Home</a></h4> <hr> </nav> </div><!-- End Page Title --> <div class="row"> <div class="col-md-1"></div> <div class="col-md-10"> <p class="lead">Lernform</p> Die Inhalte stehen als Online-Kurs (<a href="http://www.bandegani.de/itkurs">http://www.bandegani.de/itkurs/</a>) zum Selbststudium zur Verfügung. Die einzelnen Konzepte werden Schritt für Schritt aufeinander aufbauend in kleinen, überschaubaren Wissensbausteinen/Kapiteln vermittelt. Besonderer Wert wird auf jeweils vollständige Beispiele gelegt, die selbst weiterentwickelt werden können. Selbsttestaufgaben mit Musterlösungen erlauben es, die erworbenen Programmierfähigkeiten zu überprüfen. Am Anfang und am Ende jedes Wissensbausteins können die bereits vorhandenen Fähigkeiten oder die im Wissensbaustein erworbenen Fähigkeiten anhand von Tests und Aufgaben in dem privat zugelassenen Bereich überprüft werden. <hr> <p class="lead">Berufsfeldorientierung</p> Die Studierenden werden auf typische Problemstellungen in der beruflichen Praxis des Entwurfs und der Anwendungsentwicklung wie ein Content Management System für Online-Shops mit PHP und MySQL vorbereitet. <hr> <p class="lead">Webbasierte Datenbank</p> Diese Website (www.bandegani.de/itkurs) steht zum Selbststudium und zur eigenen Entwicklung zur Verfügung. Zur Selbstentwicklung muss man den Zugriff auf den eigenen Bereich, das FTP-Programm und die MYSQL-Datenbank haben. Diese Zugriffe werden nach dem Einschreiben in den Kurs ermöglicht und erlischen nach vier Jahren. Danach werden auch alle dort gespeicherten Daten aus dem System entfernt. <hr> <p class="lead">Mögliche Themen für die Hausarbeit</p> <ul> <li>Online-Shop im Bereich Bekleidungstechnik,</li> <li>Protokollierung der Arbeitsvorgänge in einem Unternehmen,</li> <li>Einkauf von Rohmaterialien bei verschiedenen Lieferanten,</li> <li>Lagerbestandssystem für die Materialien,</li> <li>Erstellung von Vor- und Nachkalkulationen für die Materialien, Maschinen und Personalien,</li> <li>Auswertung für die Zufriedenheit der Mitarbeiter, Kunden, usw.,</li> <li>Datenbanksysteme für Stoffe, Veredlungen, Zubehör, Kleidung, Lieferanten, Kunden, Mitarbeiter, Maßtabellen, Passformklassen, Gehälter usw.</li> </ul> <hr> <p class="lead">Hilfestellung</p> Sie können für die Realisation Ihrer Hausarbeit auch ein beliebiges Open Source System benutzen, das auf PHP5, MySQL und JavaScript basiert ist. Vor der Installation muss aber das Open Source System von der Kursleiterin oder dem Kursleiter genehmigt werden. <hr> <p class="lead">Organisation, Voraussetzung und Gewichtung</p> In der Lehrveranstaltung werden Konzept, Systemstruktur, Hilfsmittel, Vorlagen und wesentliche Informationen zu der Entwicklung von einem Online-System besprochen. Die Studierenden müssen dem Kursleiter das Thema der Hausarbeit spätestens 5 Wochen vor dem Prüfungstermin bekannt geben. Die Studierenden müssen ihre Hausarbeit am Prüfungstermin präsentiert. An dem Termin sollen alle eingeschriebene Studierenden teilnehmen und die Präsentationen anhören. Die Präsentation und die Anwesenheit der Studierenden fließen mit in die Note ein. Für das Bestehen des Kurses erhalten Sie 5 CP's. </div><!-- End col-lg-10 --> <div class="col-md-1"></div> </div><!-- End row --> </div><!-- End container --> </section> <!-- End Search Result --> </main><!-- End #main --> <?php include("footer.php"); ?>
articles.php
Diese Datei (articles.php) zeigt alle Artikeln in Abhängigkeit von einer Unterkategorie.<?php session_start(); include("header.php"); include("nav.php"); $subcatid = $_GET["subcatid"]; if ($subcatid<=0 ) { $subcatid=1; } $_SESSION["subcatid"] = $subcatid; if (isset($_GET["pageno"])) { $pageno = $_GET["pageno"]; } else { $pageno = 1; } $no_of_records_per_page = 10; $offset = ($pageno-1) * $no_of_records_per_page; ?> <div class="container cont_new col-md-12"> <h2 class="h2_cont_new text-center">Home</h2> <div class="cont-card row"> <?php $sqlstr="SELECT * FROM lab_tblsubcategories WHERE subcatid = ". $subcatid; //$sqlstr = "SELECT COUNT(*) FROM lab_tblarticles"; $res = mysql_query($sqlstr) or die ("no connection to the database ".mysql_error()); $total_rows = mysql_fetch_array($res)[0]; $total_pages = ceil($total_rows / $no_of_records_per_page); $sqlstr_limit = "SELECT * FROM lab_tblarticles WHERE approved='yes' AND subcatid ='$subcatid' ORDER BY modified DESC LIMIT $offset, $no_of_records_per_page"; $res= mysql_query($sqlstr_limit) or die ("no connection to the database ".mysql_error()); $i=0; while ($result = mysql_fetch_assoc($res)) { $i=$i+1; ?> <div class="card border-0 col-md-3 mb-2" style="width: 18rem;"> <?php if ($result["type"] == "image") { ?> <img src="../admin/<?php echo $result["pic"]; ?>" style="width: 18rem; height: 18rem;" class="card-img-top" alt=""> <?php } else if ($result["type"] == "video") { ?> <video src="../admin/<?php echo $result["pic"]; ?>" type="video/mp4" controls/></video> <?php }?> <div class="card-body"> <p class="card-head text-center"><?php echo shortText($result["articlename"], 150); ?></p> <p class="card-head text-center">$ <?php echo $result["price"]; ?></p> <a href="articlesdetails.php?articleid=<?php echo $result["articleid"];?>" type="button" class="btn btn-dark w-100">Quick View</a> </div> </div><!-- Ende von col in Content --> <?php } mysql_free_result($res); ?> </div><!-- Ende von Row in Conent --> <hr> <div class="cont-card row"> <!-- Pagination with icons --> <nav aria-label="Page navigation example"> <ul class="pagination"> <li class="page-item"> <a class="page-link" href="?pageno=1" aria-label="Previous"> <span aria-hidden="true">«</span> </a> </li> <li class="page-item" class="<?php if($pageno <= 1){ echo "disabled"; } ?>"> <a class="page-link" href="<?php if($pageno <= 1){ echo "#"; } else { echo "?pageno=".($pageno - 1); } ?>"> <?php echo $pageno; ?></a></li> <li class="page-item" class="<?php if($pageno >= $total_pages){ echo "disabled"; } ?>"> <a class="page-link" href="<?php if($pageno >= $total_pages){ echo "#"; } else { echo "?pageno=".($pageno + 1); } ?>"> <?php echo ($pageno+1); ?></a></li> <li class="page-item"> <a class="page-link" href="?pageno=<?php echo $total_pages; ?>" aria-label="Next"> <span aria-hidden="true">»</span> </a> </li> </ul> </nav><!-- End Pagination with icons --> </div> </div><!-- End container --> <?php function shortText($string,$lenght) { if(strlen($string) > $lenght) { $string = substr($string,0,$lenght)."..."; $string_ende = strrchr($string, " "); $string = str_replace($string_ende," ...", $string); } return $string; } ?> <?php include("footer.php"); ?>
articlesdetails.php
Diese Datei (articlesdetails.php) stellt die Dtails von einem Artikel.<?php session_start(); include("header.php"); include("nav.php"); if(isset($_POST["articleid"])){ $articleid = $_POST["articleid"]; }elseif(!isset($_GET["articleid"]) ||$_GET["articleid"]==NULL ){ }else{ $articleid=$_GET["articleid"]; } $subcatid = $_GET["subcatid"]; if ($subcatid<=0 ) { $subcatid=1; } $_SESSION["subcatid"] = $subcatid; ?> <?php $sqlstr="SELECT * FROM lab_tblarticles WHERE approved='yes' AND articleid=$articleid"; $res = mysql_query($sqlstr) or die ("no connection to the database ".mysql_error()); while($result = mysql_fetch_assoc($res)) { ?> <head> <link rel="stylesheet" href="./static/product.css"> </head> <!-- main --> <div class="container my-5"> <div class="row"> <div class="col-md-5"> <div class="main-img"> <?php if ($result["type"] == "image") { if($result["pic"]=="../assets/img/logo_shop.png"){?> <?php } else { ?> <img src="../admin/<?php echo $result["pic"]; ?>" class="img-fluid"> <?php } } else if ($result["type"] == "video") { ?> <video src="../admin/<?php echo $result["pic"]; ?>" type="video/mp4" controls/></video> <?php } ?> <div class="row my-3 previews"> <div class="col-md-3"> <img class="w-100" src="../admin/<?php echo $result["pic"]; ?>" alt="Sale"> </div> <div class="col-md-3"> <img class="w-100" src="../admin/<?php echo $result["pic"]; ?>" alt="Sale"> </div> </div> </div> </div> <div class="col-md-7"> <div class="main-description px-2"> <div class="category text-bold"> Collection: <?php echo $result["product"]; ?> </div> <div class="product-title fw-semibold my-3 text-uppercase fs-3"> <?php echo $result["articlename"]; ?> </div> <div class="price-area my-4"> <p class="new-price text-bold mb-1">$ <?php echo $result["price"]; ?> <span class="old-price-discount text-danger fs-5 fw-normal">(20% off)</span> </p> <p class="text-secondary mb-1">(Additional tax may apply on checkout)</p> </div> <div class="product-details my-4"> <h4 style="color:green;">In Stock</h4> <p class="new-price text-bold mb-1 fs-4">Quantity</p> <!-- ----------------------------- --> <form action="add_to_cart.php" method="POST"> <input type="number" class="form-control w-25" name="quantity" min="1" max="" value="1" placeholder="Enter the Quantity"> <input type="hidden" name="articleid" value="<?php echo $result["articleid"]; ?>"> <?php if(isset($_SESSION["customerid"])) { ?> <!-- customerid wurde in clogin.php festgelegt. --> <input type="hidden" name="customerid" value="<?php echo $_SESSION["customerid"]; ?>"> <div class="buttons d-flex my-5"> <div class="block text-white" style="background-color: #add0db"> <button type="submit" name="submit" class="shadow btn custom-btn">Add to cart</button> </div> </div> <?php } else {?> <div class="buttons d-flex my-5"> <div class="block text-white" style="background-color: #add0db"> <a class="shadow btn custom-btn disabled">Add to cart</a> </div> </div> <p class="description text-danger">Log in to add this item to cart!</p> <?php } ?> </form> </div> </div> <div class="product-details my-4"> <p class="details-title text-color mb-1">Product Details</p> <p class="description"><?php echo $result["description"]; ?></p> </div> <div class="product-details my-4"> <p class="details-title text-color mb-2">Material & Care</p> <ul> <li>Cotton</li> <li>Machine-wash</li> </ul> </div> <div class="product-details my-4"> <p class="details-title text-color mb-2">Sold by</p> <ul> <li>Cotton</li> <li>Machine-wash</li> </ul> </div> </div> </div> </div> <?php } mysql_free_result($res); ?> <div class="container similar-products my-4"> <hr> <p class="display-5">Shop More</p> <div class="row"> <?php $sqlstr="SELECT * FROM lab_tblarticles where approved='yes' ORDER BY modified DESC LIMIT 4"; $res = mysql_query($sqlstr) or die ("no connection to the database ".mysql_error()); while ($result = mysql_fetch_assoc($res)) { ?> <div class="col-md-3"> <div class="similar-product text-center"> <?php if ($result["type"] == "image") { ?> <img src="../admin/<?php echo $result["pic"]; ?>" style="width: 18rem; height: 18rem;" class="card-img-top" alt=""> <?php } else if ($result["type"] == "video") { ?> <video src="../admin/<?php echo $result["pic"]; ?>" type="video/mp4" controls/></video> <?php }?> <div class="card-body"> <p class="card-head text-center"><?php echo shortText($result["articlename"], 150); ?></p> <p class="card-head text-center">$ <?php echo $result["price"]; ?></p> <a href="articlesdetails.php?articleid=<?php echo $result["articleid"];?>" type="button" class="btn btn-dark w-100">Quick View</a> </div> </div> </div> <?php } ?> </div> </div> <?php include("footer.php"); ?> <?php function shortText($string,$lenght) { if(strlen($string) > $lenght) { $string = substr($string,0,$lenght)."..."; $string_ende = strrchr($string, " "); $string = str_replace($string_ende," ...", $string); } return $string; } ?>
results.php
Diese Datei (results.php) sucht nach dem Artikelnamen.<?php session_start(); include("header.php"); include("nav.php"); ?> <div class="container cont_new col-md-12"> <h2>search results</h2> <form method="POST" action="results.php"> <div class="mb-3 search-box"> <input class="form-control me-2 shadow-none border-0" type="search" name="search" placeholder="Search..." aria-label="Search" autocomplete="off"> <button class="border-0 btn btn-r" name="submit" type="submit" href="#" aria-label="Search"> </button> </div> </form> <div class="row"> <?php if(isset($_POST["submit"])) { $search=$_POST["search"]; $sqlstr = "select * from lab_tblarticles where articlename like '%$search%'"; $res = mysql_query($sqlstr) or die ("no connection to the database ".mysql_error()); if($res) { while($result = mysql_fetch_assoc($res)) { ?> <div class="col-md-3"> <div class="similar-product text-center"> <?php if ($result["type"] == "image") { ?> <img src="../admin/<?php echo $result["pic"]; ?>" style="width: 18rem; height: 18rem;" class="card-img-top" alt=""> <?php } else if ($result["type"] == "video") { ?> <video src="../admin/<?php echo $result["pic"]; ?>" type="video/mp4" controls/></video> <?php }?> <div class="card-body"> <p class="card-head text-center"><?php echo shortText($result["articlename"], 150); ?></p> <p class="card-head text-center">$ <?php echo $result["price"]; ?></p> <a href="articlesdetails.php?articleid=<?php echo $result["articleid"];?>" type="button" class="btn btn-dark w-100">Quick View</a> </div> </div> </div> <?php } } else { ?> <div class="container search-result w-100"> <h5 class="h5-search text-start fw-light">No results found. Try a new search.</h5> </div> <?php } } ?> </div> </div> <?php include("footer.php"); ?> <?php function shortText($string,$lenght) { if(strlen($string) > $lenght) { $string = substr($string,0,$lenght)."..."; $string_ende = strrchr($string, " "); $string = str_replace($string_ende," ...", $string); } return $string; } ?>
clogin.php
Diese Datei (clogin.php) wird aufgerufen, wenn der Kunde sich anmeldet, um die ausgewählten Artikeln zu bestellen.<?php session_start(); include("header.php"); include("nav.php"); if(isset($_POST["send"])) { if ($_POST["email"] && $_POST["password"]) { $email = $_POST["email"]; $password=MD5($_POST["password"]); $sqlstr = "select * from lab_tblcustomers where email='$email' and password = '$password'"; $res = mysql_query($sqlstr) or die ("no connection to the database ".mysql_error()); $num = mysql_fetch_array($res); if ($num["email"]==$email && $num["password"]==$password){ $_SESSION["customer_user"] = $email; $_SESSION["customerid"] = $num["customerid"]; ?> <script> location.replace("cart.php"); </script> <?php } else { echo "<script>alert('password Incorrect');</script>"; } } else { echo "<script>alert('Invalid Email');</script>"; } mysql_free_result($res); } ?> <div class="row"> <div class="col-xs-12 col-sm-12 col-md-3 col-lg-3"></div> <div class="col-xs-12 col-sm-12 col-md-6 col-lg-6"> <div class="signup-form"> <form action="<?php echo htmlentities($_SERVER["PHP_SELF"]); ?>" method="POST"> <h2>Log In</h2> <div class="form-group"> <input type="email" class="form-control input-lg" name="email" placeholder="Email Address" required="required"> </div> <div class="form-group"> <input type="password" class="form-control input-lg" name="password" placeholder="Password" required="required"> </div> <div class="form-group"> <button type="submit" name="send" class="btn btn-success btn-lg btn-block signup-btn">Log In</button> </div> </form> <div class="text-center">New to site? <a href="cregister.php">Sign Up</a></div> </div> </div> <div class="col-xs-12 col-sm-12 col-md-3 col-lg-3"></div> </div> <?php include("footer.php"); ?>
clogout.php
Diese Datei (clogout.php) wird aufgerufen, wenn der Kunde sich abmelden will.
<?php
session_start();
session_destroy();
header("location:index.php");
?>
cregister.php
Diese Datei (cregister.php) wird aufgerufen, wenn der Kunde sich neu registrieren will.<?php session_start(); include("header.php"); include("nav.php"); $uploadDirectory = __DIR__."/"; ?> <main id="main"> <!-- ======= Search Results ======= --> <section id="gallery" class="gallery"> <div class="container" data-aos="fade-up"> <div ="page-title"> <nav> <h3>Blogsystem - <?php echo date("l j F Y"); ?></h3> <h4><a href="home.php">Home</a></h4> <hr> </nav> </div><!-- End Page Title --> <div class="row"> <div class="col-lg-3 col-md-3 col-sm-12 col-xs-12"></div><!-- end col --> <div class="col-lg-6 col-md-6 col-sm-12 col-xs-12"> <div class="panel panel-primary"> <div class="card-body"> <h2>Add new customer</h2> <?php if(isset($_FILES["uploadFile"])){ $errors= array(); $file_name = $_FILES["uploadFile"]["name"]; $file_size = $_FILES["uploadFile"]["size"]; $file_tmp = $_FILES["uploadFile"]["tmp_name"]; $file_type = $_FILES["uploadFile"]["type"]; $file_ext=strtolower(end(explode(".",$_FILES["uploadFile"]["name"]))); $unique_pic = substr(md5(time()), 0, 10).".".$file_ext; $uploaded_pic = "admin/uploads/customers/".$unique_pic; if(!empty($file_name)){ if(empty($errors)==true) { $type = pathinfo($file_name, PATHINFO_EXTENSION); $fileExists = true; while ($fileExists) { if (!file_exists($uploadDirectory.$uploaded_pic)) { $fileExists = false; } } move_uploaded_file($file_tmp,$uploadDirectory.$uploaded_pic); $mediaType = "something"; switch ($type) { case "mp4": case "mkv": case "mov": case "ogg": case "webm": $mediaType = "video"; break; case "jpg": case "jpeg": case "gif": case "png": default: $mediaType = "image"; break; } } }else { $uploaded_pic = "admin/uploads/customers/shop_logo.gif"; $mediaType = "pic"; } $userid=1; $approved="yes"; $prename= $_POST["prename"]; $customername = $_POST["customername"]; $gender = $_POST["gender"]; $address = $_POST["address"]; $city = $_POST["city"]; $region = $_POST["region"]; $country = $_POST["country"]; $postcode = $_POST["postcode"]; $phone = $_POST["phone"]; $email = $_POST["email"]; $password = MD5($_POST["password"]); $sqlstr = "INSERT INTO lab_tblcustomers (prename, customername, gender, address, city, region, country, postcode, phone, email, password,pic, type, modified, userid, approved) VALUES('$prename', '$customername', '$gender', '$address', '$city', '$region', '$country', '$postcode', '$phone', '$email', '$password', '$uploaded_pic', '$mediaType', NOW() , '$userid', '$approved')"; $res = mysql_query($sqlstr) or die ("no connection to the database ".mysql_error()); $num = mysql_affected_rows(); if ($num>0) { echo '<div class="alert alert-success bg-success text-light border-0 alert-dismissible fade show" role="alert"> The Customerhas been added! </div>'; }else { echo '<div class="alert alert-danger bg-danger text-light border-0 alert-dismissible fade show" role="alert"> An error occurred while adding the Customer to the system! </div>'; } }else{ print_r($errors); } ?> <form class="forms-sample" action="cregister.php" method="POST" enctype="multipart/form-data"> <div class="form-group row mb-3"> <label class="col-sm-2 col-form-label">Gender</label> <select class="form-select" name="gender" aria-label="Floating label select example"> <option selected>Open this select menu</option> <option value="girl">Girl</option> <option value="boy">Boy</option> <option value="woman">Woman</option> <option value="gentleman">Gentleman</option> </select> </div> <div class="form-group row mb-3"> <label for="exampleInputprename">Prename</label> <input type="text" name="prename" class="form-control" id="exampleInputprename" placeholder="Elke"> </div> <div class="form-group row mb-3"> <label for="exampleInputname">Name</label> <input type="text" name="customername" class="form-control" id="exampleInputname" placeholder="Raza"> </div> <div class="form-group row mb-3"> <label for="exampleInputaddress">Address</label> <input type="text" name="address" class="form-control" id="exampleInputaddress" placeholder="Armgartstraße 24"> </div> <div class="form-group"> <label for="exampleInputpostcode">Postcode</label> <input type="text" name="postcode" class="form-control" id="exampleInputpostcode" placeholder="22179"> </div> <div class="form-group"> <label for="exampleInputcity">City</label> <input type="text" name="city" class="form-control" id="exampleInputcity" placeholder="Hamburg"> </div> <div class="form-group"> <label for="exampleInputregion">Region</label> <input type="text" name="region" class="form-control" id="exampleInputregion" placeholder="Hamburg"> </div> <div class="form-group"> <label for="exampleInputcountry">Country</label> <input type="text" name="country" class="form-control" id="exampleInputcountry" placeholder="Germany"> </div> <div class="form-group"> <label for="exampleInputphone">Phone</label> <input type="text" name="phone" class="form-control" id="exampleInputphone" placeholder="040/4285238"> </div> <div class="form-group"> <label for="exampleInputemail">Email</label> <input type="email" name="email" class="form-control" id="exampleInputemail" placeholder="elke.raza@haw-hamburg.de"> </div> <div class="form-group"> <label for="exampleInputpassword">Password</label> <input type="password" name="password" class="form-control" id="exampleInputpassword"> </div> <div> <label class="form-label">Image/Clip</label> <div> <input type="file" name="uploadFile" class="form-control"> </div> </div> <button class="btn btn-success" type="submit" name="submit">Add customer</button> </form> </div> </div><!-- Ende von Panel --> </div><!-- end col --> <div class="col-lg-3 col-md-3 col-sm-12 col-xs-12"></div><!-- end col --> </div><!-- end row --> </div><!-- end container --> </section><!-- end section --> </main> <?php include("footer.php"); ?>
cart.php
Diese Datei (cart.php) stellt den Warenkorb dar.<?php session_start(); include("header.php"); include("nav.php"); $customerid=$_SESSION["customerid"]; $summe=0; // Total of all the items in the cart if(isset($_SESSION["customerid"])) { $sqlstr_quantity = "SELECT * FROM lab_tblcart WHERE customerid ='$customerid'"; $res_quantity = mysql_query($sqlstr_quantity) or die ("no connection to the database ".mysql_error()); while($quantity_row = mysql_fetch_assoc($res_quantity)) { $articleid=$quantity_row["articleid"]; $quantity=$quantity_row["quantity"]; $sqlstr_price = "SELECT * FROM lab_tblarticles WHERE articleid='$articleid'"; $res_price = mysql_query($sqlstr_price) or die ("no connection to the database ".mysql_error()); if(mysql_num_rows($res_price) > 0) { while($price_row = mysql_fetch_assoc($res_price)) { $price=$price_row["price"]; $summe=$summe + $quantity*$price; } } } } // Total amount INCLUDING delivery charges $total_price = $summe; mysql_free_result($res_cart); mysql_free_result($res_quantity); mysql_free_result($res_price); ?> <div class="container cont_new col-md-12"> <div class="card" style="min-height: 50vh;"> <div class="row"> <div class="col-md-8 cart"> <div class="title"> <div class="row"> <div class="col"><h4><b>My Shopping Cart</b></h4></div> </div> </div> <?php if(isset($_SESSION["customerid"])) { //Authorized user $sql = "SELECT * FROM lab_tblcart WHERE customerid ='$customerid'"; $all_product = mysql_query($sql) or die ("no connection to the database ".mysql_error()); if(mysql_num_rows($all_product) > 0) { // If user has items in cart while($row = mysql_fetch_assoc($all_product)) { $articleid=$row["articleid"]; $quantity=$row["quantity"]; $cartid=$row["cartid"]; $new_sql = "SELECT * FROM lab_tblarticles WHERE articleid ='$articleid'"; $new_product = mysql_query($new_sql) or die ("no connection to the database ".mysql_error()); while($new_row = mysql_fetch_assoc($new_product)) { ?> <div class="row border-top border-bottom"> <div class="row main align-items-center"> <div class="col-2"><img class="img-fluid" src="../admin/<?php echo $new_row["pic"]; ?>"></div> <div class="col"> <div class="row text-muted"><?php echo $new_row["product"]; ?></div> <div class="row"><?php echo $new_row["articlename"]; ?></div> </div> <div class="col"><?php echo $quantity; ?> </div> <div class="col">$ <?php echo $quantity*$new_row["price"]; ?> <a href="cart_delete.php?cartid=<?php echo $cartid; ?>&customerid=<?php echo $custometid; ?>" class="close text-decoration-none">✕</a> </div> </div> </div> <?php } } ?> <div class="back-to-shop"> <a href="index.php" class="text-decoration-none">←<span class="text-muted">Back to shop</span></a> </div> </div> <div class="col-md-4 summary"> <div><h5><b>Summary</b></h5></div> <hr> <div class="row"> <div class="col" style="padding-left:0;">ITEMS <?php echo $num_row; ?></div> <div class="col text-right">$ <?php echo $total_price; ?></div> </div> <a href="checkout.php" class="btn btn-primary">CHECKOUT</a> </div> </div> </div> <?php } else { ?> <!-- If user has no item in cart --> <div class="row text-danger">Empty cart !</div> <div class="back-to-shop"> <a href="index..php" class="text-decoration-none">←<span class="text-muted">Back to shop</span></a> </div> </div> <div class="col-md-4 summary"> <div><img style="width: 25vw; height:40vh;" src="https://cdni.iconscout.com/illustration/premium/thumb/empty-cart-5521508-4610092.png"></div> </div> </div> </div> <?php } } else { ?> <!-- Unauthorized User --> <div class="row text-danger">Empty cart !</div> <div class="back-to-shop"> <a href="log-in.php" class="text-decoration-none fs-4 fw-bold"><i class="fa fa-sign-in" aria-hidden="true"></i> <span class="text-muted">Sign In to Shop Now</span></a> </div> </div> <div class="col-md-4 summary"> <div><img style="width: 25vw; height:40vh;" src="./static/cart.png"></div> </div> </div> </div> <?php } mysql_free_result($all_product); mysql_free_result($$new_product); ?> </div> <?php include("footer.php"); ?>
add_to_cart.php
Diese Datei (add_to_cart.php) fügt einen neuen Artikel in Warenkorb hinzu, wenn der Kunde angemeldet ist.<?php session_start(); include("header.php"); $articleid = $_POST["articleid"]; $customerid = $_POST["customerid"]; $quantity = $_POST["quantity"]; $sqlstr="INSERT INTO lab_tblcart (articleid, customerid, quantity, modified) VALUES ('$articleid', '$customerid', '$quantity', NOW())"; $res = mysql_query($sqlstr) or die ("no connection to the database ".mysql_error()); header("Location: articlesdetails.php?articleid={$articleid}"); ?>
cart_delete.php
Diese Datei (cart_delete.php) entfernt einen Eintrag aus dem Warenkorb.<!-- To delete item from cart --> <?php session_start(); include("header.php"); $cartid = $_GET["cartid"]; $customerid = $_SESSION["customerid"]; $sqlstr = "DELETE FROM lab_tblcart WHERE cartid='$cartid' AND customerid='$customerid'"; $res = mysql_query($sqlstr) or die ("no connection to the database ".mysql_error()); header("Location: cart.php?cartid='$cartid'"); mysql_free_result($res); ?>
checkout.php
Diese Datei (checkout.php) stellt das Formular zur Zahlungsart her.<?php session_start(); include("header.php"); include("nav.php"); $customerid=$_SESSION["customerid"]; ?> <div class="container cont_new col-md-12"> <div class="py-5 text-center"> <img class="d-block mx-auto mb-4" src="./static/cart.png" alt="" width="120" height="auto"> <h2>Checkout form</h2> <p class="lead">Each required form group has a validation state that can be triggered by attempting to submit the form without completing it.</p> </div> <div class="row"> <div class="col-md-4 order-md-2 mb-4"> <h4 class="d-flex justify-content-between align-items-center mb-3"> <span class="text-muted">Your cart</span> </h4> <ul class="list-group mb-3"> <?php if(isset($_SESSION["customerid"])) { $sql = "SELECT * FROM lab_tblcart WHERE customerid ='$customerid'"; $all_product = mysql_query($sql) or die ("no connection to the database ".mysql_error()); if(mysql_num_rows($all_product) > 0) { // If user has items in cart while($row = mysql_fetch_assoc($all_product)) { $articleid=$row["articleid"]; $quantity=$row["quantity"]; $cartid=$row["cartid"]; $new_sql = "SELECT * FROM lab_tblarticles WHERE articleid ='$articleid'"; $new_product = mysql_query($new_sql) or die ("no connection to the database ".mysql_error()); while($new_row = mysql_fetch_assoc($new_product)) { ?> <li class="list-group-item d-flex justify-content-between lh-condensed"> <div> <h6 class="my-0"><a href="articlesdetails.php?articleid=<?php echo $new_row["articleid"];?>" ><?php echo $new_row["number"]; ?></a></h6> <small class="text-muted">quantity: <?php echo $quantity ?></small> </div> <span class="text-muted">$ <?php echo $quantity*$new_row["price"]; ?></span> </li> <?php } } } mysql_free_result($all_product); mysql_free_result($$new_product); } ?> </ul> <form class="card p-2"> <div class="input-group"> <input type="text" class="form-control" placeholder="Promo code"> <div class="input-group-append"> <button type="submit" class="btn btn-secondary">Redeem</button> </div> </div> </form> </div> <div class="col-md-8 order-md-1"> <h4 class="mb-3">Billing address</h4> <form class="needs-validation" novalidate> <div class="row"> <div class="col-md-6 mb-3"> <label for="firstName">First name</label> <input type="text" class="form-control" id="firstName" placeholder="" value="" required> <div class="invalid-feedback"> Valid first name is required. </div> </div> <div class="col-md-6 mb-3"> <label for="lastName">Last name</label> <input type="text" class="form-control" id="lastName" placeholder="" value="" required> <div class="invalid-feedback"> Valid last name is required. </div> </div> </div> <div class="mb-3"> <label for="username">Username</label> <div class="input-group"> <div class="input-group-prepend"> <span class="input-group-text">@</span> </div> <input type="text" class="form-control" id="username" placeholder="Username" required> <div class="invalid-feedback" style="width: 100%;"> Your username is required. </div> </div> </div> <div class="mb-3"> <label for="email">Email <span class="text-muted">(Optional)</span></label> <input type="email" class="form-control" id="email" placeholder="you@example.com"> <div class="invalid-feedback"> Please enter a valid email address for shipping updates. </div> </div> <div class="mb-3"> <label for="address">Address</label> <input type="text" class="form-control" id="address" placeholder="1234 Main St" required> <div class="invalid-feedback"> Please enter your shipping address. </div> </div> <div class="mb-3"> <label for="address2">Address 2 <span class="text-muted">(Optional)</span></label> <input type="text" class="form-control" id="address2" placeholder="Apartment or suite"> </div> <div class="row"> <div class="col-md-5 mb-3"> <label for="country">Country</label> <select class="custom-select d-block w-100" id="country" required> <option value="">Choose...</option> <option>United States</option> </select> <div class="invalid-feedback"> Please select a valid country. </div> </div> <div class="col-md-4 mb-3"> <label for="state">State</label> <select class="custom-select d-block w-100" id="state" required> <option value="">Choose...</option> <option>California</option> </select> <div class="invalid-feedback"> Please provide a valid state. </div> </div> <div class="col-md-3 mb-3"> <label for="zip">Zip</label> <input type="text" class="form-control" id="zip" placeholder="" required> <div class="invalid-feedback"> Zip code required. </div> </div> </div> <hr class="mb-4"> <div class="custom-control custom-checkbox"> <input type="checkbox" class="custom-control-input" id="same-address"> <label class="custom-control-label" for="same-address">Shipping address is the same as my billing address</label> </div> <div class="custom-control custom-checkbox"> <input type="checkbox" class="custom-control-input" id="save-info"> <label class="custom-control-label" for="save-info">Save this information for next time</label> </div> <hr class="mb-4"> <h4 class="mb-3">Payment</h4> <div class="d-block my-3"> <div class="custom-control custom-radio"> <input id="credit" name="paymentMethod" type="radio" class="custom-control-input" checked required> <label class="custom-control-label" for="credit">Credit card</label> </div> <div class="custom-control custom-radio"> <input id="debit" name="paymentMethod" type="radio" class="custom-control-input" required> <label class="custom-control-label" for="debit">Debit card</label> </div> <div class="custom-control custom-radio"> <input id="paypal" name="paymentMethod" type="radio" class="custom-control-input" required> <label class="custom-control-label" for="paypal">PayPal</label> </div> </div> <div class="row"> <div class="col-md-6 mb-3"> <label for="cc-name">Name on card</label> <input type="text" class="form-control" id="cc-name" placeholder="" required> <small class="text-muted">Full name as displayed on card</small> <div class="invalid-feedback"> Name on card is required </div> </div> <div class="col-md-6 mb-3"> <label for="cc-number">Credit card number</label> <input type="text" class="form-control" id="cc-number" placeholder="" required> <div class="invalid-feedback"> Credit card number is required </div> </div> </div> <div class="row"> <div class="col-md-3 mb-3"> <label for="cc-expiration">Expiration</label> <input type="text" class="form-control" id="cc-expiration" placeholder="" required> <div class="invalid-feedback"> Expiration date required </div> </div> <div class="col-md-3 mb-3"> <label for="cc-cvv">CVV</label> <input type="text" class="form-control" id="cc-cvv" placeholder="" required> <div class="invalid-feedback"> Security code required </div> </div> </div> <hr class="mb-4"> <button type="submit" class="btn btn-secondary">Continue to checkout</button> </form> </div> </div> </div> <?php include("footer.php"); ?>